Wekan REST API v7.61
Scroll down for code samples, example requests and responses. Select a language for code samples from the tabs above or the mobile navigation menu.
The REST API allows you to control and extend Wekan with ease.
If you are an end-user and not a dev or a tester, create an issue to request new APIs.
All API calls in the documentation are made using
curl
. However, you are free to use Java / Python / PHP / Golang / Ruby / Swift / Objective-C / Rust / Scala / C# or any other programming languages.
Production Security Concerns
When calling a production Wekan server, ensure it is running via HTTPS and has a valid SSL Certificate. The login method requires you to post your username and password in plaintext, which is why we highly suggest only calling the REST login api over HTTPS. Also, few things to note:
- Only call via HTTPS
- Implement a timed authorization token expiration strategy
- Ensure the calling user only has permissions for what they are calling and no more
Authentication
- API Key (UserSecurity)
- Parameter Name: Authorization, in: header.
Login
login
Code samples
# You can also use wget
curl -X POST /users/login \
-H 'Content-Type: application/x-www-form-urlencoded' \
-H 'Accept: */*'
POST /users/login HTTP/1.1
Content-Type: application/x-www-form-urlencoded
Accept: */*
const inputBody = '{
"username": "string",
"password": "pa$$word"
}';
const headers = {
'Content-Type':'application/x-www-form-urlencoded',
'Accept':'*/*'
};
fetch('/users/login',
{
method: 'POST',
body: inputBody,
headers: headers
})
.then(function(res) {
return res.json();
}).then(function(body) {
console.log(body);
});
const fetch = require('node-fetch');
const inputBody = {
"username": "string",
"password": "pa$$word"
};
const headers = {
'Content-Type':'application/x-www-form-urlencoded',
'Accept':'*/*'
};
fetch('/users/login',
{
method: 'POST',
body: JSON.stringify(inputBody),
headers: headers
})
.then(function(res) {
return res.json();
}).then(function(body) {
console.log(body);
});
require 'rest-client'
require 'json'
headers = {
'Content-Type' => 'application/x-www-form-urlencoded',
'Accept' => '*/*'
}
result = RestClient.post '/users/login',
params: {
}, headers: headers
p JSON.parse(result)
import requests
headers = {
'Content-Type': 'application/x-www-form-urlencoded',
'Accept': '*/*'
}
r = requests.post('/users/login', headers = headers)
print(r.json())
URL obj = new URL("/users/login");
HttpURLConnection con = (HttpURLConnection) obj.openConnection();
con.setRequestMethod("POST");
int responseCode = con.getResponseCode();
BufferedReader in = new BufferedReader(
new InputStreamReader(con.getInputStream()));
String inputLine;
StringBuffer response = new StringBuffer();
while ((inputLine = in.readLine()) != null) {
response.append(inputLine);
}
in.close();
System.out.println(response.toString());
package main
import (
"bytes"
"net/http"
)
func main() {
headers := map[string][]string{
"Content-Type": []string{"application/x-www-form-urlencoded"},
"Accept": []string{"*/*"},
}
data := bytes.NewBuffer([]byte{jsonReq})
req, err := http.NewRequest("POST", "/users/login", data)
req.Header = headers
client := &http.Client{}
resp, err := client.Do(req)
// ...
}
'application/x-www-form-urlencoded',
'Accept' => '*/*',
);
$client = new \GuzzleHttp\Client();
// Define array of request body.
$request_body = array();
try {
$response = $client->request('POST','/users/login', array(
'headers' => $headers,
'json' => $request_body,
)
);
print_r($response->getBody()->getContents());
}
catch (\GuzzleHttp\Exception\BadResponseException $e) {
// handle exception or api errors.
print_r($e->getMessage());
}
// ...
POST /users/login
Login with REST API
Body parameter
username: string
password: pa$$word
Parameters
Name | In | Type | Required | Description |
---|---|---|---|---|
body | body | object | true | none |
» username | body | string | true | Your username |
» password | body | string(password) | true | Your password |
Example responses
200 Response
Responses
Status | Meaning | Description | Schema |
---|---|---|---|
200 | OK | Successful authentication | Inline |
400 | Bad Request | Error in authentication | Inline |
default | Default | Error in authentication | None |
Response Schema
Status Code 200
Name | Type | Required | Restrictions | Description |
---|---|---|---|---|
» id | string | false | none | none |
» token | string | false | none | none |
» tokenExpires | string | false | none | none |
Status Code 400
Name | Type | Required | Restrictions | Description |
---|---|---|---|---|
» error | number | false | none | none |
» reason | string | false | none | none |
register
Code samples
# You can also use wget
curl -X POST /users/register \
-H 'Content-Type: application/x-www-form-urlencoded' \
-H 'Accept: */*'
POST /users/register HTTP/1.1
Content-Type: application/x-www-form-urlencoded
Accept: */*
const inputBody = '{
"username": "string",
"password": "pa$$word",
"email": "string"
}';
const headers = {
'Content-Type':'application/x-www-form-urlencoded',
'Accept':'*/*'
};
fetch('/users/register',
{
method: 'POST',
body: inputBody,
headers: headers
})
.then(function(res) {
return res.json();
}).then(function(body) {
console.log(body);
});
const fetch = require('node-fetch');
const inputBody = {
"username": "string",
"password": "pa$$word",
"email": "string"
};
const headers = {
'Content-Type':'application/x-www-form-urlencoded',
'Accept':'*/*'
};
fetch('/users/register',
{
method: 'POST',
body: JSON.stringify(inputBody),
headers: headers
})
.then(function(res) {
return res.json();
}).then(function(body) {
console.log(body);
});
require 'rest-client'
require 'json'
headers = {
'Content-Type' => 'application/x-www-form-urlencoded',
'Accept' => '*/*'
}
result = RestClient.post '/users/register',
params: {
}, headers: headers
p JSON.parse(result)
import requests
headers = {
'Content-Type': 'application/x-www-form-urlencoded',
'Accept': '*/*'
}
r = requests.post('/users/register', headers = headers)
print(r.json())
URL obj = new URL("/users/register");
HttpURLConnection con = (HttpURLConnection) obj.openConnection();
con.setRequestMethod("POST");
int responseCode = con.getResponseCode();
BufferedReader in = new BufferedReader(
new InputStreamReader(con.getInputStream()));
String inputLine;
StringBuffer response = new StringBuffer();
while ((inputLine = in.readLine()) != null) {
response.append(inputLine);
}
in.close();
System.out.println(response.toString());
package main
import (
"bytes"
"net/http"
)
func main() {
headers := map[string][]string{
"Content-Type": []string{"application/x-www-form-urlencoded"},
"Accept": []string{"*/*"},
}
data := bytes.NewBuffer([]byte{jsonReq})
req, err := http.NewRequest("POST", "/users/register", data)
req.Header = headers
client := &http.Client{}
resp, err := client.Do(req)
// ...
}
'application/x-www-form-urlencoded',
'Accept' => '*/*',
);
$client = new \GuzzleHttp\Client();
// Define array of request body.
$request_body = array();
try {
$response = $client->request('POST','/users/register', array(
'headers' => $headers,
'json' => $request_body,
)
);
print_r($response->getBody()->getContents());
}
catch (\GuzzleHttp\Exception\BadResponseException $e) {
// handle exception or api errors.
print_r($e->getMessage());
}
// ...
POST /users/register
Register with REST API
Notes:
- You will need to provide the token for any of the authenticated methods.
Body parameter
username: string
password: pa$$word
email: string
Parameters
Name | In | Type | Required | Description |
---|---|---|---|---|
body | body | object | true | none |
» username | body | string | true | Your username |
» password | body | string(password) | true | Your password |
body | string | true | Your email |
Example responses
200 Response
Responses
Status | Meaning | Description | Schema |
---|---|---|---|
200 | OK | Successful registration | Inline |
400 | Bad Request | Error in registration | Inline |
default | Default | Error in registration | None |
Response Schema
Status Code 200
Name | Type | Required | Restrictions | Description |
---|---|---|---|---|
» id | string | false | none | none |
» token | string | false | none | none |
» tokenExpires | string | false | none | none |
Status Code 400
Name | Type | Required | Restrictions | Description |
---|---|---|---|---|
» error | number | false | none | none |
» reason | string | false | none | none |
Boards
get_public_boards
Code samples
# You can also use wget
curl -X GET /api/boards \
-H 'Accept: application/json' \
-H 'Authorization: API_KEY'
GET /api/boards HTTP/1.1
Accept: application/json
const headers = {
'Accept':'application/json',
'Authorization':'API_KEY'
};
fetch('/api/boards',
{
method: 'GET',
headers: headers
})
.then(function(res) {
return res.json();
}).then(function(body) {
console.log(body);
});
const fetch = require('node-fetch');
const headers = {
'Accept':'application/json',
'Authorization':'API_KEY'
};
fetch('/api/boards',
{
method: 'GET',
headers: headers
})
.then(function(res) {
return res.json();
}).then(function(body) {
console.log(body);
});
require 'rest-client'
require 'json'
headers = {
'Accept' => 'application/json',
'Authorization' => 'API_KEY'
}
result = RestClient.get '/api/boards',
params: {
}, headers: headers
p JSON.parse(result)
import requests
headers = {
'Accept': 'application/json',
'Authorization': 'API_KEY'
}
r = requests.get('/api/boards', headers = headers)
print(r.json())
URL obj = new URL("/api/boards");
HttpURLConnection con = (HttpURLConnection) obj.openConnection();
con.setRequestMethod("GET");
int responseCode = con.getResponseCode();
BufferedReader in = new BufferedReader(
new InputStreamReader(con.getInputStream()));
String inputLine;
StringBuffer response = new StringBuffer();
while ((inputLine = in.readLine()) != null) {
response.append(inputLine);
}
in.close();
System.out.println(response.toString());
package main
import (
"bytes"
"net/http"
)
func main() {
headers := map[string][]string{
"Accept": []string{"application/json"},
"Authorization": []string{"API_KEY"},
}
data := bytes.NewBuffer([]byte{jsonReq})
req, err := http.NewRequest("GET", "/api/boards", data)
req.Header = headers
client := &http.Client{}
resp, err := client.Do(req)
// ...
}
'application/json',
'Authorization' => 'API_KEY',
);
$client = new \GuzzleHttp\Client();
// Define array of request body.
$request_body = array();
try {
$response = $client->request('GET','/api/boards', array(
'headers' => $headers,
'json' => $request_body,
)
);
print_r($response->getBody()->getContents());
}
catch (\GuzzleHttp\Exception\BadResponseException $e) {
// handle exception or api errors.
print_r($e->getMessage());
}
// ...
GET /api/boards
Get all public boards
Example responses
200 Response
[
{
"_id": "string",
"title": "string"
}
]
Responses
Status | Meaning | Description | Schema |
---|---|---|---|
200 | OK | 200 response | Inline |
Response Schema
Status Code 200
Name | Type | Required | Restrictions | Description |
---|---|---|---|---|
» _id | string | false | none | none |
» title | string | false | none | none |
new_board
Code samples
# You can also use wget
curl -X POST /api/boards \
-H 'Content-Type: multipart/form-data' \
-H 'Accept: application/json' \
-H 'Authorization: API_KEY'
POST /api/boards HTTP/1.1
Content-Type: multipart/form-data
Accept: application/json
const inputBody = '{
"title": "string",
"owner": "string",
"isAdmin": true,
"isActive": true,
"isNoComments": true,
"isCommentOnly": true,
"isWorker": true,
"permission": "string",
"color": "string"
}';
const headers = {
'Content-Type':'multipart/form-data',
'Accept':'application/json',
'Authorization':'API_KEY'
};
fetch('/api/boards',
{
method: 'POST',
body: inputBody,
headers: headers
})
.then(function(res) {
return res.json();
}).then(function(body) {
console.log(body);
});
const fetch = require('node-fetch');
const inputBody = {
"title": "string",
"owner": "string",
"isAdmin": true,
"isActive": true,
"isNoComments": true,
"isCommentOnly": true,
"isWorker": true,
"permission": "string",
"color": "string"
};
const headers = {
'Content-Type':'multipart/form-data',
'Accept':'application/json',
'Authorization':'API_KEY'
};
fetch('/api/boards',
{
method: 'POST',
body: JSON.stringify(inputBody),
headers: headers
})
.then(function(res) {
return res.json();
}).then(function(body) {
console.log(body);
});
require 'rest-client'
require 'json'
headers = {
'Content-Type' => 'multipart/form-data',
'Accept' => 'application/json',
'Authorization' => 'API_KEY'
}
result = RestClient.post '/api/boards',
params: {
}, headers: headers
p JSON.parse(result)
import requests
headers = {
'Content-Type': 'multipart/form-data',
'Accept': 'application/json',
'Authorization': 'API_KEY'
}
r = requests.post('/api/boards', headers = headers)
print(r.json())
URL obj = new URL("/api/boards");
HttpURLConnection con = (HttpURLConnection) obj.openConnection();
con.setRequestMethod("POST");
int responseCode = con.getResponseCode();
BufferedReader in = new BufferedReader(
new InputStreamReader(con.getInputStream()));
String inputLine;
StringBuffer response = new StringBuffer();
while ((inputLine = in.readLine()) != null) {
response.append(inputLine);
}
in.close();
System.out.println(response.toString());
package main
import (
"bytes"
"net/http"
)
func main() {
headers := map[string][]string{
"Content-Type": []string{"multipart/form-data"},
"Accept": []string{"application/json"},
"Authorization": []string{"API_KEY"},
}
data := bytes.NewBuffer([]byte{jsonReq})
req, err := http.NewRequest("POST", "/api/boards", data)
req.Header = headers
client := &http.Client{}
resp, err := client.Do(req)
// ...
}
'multipart/form-data',
'Accept' => 'application/json',
'Authorization' => 'API_KEY',
);
$client = new \GuzzleHttp\Client();
// Define array of request body.
$request_body = array();
try {
$response = $client->request('POST','/api/boards', array(
'headers' => $headers,
'json' => $request_body,
)
);
print_r($response->getBody()->getContents());
}
catch (\GuzzleHttp\Exception\BadResponseException $e) {
// handle exception or api errors.
print_r($e->getMessage());
}
// ...
POST /api/boards
Create a board
This allows to create a board.
The color has to be chosen between belize
, nephritis
, pomegranate
,
pumpkin
, wisteria
, moderatepink
, strongcyan
,
limegreen
, midnight
, dark
, relax
, corteza
:
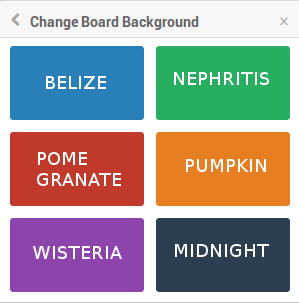
Body parameter
title: string
owner: string
isAdmin: true
isActive: true
isNoComments: true
isCommentOnly: true
isWorker: true
permission: string
color: string
Parameters
Name | In | Type | Required | Description |
---|---|---|---|---|
body | body | object | true | none |
» title | body | string | true | the new title of the board |
» owner | body | string | true | "ABCDE12345" <= User ID in Wekan. |
» isAdmin | body | boolean | false | is the owner an admin of the board (default true) |
» isActive | body | boolean | false | is the board active (default true) |
» isNoComments | body | boolean | false | disable comments (default false) |
» isCommentOnly | body | boolean | false | only enable comments (default false) |
» isWorker | body | boolean | false | only move cards, assign himself to card and comment (default false) |
» permission | body | string | false | "private" board <== Set to "public" if you |
» color | body | string | false | the color of the board |
Detailed descriptions
» owner: "ABCDE12345" <= User ID in Wekan. (Not username or email)
» permission: "private" board <== Set to "public" if you want public Wekan board
Example responses
200 Response
{
"_id": "string",
"defaultSwimlaneId": "string"
}
Responses
Status | Meaning | Description | Schema |
---|---|---|---|
200 | OK | 200 response | Inline |
Response Schema
Status Code 200
Name | Type | Required | Restrictions | Description |
---|---|---|---|---|
» _id | string | false | none | none |
» defaultSwimlaneId | string | false | none | none |
get_board
Code samples
# You can also use wget
curl -X GET /api/boards/{board} \
-H 'Accept: application/json' \
-H 'Authorization: API_KEY'
GET /api/boards/{board} HTTP/1.1
Accept: application/json
const headers = {
'Accept':'application/json',
'Authorization':'API_KEY'
};
fetch('/api/boards/{board}',
{
method: 'GET',
headers: headers
})
.then(function(res) {
return res.json();
}).then(function(body) {
console.log(body);
});
const fetch = require('node-fetch');
const headers = {
'Accept':'application/json',
'Authorization':'API_KEY'
};
fetch('/api/boards/{board}',
{
method: 'GET',
headers: headers
})
.then(function(res) {
return res.json();
}).then(function(body) {
console.log(body);
});
require 'rest-client'
require 'json'
headers = {
'Accept' => 'application/json',
'Authorization' => 'API_KEY'
}
result = RestClient.get '/api/boards/{board}',
params: {
}, headers: headers
p JSON.parse(result)
import requests
headers = {
'Accept': 'application/json',
'Authorization': 'API_KEY'
}
r = requests.get('/api/boards/{board}', headers = headers)
print(r.json())
URL obj = new URL("/api/boards/{board}");
HttpURLConnection con = (HttpURLConnection) obj.openConnection();
con.setRequestMethod("GET");
int responseCode = con.getResponseCode();
BufferedReader in = new BufferedReader(
new InputStreamReader(con.getInputStream()));
String inputLine;
StringBuffer response = new StringBuffer();
while ((inputLine = in.readLine()) != null) {
response.append(inputLine);
}
in.close();
System.out.println(response.toString());
package main
import (
"bytes"
"net/http"
)
func main() {
headers := map[string][]string{
"Accept": []string{"application/json"},
"Authorization": []string{"API_KEY"},
}
data := bytes.NewBuffer([]byte{jsonReq})
req, err := http.NewRequest("GET", "/api/boards/{board}", data)
req.Header = headers
client := &http.Client{}
resp, err := client.Do(req)
// ...
}
'application/json',
'Authorization' => 'API_KEY',
);
$client = new \GuzzleHttp\Client();
// Define array of request body.
$request_body = array();
try {
$response = $client->request('GET','/api/boards/{board}', array(
'headers' => $headers,
'json' => $request_body,
)
);
print_r($response->getBody()->getContents());
}
catch (\GuzzleHttp\Exception\BadResponseException $e) {
// handle exception or api errors.
print_r($e->getMessage());
}
// ...
GET /api/boards/{board}
Get the board with that particular ID
Parameters
Name | In | Type | Required | Description |
---|---|---|---|---|
board | path | string | true | the ID of the board to retrieve the data |
Detailed descriptions
board: the ID of the board to retrieve the data
Example responses
200 Response
{
"title": "string",
"slug": "string",
"archived": true,
"archivedAt": "string",
"createdAt": "string",
"modifiedAt": "string",
"stars": 0,
"labels": [
{
"_id": "string",
"name": "string",
"color": "white"
}
],
"members": [
{
"userId": "string",
"isAdmin": true,
"isActive": true,
"isNoComments": true,
"isCommentOnly": true,
"isWorker": true
}
],
"permission": "public",
"orgs": [
{
"orgId": "string",
"orgDisplayName": "string",
"isActive": true
}
],
"teams": [
{
"teamId": "string",
"teamDisplayName": "string",
"isActive": true
}
],
"color": "belize",
"backgroundImageURL": "string",
"allowsCardCounterList": true,
"allowsBoardMemberList": true,
"description": "string",
"subtasksDefaultBoardId": "string",
"subtasksDefaultListId": "string",
"dateSettingsDefaultBoardId": "string",
"dateSettingsDefaultListId": "string",
"allowsSubtasks": true,
"allowsAttachments": true,
"allowsChecklists": true,
"allowsComments": true,
"allowsDescriptionTitle": true,
"allowsDescriptionText": true,
"allowsDescriptionTextOnMinicard": true,
"allowsCoverAttachmentOnMinicard": true,
"allowsBadgeAttachmentOnMinicard": true,
"allowsCardSortingByNumberOnMinicard": true,
"allowsCardNumber": true,
"allowsActivities": true,
"allowsLabels": true,
"allowsCreator": true,
"allowsCreatorOnMinicard": true,
"allowsAssignee": true,
"allowsMembers": true,
"allowsRequestedBy": true,
"allowsCardSortingByNumber": true,
"allowsShowLists": true,
"allowsAssignedBy": true,
"allowsReceivedDate": true,
"allowsStartDate": true,
"allowsEndDate": true,
"allowsDueDate": true,
"presentParentTask": "prefix-with-full-path",
"receivedAt": "string",
"startAt": "string",
"dueAt": "string",
"endAt": "string",
"spentTime": 0,
"isOvertime": true,
"type": "board",
"sort": 0
}
Responses
Status | Meaning | Description | Schema |
---|---|---|---|
200 | OK | 200 response | Boards |
delete_board
Code samples
# You can also use wget
curl -X DELETE /api/boards/{board} \
-H 'Authorization: API_KEY'
DELETE /api/boards/{board} HTTP/1.1
const headers = {
'Authorization':'API_KEY'
};
fetch('/api/boards/{board}',
{
method: 'DELETE',
headers: headers
})
.then(function(res) {
return res.json();
}).then(function(body) {
console.log(body);
});
const fetch = require('node-fetch');
const headers = {
'Authorization':'API_KEY'
};
fetch('/api/boards/{board}',
{
method: 'DELETE',
headers: headers
})
.then(function(res) {
return res.json();
}).then(function(body) {
console.log(body);
});
require 'rest-client'
require 'json'
headers = {
'Authorization' => 'API_KEY'
}
result = RestClient.delete '/api/boards/{board}',
params: {
}, headers: headers
p JSON.parse(result)
import requests
headers = {
'Authorization': 'API_KEY'
}
r = requests.delete('/api/boards/{board}', headers = headers)
print(r.json())
URL obj = new URL("/api/boards/{board}");
HttpURLConnection con = (HttpURLConnection) obj.openConnection();
con.setRequestMethod("DELETE");
int responseCode = con.getResponseCode();
BufferedReader in = new BufferedReader(
new InputStreamReader(con.getInputStream()));
String inputLine;
StringBuffer response = new StringBuffer();
while ((inputLine = in.readLine()) != null) {
response.append(inputLine);
}
in.close();
System.out.println(response.toString());
package main
import (
"bytes"
"net/http"
)
func main() {
headers := map[string][]string{
"Authorization": []string{"API_KEY"},
}
data := bytes.NewBuffer([]byte{jsonReq})
req, err := http.NewRequest("DELETE", "/api/boards/{board}", data)
req.Header = headers
client := &http.Client{}
resp, err := client.Do(req)
// ...
}
'API_KEY',
);
$client = new \GuzzleHttp\Client();
// Define array of request body.
$request_body = array();
try {
$response = $client->request('DELETE','/api/boards/{board}', array(
'headers' => $headers,
'json' => $request_body,
)
);
print_r($response->getBody()->getContents());
}
catch (\GuzzleHttp\Exception\BadResponseException $e) {
// handle exception or api errors.
print_r($e->getMessage());
}
// ...
DELETE /api/boards/{board}
Delete a board
Parameters
Name | In | Type | Required | Description |
---|---|---|---|---|
board | path | string | true | the ID of the board |
Detailed descriptions
board: the ID of the board
Responses
Status | Meaning | Description | Schema |
---|---|---|---|
200 | OK | 200 response | None |
get_board_attachments
Code samples
# You can also use wget
curl -X GET /api/boards/{board}/attachments \
-H 'Accept: application/json' \
-H 'Authorization: API_KEY'
GET /api/boards/{board}/attachments HTTP/1.1
Accept: application/json
const headers = {
'Accept':'application/json',
'Authorization':'API_KEY'
};
fetch('/api/boards/{board}/attachments',
{
method: 'GET',
headers: headers
})
.then(function(res) {
return res.json();
}).then(function(body) {
console.log(body);
});
const fetch = require('node-fetch');
const headers = {
'Accept':'application/json',
'Authorization':'API_KEY'
};
fetch('/api/boards/{board}/attachments',
{
method: 'GET',
headers: headers
})
.then(function(res) {
return res.json();
}).then(function(body) {
console.log(body);
});
require 'rest-client'
require 'json'
headers = {
'Accept' => 'application/json',
'Authorization' => 'API_KEY'
}
result = RestClient.get '/api/boards/{board}/attachments',
params: {
}, headers: headers
p JSON.parse(result)
import requests
headers = {
'Accept': 'application/json',
'Authorization': 'API_KEY'
}
r = requests.get('/api/boards/{board}/attachments', headers = headers)
print(r.json())
URL obj = new URL("/api/boards/{board}/attachments");
HttpURLConnection con = (HttpURLConnection) obj.openConnection();
con.setRequestMethod("GET");
int responseCode = con.getResponseCode();
BufferedReader in = new BufferedReader(
new InputStreamReader(con.getInputStream()));
String inputLine;
StringBuffer response = new StringBuffer();
while ((inputLine = in.readLine()) != null) {
response.append(inputLine);
}
in.close();
System.out.println(response.toString());
package main
import (
"bytes"
"net/http"
)
func main() {
headers := map[string][]string{
"Accept": []string{"application/json"},
"Authorization": []string{"API_KEY"},
}
data := bytes.NewBuffer([]byte{jsonReq})
req, err := http.NewRequest("GET", "/api/boards/{board}/attachments", data)
req.Header = headers
client := &http.Client{}
resp, err := client.Do(req)
// ...
}
'application/json',
'Authorization' => 'API_KEY',
);
$client = new \GuzzleHttp\Client();
// Define array of request body.
$request_body = array();
try {
$response = $client->request('GET','/api/boards/{board}/attachments', array(
'headers' => $headers,
'json' => $request_body,
)
);
print_r($response->getBody()->getContents());
}
catch (\GuzzleHttp\Exception\BadResponseException $e) {
// handle exception or api errors.
print_r($e->getMessage());
}
// ...
GET /api/boards/{board}/attachments
Get the list of attachments of a board
Parameters
Name | In | Type | Required | Description |
---|---|---|---|---|
board | path | string | true | the board ID |
Detailed descriptions
board: the board ID
Example responses
200 Response
[
{
"attachmentId": "string",
"attachmentName": "string",
"attachmentType": "string",
"url": "string",
"urlDownload": "string",
"boardId": "string",
"swimlaneId": "string",
"listId": "string",
"cardId": "string"
}
]
Responses
Status | Meaning | Description | Schema |
---|---|---|---|
200 | OK | 200 response | Inline |
Response Schema
Status Code 200
Name | Type | Required | Restrictions | Description |
---|---|---|---|---|
» attachmentId | string | false | none | none |
» attachmentName | string | false | none | none |
» attachmentType | string | false | none | none |
» url | string | false | none | none |
» urlDownload | string | false | none | none |
» boardId | string | false | none | none |
» swimlaneId | string | false | none | none |
» listId | string | false | none | none |
» cardId | string | false | none | none |
exportJson
Code samples
# You can also use wget
curl -X GET /api/boards/{board}/export \
-H 'Authorization: API_KEY'
GET /api/boards/{board}/export HTTP/1.1
const headers = {
'Authorization':'API_KEY'
};
fetch('/api/boards/{board}/export',
{
method: 'GET',
headers: headers
})
.then(function(res) {
return res.json();
}).then(function(body) {
console.log(body);
});
const fetch = require('node-fetch');
const headers = {
'Authorization':'API_KEY'
};
fetch('/api/boards/{board}/export',
{
method: 'GET',
headers: headers
})
.then(function(res) {
return res.json();
}).then(function(body) {
console.log(body);
});
require 'rest-client'
require 'json'
headers = {
'Authorization' => 'API_KEY'
}
result = RestClient.get '/api/boards/{board}/export',
params: {
}, headers: headers
p JSON.parse(result)
import requests
headers = {
'Authorization': 'API_KEY'
}
r = requests.get('/api/boards/{board}/export', headers = headers)
print(r.json())
URL obj = new URL("/api/boards/{board}/export");
HttpURLConnection con = (HttpURLConnection) obj.openConnection();
con.setRequestMethod("GET");
int responseCode = con.getResponseCode();
BufferedReader in = new BufferedReader(
new InputStreamReader(con.getInputStream()));
String inputLine;
StringBuffer response = new StringBuffer();
while ((inputLine = in.readLine()) != null) {
response.append(inputLine);
}
in.close();
System.out.println(response.toString());
package main
import (
"bytes"
"net/http"
)
func main() {
headers := map[string][]string{
"Authorization": []string{"API_KEY"},
}
data := bytes.NewBuffer([]byte{jsonReq})
req, err := http.NewRequest("GET", "/api/boards/{board}/export", data)
req.Header = headers
client := &http.Client{}
resp, err := client.Do(req)
// ...
}
'API_KEY',
);
$client = new \GuzzleHttp\Client();
// Define array of request body.
$request_body = array();
try {
$response = $client->request('GET','/api/boards/{board}/export', array(
'headers' => $headers,
'json' => $request_body,
)
);
print_r($response->getBody()->getContents());
}
catch (\GuzzleHttp\Exception\BadResponseException $e) {
// handle exception or api errors.
print_r($e->getMessage());
}
// ...
GET /api/boards/{board}/export
This route is used to export the board to a json file format.
If user is already logged-in, pass loginToken as param "authToken": '/api/boards/:boardId/export?authToken=:token'
See https://blog.kayla.com.au/server-side-route-authentication-in-meteor/ for detailed explanations
Parameters
Name | In | Type | Required | Description |
---|---|---|---|---|
board | path | string | true | the ID of the board we are exporting |
Detailed descriptions
board: the ID of the board we are exporting
Responses
Status | Meaning | Description | Schema |
---|---|---|---|
200 | OK | 200 response | None |
copy_board
Code samples
# You can also use wget
curl -X POST /api/boards/{board}/copy \
-H 'Content-Type: multipart/form-data' \
-H 'Accept: application/json' \
-H 'Authorization: API_KEY'
POST /api/boards/{board}/copy HTTP/1.1
Content-Type: multipart/form-data
Accept: application/json
const inputBody = '{
"title": "string"
}';
const headers = {
'Content-Type':'multipart/form-data',
'Accept':'application/json',
'Authorization':'API_KEY'
};
fetch('/api/boards/{board}/copy',
{
method: 'POST',
body: inputBody,
headers: headers
})
.then(function(res) {
return res.json();
}).then(function(body) {
console.log(body);
});
const fetch = require('node-fetch');
const inputBody = {
"title": "string"
};
const headers = {
'Content-Type':'multipart/form-data',
'Accept':'application/json',
'Authorization':'API_KEY'
};
fetch('/api/boards/{board}/copy',
{
method: 'POST',
body: JSON.stringify(inputBody),
headers: headers
})
.then(function(res) {
return res.json();
}).then(function(body) {
console.log(body);
});
require 'rest-client'
require 'json'
headers = {
'Content-Type' => 'multipart/form-data',
'Accept' => 'application/json',
'Authorization' => 'API_KEY'
}
result = RestClient.post '/api/boards/{board}/copy',
params: {
}, headers: headers
p JSON.parse(result)
import requests
headers = {
'Content-Type': 'multipart/form-data',
'Accept': 'application/json',
'Authorization': 'API_KEY'
}
r = requests.post('/api/boards/{board}/copy', headers = headers)
print(r.json())
URL obj = new URL("/api/boards/{board}/copy");
HttpURLConnection con = (HttpURLConnection) obj.openConnection();
con.setRequestMethod("POST");
int responseCode = con.getResponseCode();
BufferedReader in = new BufferedReader(
new InputStreamReader(con.getInputStream()));
String inputLine;
StringBuffer response = new StringBuffer();
while ((inputLine = in.readLine()) != null) {
response.append(inputLine);
}
in.close();
System.out.println(response.toString());
package main
import (
"bytes"
"net/http"
)
func main() {
headers := map[string][]string{
"Content-Type": []string{"multipart/form-data"},
"Accept": []string{"application/json"},
"Authorization": []string{"API_KEY"},
}
data := bytes.NewBuffer([]byte{jsonReq})
req, err := http.NewRequest("POST", "/api/boards/{board}/copy", data)
req.Header = headers
client := &http.Client{}
resp, err := client.Do(req)
// ...
}
'multipart/form-data',
'Accept' => 'application/json',
'Authorization' => 'API_KEY',
);
$client = new \GuzzleHttp\Client();
// Define array of request body.
$request_body = array();
try {
$response = $client->request('POST','/api/boards/{board}/copy', array(
'headers' => $headers,
'json' => $request_body,
)
);
print_r($response->getBody()->getContents());
}
catch (\GuzzleHttp\Exception\BadResponseException $e) {
// handle exception or api errors.
print_r($e->getMessage());
}
// ...
POST /api/boards/{board}/copy
Copy a board to a new one
If your are board admin or wekan admin, this copies the given board to a new one.
Body parameter
title: string
Parameters
Name | In | Type | Required | Description |
---|---|---|---|---|
board | path | string | true | the board |
body | body | object | true | none |
» title | body | string | true | the title of the new board (default to old one) |
Detailed descriptions
board: the board
Example responses
200 Response
"string"
Responses
Status | Meaning | Description | Schema |
---|---|---|---|
200 | OK | 200 response | string |
add_board_label
Code samples
# You can also use wget
curl -X PUT /api/boards/{board}/labels \
-H 'Content-Type: multipart/form-data' \
-H 'Accept: application/json' \
-H 'Authorization: API_KEY'
PUT /api/boards/{board}/labels HTTP/1.1
Content-Type: multipart/form-data
Accept: application/json
const inputBody = '{
"label": "string"
}';
const headers = {
'Content-Type':'multipart/form-data',
'Accept':'application/json',
'Authorization':'API_KEY'
};
fetch('/api/boards/{board}/labels',
{
method: 'PUT',
body: inputBody,
headers: headers
})
.then(function(res) {
return res.json();
}).then(function(body) {
console.log(body);
});
const fetch = require('node-fetch');
const inputBody = {
"label": "string"
};
const headers = {
'Content-Type':'multipart/form-data',
'Accept':'application/json',
'Authorization':'API_KEY'
};
fetch('/api/boards/{board}/labels',
{
method: 'PUT',
body: JSON.stringify(inputBody),
headers: headers
})
.then(function(res) {
return res.json();
}).then(function(body) {
console.log(body);
});
require 'rest-client'
require 'json'
headers = {
'Content-Type' => 'multipart/form-data',
'Accept' => 'application/json',
'Authorization' => 'API_KEY'
}
result = RestClient.put '/api/boards/{board}/labels',
params: {
}, headers: headers
p JSON.parse(result)
import requests
headers = {
'Content-Type': 'multipart/form-data',
'Accept': 'application/json',
'Authorization': 'API_KEY'
}
r = requests.put('/api/boards/{board}/labels', headers = headers)
print(r.json())
URL obj = new URL("/api/boards/{board}/labels");
HttpURLConnection con = (HttpURLConnection) obj.openConnection();
con.setRequestMethod("PUT");
int responseCode = con.getResponseCode();
BufferedReader in = new BufferedReader(
new InputStreamReader(con.getInputStream()));
String inputLine;
StringBuffer response = new StringBuffer();
while ((inputLine = in.readLine()) != null) {
response.append(inputLine);
}
in.close();
System.out.println(response.toString());
package main
import (
"bytes"
"net/http"
)
func main() {
headers := map[string][]string{
"Content-Type": []string{"multipart/form-data"},
"Accept": []string{"application/json"},
"Authorization": []string{"API_KEY"},
}
data := bytes.NewBuffer([]byte{jsonReq})
req, err := http.NewRequest("PUT", "/api/boards/{board}/labels", data)
req.Header = headers
client := &http.Client{}
resp, err := client.Do(req)
// ...
}
'multipart/form-data',
'Accept' => 'application/json',
'Authorization' => 'API_KEY',
);
$client = new \GuzzleHttp\Client();
// Define array of request body.
$request_body = array();
try {
$response = $client->request('PUT','/api/boards/{board}/labels', array(
'headers' => $headers,
'json' => $request_body,
)
);
print_r($response->getBody()->getContents());
}
catch (\GuzzleHttp\Exception\BadResponseException $e) {
// handle exception or api errors.
print_r($e->getMessage());
}
// ...
PUT /api/boards/{board}/labels
Add a label to a board
If the board doesn't have the name/color label, this function adds the label to the board.
Body parameter
label: string
Parameters
Name | In | Type | Required | Description |
---|---|---|---|---|
board | path | string | true | the board |
body | body | object | true | none |
» label | body | string | true | the label value |
Detailed descriptions
board: the board
Example responses
200 Response
"string"
Responses
Status | Meaning | Description | Schema |
---|---|---|---|
200 | OK | 200 response | string |
set_board_member_permission
Code samples
# You can also use wget
curl -X POST /api/boards/{board}/members/{member} \
-H 'Content-Type: multipart/form-data' \
-H 'Authorization: API_KEY'
POST /api/boards/{board}/members/{member} HTTP/1.1
Content-Type: multipart/form-data
const inputBody = '{
"isAdmin": true,
"isNoComments": true,
"isCommentOnly": true,
"isWorker": true
}';
const headers = {
'Content-Type':'multipart/form-data',
'Authorization':'API_KEY'
};
fetch('/api/boards/{board}/members/{member}',
{
method: 'POST',
body: inputBody,
headers: headers
})
.then(function(res) {
return res.json();
}).then(function(body) {
console.log(body);
});
const fetch = require('node-fetch');
const inputBody = {
"isAdmin": true,
"isNoComments": true,
"isCommentOnly": true,
"isWorker": true
};
const headers = {
'Content-Type':'multipart/form-data',
'Authorization':'API_KEY'
};
fetch('/api/boards/{board}/members/{member}',
{
method: 'POST',
body: JSON.stringify(inputBody),
headers: headers
})
.then(function(res) {
return res.json();
}).then(function(body) {
console.log(body);
});
require 'rest-client'
require 'json'
headers = {
'Content-Type' => 'multipart/form-data',
'Authorization' => 'API_KEY'
}
result = RestClient.post '/api/boards/{board}/members/{member}',
params: {
}, headers: headers
p JSON.parse(result)
import requests
headers = {
'Content-Type': 'multipart/form-data',
'Authorization': 'API_KEY'
}
r = requests.post('/api/boards/{board}/members/{member}', headers = headers)
print(r.json())
URL obj = new URL("/api/boards/{board}/members/{member}");
HttpURLConnection con = (HttpURLConnection) obj.openConnection();
con.setRequestMethod("POST");
int responseCode = con.getResponseCode();
BufferedReader in = new BufferedReader(
new InputStreamReader(con.getInputStream()));
String inputLine;
StringBuffer response = new StringBuffer();
while ((inputLine = in.readLine()) != null) {
response.append(inputLine);
}
in.close();
System.out.println(response.toString());
package main
import (
"bytes"
"net/http"
)
func main() {
headers := map[string][]string{
"Content-Type": []string{"multipart/form-data"},
"Authorization": []string{"API_KEY"},
}
data := bytes.NewBuffer([]byte{jsonReq})
req, err := http.NewRequest("POST", "/api/boards/{board}/members/{member}", data)
req.Header = headers
client := &http.Client{}
resp, err := client.Do(req)
// ...
}
'multipart/form-data',
'Authorization' => 'API_KEY',
);
$client = new \GuzzleHttp\Client();
// Define array of request body.
$request_body = array();
try {
$response = $client->request('POST','/api/boards/{board}/members/{member}', array(
'headers' => $headers,
'json' => $request_body,
)
);
print_r($response->getBody()->getContents());
}
catch (\GuzzleHttp\Exception\BadResponseException $e) {
// handle exception or api errors.
print_r($e->getMessage());
}
// ...
POST /api/boards/{board}/members/{member}
Change the permission of a member of a board
Body parameter
isAdmin: true
isNoComments: true
isCommentOnly: true
isWorker: true
Parameters
Name | In | Type | Required | Description |
---|---|---|---|---|
board | path | string | true | the ID of the board that we are changing |
member | path | string | true | the ID of the user to change permissions |
body | body | object | true | none |
» isAdmin | body | boolean | true | admin capability |
» isNoComments | body | boolean | true | NoComments capability |
» isCommentOnly | body | boolean | true | CommentsOnly capability |
» isWorker | body | boolean | true | Worker capability |
Detailed descriptions
board: the ID of the board that we are changing
member: the ID of the user to change permissions
Responses
Status | Meaning | Description | Schema |
---|---|---|---|
200 | OK | 200 response | None |
update_board_title
Code samples
# You can also use wget
curl -X PUT /api/boards/{board}/title \
-H 'Content-Type: multipart/form-data' \
-H 'Authorization: API_KEY'
PUT /api/boards/{board}/title HTTP/1.1
Content-Type: multipart/form-data
const inputBody = '{
"title": "string"
}';
const headers = {
'Content-Type':'multipart/form-data',
'Authorization':'API_KEY'
};
fetch('/api/boards/{board}/title',
{
method: 'PUT',
body: inputBody,
headers: headers
})
.then(function(res) {
return res.json();
}).then(function(body) {
console.log(body);
});
const fetch = require('node-fetch');
const inputBody = {
"title": "string"
};
const headers = {
'Content-Type':'multipart/form-data',
'Authorization':'API_KEY'
};
fetch('/api/boards/{board}/title',
{
method: 'PUT',
body: JSON.stringify(inputBody),
headers: headers
})
.then(function(res) {
return res.json();
}).then(function(body) {
console.log(body);
});
require 'rest-client'
require 'json'
headers = {
'Content-Type' => 'multipart/form-data',
'Authorization' => 'API_KEY'
}
result = RestClient.put '/api/boards/{board}/title',
params: {
}, headers: headers
p JSON.parse(result)
import requests
headers = {
'Content-Type': 'multipart/form-data',
'Authorization': 'API_KEY'
}
r = requests.put('/api/boards/{board}/title', headers = headers)
print(r.json())
URL obj = new URL("/api/boards/{board}/title");
HttpURLConnection con = (HttpURLConnection) obj.openConnection();
con.setRequestMethod("PUT");
int responseCode = con.getResponseCode();
BufferedReader in = new BufferedReader(
new InputStreamReader(con.getInputStream()));
String inputLine;
StringBuffer response = new StringBuffer();
while ((inputLine = in.readLine()) != null) {
response.append(inputLine);
}
in.close();
System.out.println(response.toString());
package main
import (
"bytes"
"net/http"
)
func main() {
headers := map[string][]string{
"Content-Type": []string{"multipart/form-data"},
"Authorization": []string{"API_KEY"},
}
data := bytes.NewBuffer([]byte{jsonReq})
req, err := http.NewRequest("PUT", "/api/boards/{board}/title", data)
req.Header = headers
client := &http.Client{}
resp, err := client.Do(req)
// ...
}
'multipart/form-data',
'Authorization' => 'API_KEY',
);
$client = new \GuzzleHttp\Client();
// Define array of request body.
$request_body = array();
try {
$response = $client->request('PUT','/api/boards/{board}/title', array(
'headers' => $headers,
'json' => $request_body,
)
);
print_r($response->getBody()->getContents());
}
catch (\GuzzleHttp\Exception\BadResponseException $e) {
// handle exception or api errors.
print_r($e->getMessage());
}
// ...
PUT /api/boards/{board}/title
Update the title of a board
Body parameter
title: string
Parameters
Name | In | Type | Required | Description |
---|---|---|---|---|
board | path | string | true | the ID of the board to update |
body | body | object | true | none |
» title | body | string | true | the new title for the board |
Detailed descriptions
board: the ID of the board to update
Responses
Status | Meaning | Description | Schema |
---|---|---|---|
200 | OK | 200 response | None |
get_boards_count
Code samples
# You can also use wget
curl -X GET /api/boards_count \
-H 'Accept: application/json' \
-H 'Authorization: API_KEY'
GET /api/boards_count HTTP/1.1
Accept: application/json
const headers = {
'Accept':'application/json',
'Authorization':'API_KEY'
};
fetch('/api/boards_count',
{
method: 'GET',
headers: headers
})
.then(function(res) {
return res.json();
}).then(function(body) {
console.log(body);
});
const fetch = require('node-fetch');
const headers = {
'Accept':'application/json',
'Authorization':'API_KEY'
};
fetch('/api/boards_count',
{
method: 'GET',
headers: headers
})
.then(function(res) {
return res.json();
}).then(function(body) {
console.log(body);
});
require 'rest-client'
require 'json'
headers = {
'Accept' => 'application/json',
'Authorization' => 'API_KEY'
}
result = RestClient.get '/api/boards_count',
params: {
}, headers: headers
p JSON.parse(result)
import requests
headers = {
'Accept': 'application/json',
'Authorization': 'API_KEY'
}
r = requests.get('/api/boards_count', headers = headers)
print(r.json())
URL obj = new URL("/api/boards_count");
HttpURLConnection con = (HttpURLConnection) obj.openConnection();
con.setRequestMethod("GET");
int responseCode = con.getResponseCode();
BufferedReader in = new BufferedReader(
new InputStreamReader(con.getInputStream()));
String inputLine;
StringBuffer response = new StringBuffer();
while ((inputLine = in.readLine()) != null) {
response.append(inputLine);
}
in.close();
System.out.println(response.toString());
package main
import (
"bytes"
"net/http"
)
func main() {
headers := map[string][]string{
"Accept": []string{"application/json"},
"Authorization": []string{"API_KEY"},
}
data := bytes.NewBuffer([]byte{jsonReq})
req, err := http.NewRequest("GET", "/api/boards_count", data)
req.Header = headers
client := &http.Client{}
resp, err := client.Do(req)
// ...
}
'application/json',
'Authorization' => 'API_KEY',
);
$client = new \GuzzleHttp\Client();
// Define array of request body.
$request_body = array();
try {
$response = $client->request('GET','/api/boards_count', array(
'headers' => $headers,
'json' => $request_body,
)
);
print_r($response->getBody()->getContents());
}
catch (\GuzzleHttp\Exception\BadResponseException $e) {
// handle exception or api errors.
print_r($e->getMessage());
}
// ...
GET /api/boards_count
Get public and private boards count
Example responses
200 Response
{
"private": 0,
"public": 0
}
Responses
Status | Meaning | Description | Schema |
---|---|---|---|
200 | OK | 200 response | Inline |
Response Schema
Status Code 200
Name | Type | Required | Restrictions | Description |
---|---|---|---|---|
» private | integer | false | none | none |
» public | integer | false | none | none |
get_boards_from_user
Code samples
# You can also use wget
curl -X GET /api/users/{user}/boards \
-H 'Accept: application/json' \
-H 'Authorization: API_KEY'
GET /api/users/{user}/boards HTTP/1.1
Accept: application/json
const headers = {
'Accept':'application/json',
'Authorization':'API_KEY'
};
fetch('/api/users/{user}/boards',
{
method: 'GET',
headers: headers
})
.then(function(res) {
return res.json();
}).then(function(body) {
console.log(body);
});
const fetch = require('node-fetch');
const headers = {
'Accept':'application/json',
'Authorization':'API_KEY'
};
fetch('/api/users/{user}/boards',
{
method: 'GET',
headers: headers
})
.then(function(res) {
return res.json();
}).then(function(body) {
console.log(body);
});
require 'rest-client'
require 'json'
headers = {
'Accept' => 'application/json',
'Authorization' => 'API_KEY'
}
result = RestClient.get '/api/users/{user}/boards',
params: {
}, headers: headers
p JSON.parse(result)
import requests
headers = {
'Accept': 'application/json',
'Authorization': 'API_KEY'
}
r = requests.get('/api/users/{user}/boards', headers = headers)
print(r.json())
URL obj = new URL("/api/users/{user}/boards");
HttpURLConnection con = (HttpURLConnection) obj.openConnection();
con.setRequestMethod("GET");
int responseCode = con.getResponseCode();
BufferedReader in = new BufferedReader(
new InputStreamReader(con.getInputStream()));
String inputLine;
StringBuffer response = new StringBuffer();
while ((inputLine = in.readLine()) != null) {
response.append(inputLine);
}
in.close();
System.out.println(response.toString());
package main
import (
"bytes"
"net/http"
)
func main() {
headers := map[string][]string{
"Accept": []string{"application/json"},
"Authorization": []string{"API_KEY"},
}
data := bytes.NewBuffer([]byte{jsonReq})
req, err := http.NewRequest("GET", "/api/users/{user}/boards", data)
req.Header = headers
client := &http.Client{}
resp, err := client.Do(req)
// ...
}
'application/json',
'Authorization' => 'API_KEY',
);
$client = new \GuzzleHttp\Client();
// Define array of request body.
$request_body = array();
try {
$response = $client->request('GET','/api/users/{user}/boards', array(
'headers' => $headers,
'json' => $request_body,
)
);
print_r($response->getBody()->getContents());
}
catch (\GuzzleHttp\Exception\BadResponseException $e) {
// handle exception or api errors.
print_r($e->getMessage());
}
// ...
GET /api/users/{user}/boards
Get all boards attached to a user
Parameters
Name | In | Type | Required | Description |
---|---|---|---|---|
user | path | string | true | the ID of the user to retrieve the data |
Detailed descriptions
user: the ID of the user to retrieve the data
Example responses
200 Response
[
{
"_id": "string",
"title": "string"
}
]
Responses
Status | Meaning | Description | Schema |
---|---|---|---|
200 | OK | 200 response | Inline |
Response Schema
Status Code 200
Name | Type | Required | Restrictions | Description |
---|---|---|---|---|
» _id | string | false | none | none |
» title | string | false | none | none |
Checklists
get_all_checklists
Code samples
# You can also use wget
curl -X GET /api/boards/{board}/cards/{card}/checklists \
-H 'Accept: application/json' \
-H 'Authorization: API_KEY'
GET /api/boards/{board}/cards/{card}/checklists HTTP/1.1
Accept: application/json
const headers = {
'Accept':'application/json',
'Authorization':'API_KEY'
};
fetch('/api/boards/{board}/cards/{card}/checklists',
{
method: 'GET',
headers: headers
})
.then(function(res) {
return res.json();
}).then(function(body) {
console.log(body);
});
const fetch = require('node-fetch');
const headers = {
'Accept':'application/json',
'Authorization':'API_KEY'
};
fetch('/api/boards/{board}/cards/{card}/checklists',
{
method: 'GET',
headers: headers
})
.then(function(res) {
return res.json();
}).then(function(body) {
console.log(body);
});
require 'rest-client'
require 'json'
headers = {
'Accept' => 'application/json',
'Authorization' => 'API_KEY'
}
result = RestClient.get '/api/boards/{board}/cards/{card}/checklists',
params: {
}, headers: headers
p JSON.parse(result)
import requests
headers = {
'Accept': 'application/json',
'Authorization': 'API_KEY'
}
r = requests.get('/api/boards/{board}/cards/{card}/checklists', headers = headers)
print(r.json())
URL obj = new URL("/api/boards/{board}/cards/{card}/checklists");
HttpURLConnection con = (HttpURLConnection) obj.openConnection();
con.setRequestMethod("GET");
int responseCode = con.getResponseCode();
BufferedReader in = new BufferedReader(
new InputStreamReader(con.getInputStream()));
String inputLine;
StringBuffer response = new StringBuffer();
while ((inputLine = in.readLine()) != null) {
response.append(inputLine);
}
in.close();
System.out.println(response.toString());
package main
import (
"bytes"
"net/http"
)
func main() {
headers := map[string][]string{
"Accept": []string{"application/json"},
"Authorization": []string{"API_KEY"},
}
data := bytes.NewBuffer([]byte{jsonReq})
req, err := http.NewRequest("GET", "/api/boards/{board}/cards/{card}/checklists", data)
req.Header = headers
client := &http.Client{}
resp, err := client.Do(req)
// ...
}
'application/json',
'Authorization' => 'API_KEY',
);
$client = new \GuzzleHttp\Client();
// Define array of request body.
$request_body = array();
try {
$response = $client->request('GET','/api/boards/{board}/cards/{card}/checklists', array(
'headers' => $headers,
'json' => $request_body,
)
);
print_r($response->getBody()->getContents());
}
catch (\GuzzleHttp\Exception\BadResponseException $e) {
// handle exception or api errors.
print_r($e->getMessage());
}
// ...
GET /api/boards/{board}/cards/{card}/checklists
Get the list of checklists attached to a card
Parameters
Name | In | Type | Required | Description |
---|---|---|---|---|
board | path | string | true | the board ID |
card | path | string | true | the card ID |
Detailed descriptions
board: the board ID
card: the card ID
Example responses
200 Response
[
{
"_id": "string",
"title": "string"
}
]
Responses
Status | Meaning | Description | Schema |
---|---|---|---|
200 | OK | 200 response | Inline |
Response Schema
Status Code 200
Name | Type | Required | Restrictions | Description |
---|---|---|---|---|
» _id | string | false | none | none |
» title | string | false | none | none |
new_checklist
Code samples
# You can also use wget
curl -X POST /api/boards/{board}/cards/{card}/checklists \
-H 'Content-Type: multipart/form-data' \
-H 'Accept: application/json' \
-H 'Authorization: API_KEY'
POST /api/boards/{board}/cards/{card}/checklists HTTP/1.1
Content-Type: multipart/form-data
Accept: application/json
const inputBody = '{
"title": "string",
"items": "string"
}';
const headers = {
'Content-Type':'multipart/form-data',
'Accept':'application/json',
'Authorization':'API_KEY'
};
fetch('/api/boards/{board}/cards/{card}/checklists',
{
method: 'POST',
body: inputBody,
headers: headers
})
.then(function(res) {
return res.json();
}).then(function(body) {
console.log(body);
});
const fetch = require('node-fetch');
const inputBody = {
"title": "string",
"items": "string"
};
const headers = {
'Content-Type':'multipart/form-data',
'Accept':'application/json',
'Authorization':'API_KEY'
};
fetch('/api/boards/{board}/cards/{card}/checklists',
{
method: 'POST',
body: JSON.stringify(inputBody),
headers: headers
})
.then(function(res) {
return res.json();
}).then(function(body) {
console.log(body);
});
require 'rest-client'
require 'json'
headers = {
'Content-Type' => 'multipart/form-data',
'Accept' => 'application/json',
'Authorization' => 'API_KEY'
}
result = RestClient.post '/api/boards/{board}/cards/{card}/checklists',
params: {
}, headers: headers
p JSON.parse(result)
import requests
headers = {
'Content-Type': 'multipart/form-data',
'Accept': 'application/json',
'Authorization': 'API_KEY'
}
r = requests.post('/api/boards/{board}/cards/{card}/checklists', headers = headers)
print(r.json())
URL obj = new URL("/api/boards/{board}/cards/{card}/checklists");
HttpURLConnection con = (HttpURLConnection) obj.openConnection();
con.setRequestMethod("POST");
int responseCode = con.getResponseCode();
BufferedReader in = new BufferedReader(
new InputStreamReader(con.getInputStream()));
String inputLine;
StringBuffer response = new StringBuffer();
while ((inputLine = in.readLine()) != null) {
response.append(inputLine);
}
in.close();
System.out.println(response.toString());
package main
import (
"bytes"
"net/http"
)
func main() {
headers := map[string][]string{
"Content-Type": []string{"multipart/form-data"},
"Accept": []string{"application/json"},
"Authorization": []string{"API_KEY"},
}
data := bytes.NewBuffer([]byte{jsonReq})
req, err := http.NewRequest("POST", "/api/boards/{board}/cards/{card}/checklists", data)
req.Header = headers
client := &http.Client{}
resp, err := client.Do(req)
// ...
}
'multipart/form-data',
'Accept' => 'application/json',
'Authorization' => 'API_KEY',
);
$client = new \GuzzleHttp\Client();
// Define array of request body.
$request_body = array();
try {
$response = $client->request('POST','/api/boards/{board}/cards/{card}/checklists', array(
'headers' => $headers,
'json' => $request_body,
)
);
print_r($response->getBody()->getContents());
}
catch (\GuzzleHttp\Exception\BadResponseException $e) {
// handle exception or api errors.
print_r($e->getMessage());
}
// ...
POST /api/boards/{board}/cards/{card}/checklists
create a new checklist
Body parameter
title: string
items: string
Parameters
Name | In | Type | Required | Description |
---|---|---|---|---|
board | path | string | true | the board ID |
card | path | string | true | the card ID |
body | body | object | true | none |
» title | body | string | true | the title of the new checklist |
» items | body | string | false | the list of items on the new checklist |
Detailed descriptions
board: the board ID
card: the card ID
Example responses
200 Response
{
"_id": "string"
}
Responses
Status | Meaning | Description | Schema |
---|---|---|---|
200 | OK | 200 response | Inline |
Response Schema
Status Code 200
Name | Type | Required | Restrictions | Description |
---|---|---|---|---|
» _id | string | false | none | none |
get_checklist
Code samples
# You can also use wget
curl -X GET /api/boards/{board}/cards/{card}/checklists/{checklist} \
-H 'Accept: application/json' \
-H 'Authorization: API_KEY'
GET /api/boards/{board}/cards/{card}/checklists/{checklist} HTTP/1.1
Accept: application/json
const headers = {
'Accept':'application/json',
'Authorization':'API_KEY'
};
fetch('/api/boards/{board}/cards/{card}/checklists/{checklist}',
{
method: 'GET',
headers: headers
})
.then(function(res) {
return res.json();
}).then(function(body) {
console.log(body);
});
const fetch = require('node-fetch');
const headers = {
'Accept':'application/json',
'Authorization':'API_KEY'
};
fetch('/api/boards/{board}/cards/{card}/checklists/{checklist}',
{
method: 'GET',
headers: headers
})
.then(function(res) {
return res.json();
}).then(function(body) {
console.log(body);
});
require 'rest-client'
require 'json'
headers = {
'Accept' => 'application/json',
'Authorization' => 'API_KEY'
}
result = RestClient.get '/api/boards/{board}/cards/{card}/checklists/{checklist}',
params: {
}, headers: headers
p JSON.parse(result)
import requests
headers = {
'Accept': 'application/json',
'Authorization': 'API_KEY'
}
r = requests.get('/api/boards/{board}/cards/{card}/checklists/{checklist}', headers = headers)
print(r.json())
URL obj = new URL("/api/boards/{board}/cards/{card}/checklists/{checklist}");
HttpURLConnection con = (HttpURLConnection) obj.openConnection();
con.setRequestMethod("GET");
int responseCode = con.getResponseCode();
BufferedReader in = new BufferedReader(
new InputStreamReader(con.getInputStream()));
String inputLine;
StringBuffer response = new StringBuffer();
while ((inputLine = in.readLine()) != null) {
response.append(inputLine);
}
in.close();
System.out.println(response.toString());
package main
import (
"bytes"
"net/http"
)
func main() {
headers := map[string][]string{
"Accept": []string{"application/json"},
"Authorization": []string{"API_KEY"},
}
data := bytes.NewBuffer([]byte{jsonReq})
req, err := http.NewRequest("GET", "/api/boards/{board}/cards/{card}/checklists/{checklist}", data)
req.Header = headers
client := &http.Client{}
resp, err := client.Do(req)
// ...
}
'application/json',
'Authorization' => 'API_KEY',
);
$client = new \GuzzleHttp\Client();
// Define array of request body.
$request_body = array();
try {
$response = $client->request('GET','/api/boards/{board}/cards/{card}/checklists/{checklist}', array(
'headers' => $headers,
'json' => $request_body,
)
);
print_r($response->getBody()->getContents());
}
catch (\GuzzleHttp\Exception\BadResponseException $e) {
// handle exception or api errors.
print_r($e->getMessage());
}
// ...
GET /api/boards/{board}/cards/{card}/checklists/{checklist}
Get a checklist
Parameters
Name | In | Type | Required | Description |
---|---|---|---|---|
board | path | string | true | the board ID |
card | path | string | true | the card ID |
checklist | path | string | true | the ID of the checklist |
Detailed descriptions
board: the board ID
card: the card ID
checklist: the ID of the checklist
Example responses
200 Response
{
"cardId": "string",
"title": "string",
"finishedAt": "string",
"createdAt": "string",
"sort": 0,
"items": [
{
"_id": "string",
"title": "string",
"isFinished": true
}
]
}
Responses
Status | Meaning | Description | Schema |
---|---|---|---|
200 | OK | 200 response | Inline |
Response Schema
Status Code 200
Name | Type | Required | Restrictions | Description |
---|---|---|---|---|
» cardId | string | false | none | none |
» title | string | false | none | none |
» finishedAt | string | false | none | none |
» createdAt | string | false | none | none |
» sort | number | false | none | none |
» items | [object] | false | none | none |
»» _id | string | false | none | none |
»» title | string | false | none | none |
»» isFinished | boolean | false | none | none |
delete_checklist
Code samples
# You can also use wget
curl -X DELETE /api/boards/{board}/cards/{card}/checklists/{checklist} \
-H 'Accept: application/json' \
-H 'Authorization: API_KEY'
DELETE /api/boards/{board}/cards/{card}/checklists/{checklist} HTTP/1.1
Accept: application/json
const headers = {
'Accept':'application/json',
'Authorization':'API_KEY'
};
fetch('/api/boards/{board}/cards/{card}/checklists/{checklist}',
{
method: 'DELETE',
headers: headers
})
.then(function(res) {
return res.json();
}).then(function(body) {
console.log(body);
});
const fetch = require('node-fetch');
const headers = {
'Accept':'application/json',
'Authorization':'API_KEY'
};
fetch('/api/boards/{board}/cards/{card}/checklists/{checklist}',
{
method: 'DELETE',
headers: headers
})
.then(function(res) {
return res.json();
}).then(function(body) {
console.log(body);
});
require 'rest-client'
require 'json'
headers = {
'Accept' => 'application/json',
'Authorization' => 'API_KEY'
}
result = RestClient.delete '/api/boards/{board}/cards/{card}/checklists/{checklist}',
params: {
}, headers: headers
p JSON.parse(result)
import requests
headers = {
'Accept': 'application/json',
'Authorization': 'API_KEY'
}
r = requests.delete('/api/boards/{board}/cards/{card}/checklists/{checklist}', headers = headers)
print(r.json())
URL obj = new URL("/api/boards/{board}/cards/{card}/checklists/{checklist}");
HttpURLConnection con = (HttpURLConnection) obj.openConnection();
con.setRequestMethod("DELETE");
int responseCode = con.getResponseCode();
BufferedReader in = new BufferedReader(
new InputStreamReader(con.getInputStream()));
String inputLine;
StringBuffer response = new StringBuffer();
while ((inputLine = in.readLine()) != null) {
response.append(inputLine);
}
in.close();
System.out.println(response.toString());
package main
import (
"bytes"
"net/http"
)
func main() {
headers := map[string][]string{
"Accept": []string{"application/json"},
"Authorization": []string{"API_KEY"},
}
data := bytes.NewBuffer([]byte{jsonReq})
req, err := http.NewRequest("DELETE", "/api/boards/{board}/cards/{card}/checklists/{checklist}", data)
req.Header = headers
client := &http.Client{}
resp, err := client.Do(req)
// ...
}
'application/json',
'Authorization' => 'API_KEY',
);
$client = new \GuzzleHttp\Client();
// Define array of request body.
$request_body = array();
try {
$response = $client->request('DELETE','/api/boards/{board}/cards/{card}/checklists/{checklist}', array(
'headers' => $headers,
'json' => $request_body,
)
);
print_r($response->getBody()->getContents());
}
catch (\GuzzleHttp\Exception\BadResponseException $e) {
// handle exception or api errors.
print_r($e->getMessage());
}
// ...
DELETE /api/boards/{board}/cards/{card}/checklists/{checklist}
Delete a checklist
The checklist will be removed, not put in the recycle bin.
Parameters
Name | In | Type | Required | Description |
---|---|---|---|---|
board | path | string | true | the board ID |
card | path | string | true | the card ID |
checklist | path | string | true | the ID of the checklist to remove |
Detailed descriptions
board: the board ID
card: the card ID
checklist: the ID of the checklist to remove
Example responses
200 Response
{
"_id": "string"
}
Responses
Status | Meaning | Description | Schema |
---|---|---|---|
200 | OK | 200 response | Inline |
Response Schema
Status Code 200
Name | Type | Required | Restrictions | Description |
---|---|---|---|---|
» _id | string | false | none | none |
ChecklistItems
new_checklist_item
Code samples
# You can also use wget
curl -X POST /api/boards/{board}/cards/{card}/checklists/{checklist}/items \
-H 'Content-Type: multipart/form-data' \
-H 'Accept: application/json' \
-H 'Authorization: API_KEY'
POST /api/boards/{board}/cards/{card}/checklists/{checklist}/items HTTP/1.1
Content-Type: multipart/form-data
Accept: application/json
const inputBody = '{
"title": "string"
}';
const headers = {
'Content-Type':'multipart/form-data',
'Accept':'application/json',
'Authorization':'API_KEY'
};
fetch('/api/boards/{board}/cards/{card}/checklists/{checklist}/items',
{
method: 'POST',
body: inputBody,
headers: headers
})
.then(function(res) {
return res.json();
}).then(function(body) {
console.log(body);
});
const fetch = require('node-fetch');
const inputBody = {
"title": "string"
};
const headers = {
'Content-Type':'multipart/form-data',
'Accept':'application/json',
'Authorization':'API_KEY'
};
fetch('/api/boards/{board}/cards/{card}/checklists/{checklist}/items',
{
method: 'POST',
body: JSON.stringify(inputBody),
headers: headers
})
.then(function(res) {
return res.json();
}).then(function(body) {
console.log(body);
});
require 'rest-client'
require 'json'
headers = {
'Content-Type' => 'multipart/form-data',
'Accept' => 'application/json',
'Authorization' => 'API_KEY'
}
result = RestClient.post '/api/boards/{board}/cards/{card}/checklists/{checklist}/items',
params: {
}, headers: headers
p JSON.parse(result)
import requests
headers = {
'Content-Type': 'multipart/form-data',
'Accept': 'application/json',
'Authorization': 'API_KEY'
}
r = requests.post('/api/boards/{board}/cards/{card}/checklists/{checklist}/items', headers = headers)
print(r.json())
URL obj = new URL("/api/boards/{board}/cards/{card}/checklists/{checklist}/items");
HttpURLConnection con = (HttpURLConnection) obj.openConnection();
con.setRequestMethod("POST");
int responseCode = con.getResponseCode();
BufferedReader in = new BufferedReader(
new InputStreamReader(con.getInputStream()));
String inputLine;
StringBuffer response = new StringBuffer();
while ((inputLine = in.readLine()) != null) {
response.append(inputLine);
}
in.close();
System.out.println(response.toString());
package main
import (
"bytes"
"net/http"
)
func main() {
headers := map[string][]string{
"Content-Type": []string{"multipart/form-data"},
"Accept": []string{"application/json"},
"Authorization": []string{"API_KEY"},
}
data := bytes.NewBuffer([]byte{jsonReq})
req, err := http.NewRequest("POST", "/api/boards/{board}/cards/{card}/checklists/{checklist}/items", data)
req.Header = headers
client := &http.Client{}
resp, err := client.Do(req)
// ...
}
'multipart/form-data',
'Accept' => 'application/json',
'Authorization' => 'API_KEY',
);
$client = new \GuzzleHttp\Client();
// Define array of request body.
$request_body = array();
try {
$response = $client->request('POST','/api/boards/{board}/cards/{card}/checklists/{checklist}/items', array(
'headers' => $headers,
'json' => $request_body,
)
);
print_r($response->getBody()->getContents());
}
catch (\GuzzleHttp\Exception\BadResponseException $e) {
// handle exception or api errors.
print_r($e->getMessage());
}
// ...
POST /api/boards/{board}/cards/{card}/checklists/{checklist}/items
add a new item to a checklist
Body parameter
title: string
Parameters
Name | In | Type | Required | Description |
---|---|---|---|---|
board | path | string | true | the board ID |
card | path | string | true | the card ID |
checklist | path | string | true | the ID of the checklist |
body | body | object | true | none |
» title | body | string | true | the title of the new item |
Detailed descriptions
board: the board ID
card: the card ID
checklist: the ID of the checklist
Example responses
200 Response
{
"_id": "string"
}
Responses
Status | Meaning | Description | Schema |
---|---|---|---|
200 | OK | 200 response | Inline |
Response Schema
Status Code 200
Name | Type | Required | Restrictions | Description |
---|---|---|---|---|
» _id | string | false | none | none |
get_checklist_item
Code samples
# You can also use wget
curl -X GET /api/boards/{board}/cards/{card}/checklists/{checklist}/items/{item} \
-H 'Accept: application/json' \
-H 'Authorization: API_KEY'
GET /api/boards/{board}/cards/{card}/checklists/{checklist}/items/{item} HTTP/1.1
Accept: application/json
const headers = {
'Accept':'application/json',
'Authorization':'API_KEY'
};
fetch('/api/boards/{board}/cards/{card}/checklists/{checklist}/items/{item}',
{
method: 'GET',
headers: headers
})
.then(function(res) {
return res.json();
}).then(function(body) {
console.log(body);
});
const fetch = require('node-fetch');
const headers = {
'Accept':'application/json',
'Authorization':'API_KEY'
};
fetch('/api/boards/{board}/cards/{card}/checklists/{checklist}/items/{item}',
{
method: 'GET',
headers: headers
})
.then(function(res) {
return res.json();
}).then(function(body) {
console.log(body);
});
require 'rest-client'
require 'json'
headers = {
'Accept' => 'application/json',
'Authorization' => 'API_KEY'
}
result = RestClient.get '/api/boards/{board}/cards/{card}/checklists/{checklist}/items/{item}',
params: {
}, headers: headers
p JSON.parse(result)
import requests
headers = {
'Accept': 'application/json',
'Authorization': 'API_KEY'
}
r = requests.get('/api/boards/{board}/cards/{card}/checklists/{checklist}/items/{item}', headers = headers)
print(r.json())
URL obj = new URL("/api/boards/{board}/cards/{card}/checklists/{checklist}/items/{item}");
HttpURLConnection con = (HttpURLConnection) obj.openConnection();
con.setRequestMethod("GET");
int responseCode = con.getResponseCode();
BufferedReader in = new BufferedReader(
new InputStreamReader(con.getInputStream()));
String inputLine;
StringBuffer response = new StringBuffer();
while ((inputLine = in.readLine()) != null) {
response.append(inputLine);
}
in.close();
System.out.println(response.toString());
package main
import (
"bytes"
"net/http"
)
func main() {
headers := map[string][]string{
"Accept": []string{"application/json"},
"Authorization": []string{"API_KEY"},
}
data := bytes.NewBuffer([]byte{jsonReq})
req, err := http.NewRequest("GET", "/api/boards/{board}/cards/{card}/checklists/{checklist}/items/{item}", data)
req.Header = headers
client := &http.Client{}
resp, err := client.Do(req)
// ...
}
'application/json',
'Authorization' => 'API_KEY',
);
$client = new \GuzzleHttp\Client();
// Define array of request body.
$request_body = array();
try {
$response = $client->request('GET','/api/boards/{board}/cards/{card}/checklists/{checklist}/items/{item}', array(
'headers' => $headers,
'json' => $request_body,
)
);
print_r($response->getBody()->getContents());
}
catch (\GuzzleHttp\Exception\BadResponseException $e) {
// handle exception or api errors.
print_r($e->getMessage());
}
// ...
GET /api/boards/{board}/cards/{card}/checklists/{checklist}/items/{item}
Get a checklist item
Parameters
Name | In | Type | Required | Description |
---|---|---|---|---|
board | path | string | true | the board ID |
card | path | string | true | the card ID |
checklist | path | string | true | the checklist ID |
item | path | string | true | the ID of the item |
Detailed descriptions
board: the board ID
card: the card ID
checklist: the checklist ID
item: the ID of the item
Example responses
200 Response
{
"title": "string",
"sort": 0,
"isFinished": true,
"checklistId": "string",
"cardId": "string",
"createdAt": "string",
"modifiedAt": "string"
}
Responses
Status | Meaning | Description | Schema |
---|---|---|---|
200 | OK | 200 response | ChecklistItems |
edit_checklist_item
Code samples
# You can also use wget
curl -X PUT /api/boards/{board}/cards/{card}/checklists/{checklist}/items/{item} \
-H 'Content-Type: multipart/form-data' \
-H 'Accept: application/json' \
-H 'Authorization: API_KEY'
PUT /api/boards/{board}/cards/{card}/checklists/{checklist}/items/{item} HTTP/1.1
Content-Type: multipart/form-data
Accept: application/json
const inputBody = '{
"isFinished": "string",
"title": "string"
}';
const headers = {
'Content-Type':'multipart/form-data',
'Accept':'application/json',
'Authorization':'API_KEY'
};
fetch('/api/boards/{board}/cards/{card}/checklists/{checklist}/items/{item}',
{
method: 'PUT',
body: inputBody,
headers: headers
})
.then(function(res) {
return res.json();
}).then(function(body) {
console.log(body);
});
const fetch = require('node-fetch');
const inputBody = {
"isFinished": "string",
"title": "string"
};
const headers = {
'Content-Type':'multipart/form-data',
'Accept':'application/json',
'Authorization':'API_KEY'
};
fetch('/api/boards/{board}/cards/{card}/checklists/{checklist}/items/{item}',
{
method: 'PUT',
body: JSON.stringify(inputBody),
headers: headers
})
.then(function(res) {
return res.json();
}).then(function(body) {
console.log(body);
});
require 'rest-client'
require 'json'
headers = {
'Content-Type' => 'multipart/form-data',
'Accept' => 'application/json',
'Authorization' => 'API_KEY'
}
result = RestClient.put '/api/boards/{board}/cards/{card}/checklists/{checklist}/items/{item}',
params: {
}, headers: headers
p JSON.parse(result)
import requests
headers = {
'Content-Type': 'multipart/form-data',
'Accept': 'application/json',
'Authorization': 'API_KEY'
}
r = requests.put('/api/boards/{board}/cards/{card}/checklists/{checklist}/items/{item}', headers = headers)
print(r.json())
URL obj = new URL("/api/boards/{board}/cards/{card}/checklists/{checklist}/items/{item}");
HttpURLConnection con = (HttpURLConnection) obj.openConnection();
con.setRequestMethod("PUT");
int responseCode = con.getResponseCode();
BufferedReader in = new BufferedReader(
new InputStreamReader(con.getInputStream()));
String inputLine;
StringBuffer response = new StringBuffer();
while ((inputLine = in.readLine()) != null) {
response.append(inputLine);
}
in.close();
System.out.println(response.toString());
package main
import (
"bytes"
"net/http"
)
func main() {
headers := map[string][]string{
"Content-Type": []string{"multipart/form-data"},
"Accept": []string{"application/json"},
"Authorization": []string{"API_KEY"},
}
data := bytes.NewBuffer([]byte{jsonReq})
req, err := http.NewRequest("PUT", "/api/boards/{board}/cards/{card}/checklists/{checklist}/items/{item}", data)
req.Header = headers
client := &http.Client{}
resp, err := client.Do(req)
// ...
}
'multipart/form-data',
'Accept' => 'application/json',
'Authorization' => 'API_KEY',
);
$client = new \GuzzleHttp\Client();
// Define array of request body.
$request_body = array();
try {
$response = $client->request('PUT','/api/boards/{board}/cards/{card}/checklists/{checklist}/items/{item}', array(
'headers' => $headers,
'json' => $request_body,
)
);
print_r($response->getBody()->getContents());
}
catch (\GuzzleHttp\Exception\BadResponseException $e) {
// handle exception or api errors.
print_r($e->getMessage());
}
// ...
PUT /api/boards/{board}/cards/{card}/checklists/{checklist}/items/{item}
Edit a checklist item
Body parameter
isFinished: string
title: string
Parameters
Name | In | Type | Required | Description |
---|---|---|---|---|
board | path | string | true | the board ID |
card | path | string | true | the card ID |
checklist | path | string | true | the checklist ID |
item | path | string | true | the ID of the item |
body | body | object | false | none |
» isFinished | body | string | false | is the item checked? |
» title | body | string | false | the new text of the item |
Detailed descriptions
board: the board ID
card: the card ID
checklist: the checklist ID
item: the ID of the item
Example responses
200 Response
{
"_id": "string"
}
Responses
Status | Meaning | Description | Schema |
---|---|---|---|
200 | OK | 200 response | Inline |
Response Schema
Status Code 200
Name | Type | Required | Restrictions | Description |
---|---|---|---|---|
» _id | string | false | none | none |
delete_checklist_item
Code samples
# You can also use wget
curl -X DELETE /api/boards/{board}/cards/{card}/checklists/{checklist}/items/{item} \
-H 'Accept: application/json' \
-H 'Authorization: API_KEY'
DELETE /api/boards/{board}/cards/{card}/checklists/{checklist}/items/{item} HTTP/1.1
Accept: application/json
const headers = {
'Accept':'application/json',
'Authorization':'API_KEY'
};
fetch('/api/boards/{board}/cards/{card}/checklists/{checklist}/items/{item}',
{
method: 'DELETE',
headers: headers
})
.then(function(res) {
return res.json();
}).then(function(body) {
console.log(body);
});
const fetch = require('node-fetch');
const headers = {
'Accept':'application/json',
'Authorization':'API_KEY'
};
fetch('/api/boards/{board}/cards/{card}/checklists/{checklist}/items/{item}',
{
method: 'DELETE',
headers: headers
})
.then(function(res) {
return res.json();
}).then(function(body) {
console.log(body);
});
require 'rest-client'
require 'json'
headers = {
'Accept' => 'application/json',
'Authorization' => 'API_KEY'
}
result = RestClient.delete '/api/boards/{board}/cards/{card}/checklists/{checklist}/items/{item}',
params: {
}, headers: headers
p JSON.parse(result)
import requests
headers = {
'Accept': 'application/json',
'Authorization': 'API_KEY'
}
r = requests.delete('/api/boards/{board}/cards/{card}/checklists/{checklist}/items/{item}', headers = headers)
print(r.json())
URL obj = new URL("/api/boards/{board}/cards/{card}/checklists/{checklist}/items/{item}");
HttpURLConnection con = (HttpURLConnection) obj.openConnection();
con.setRequestMethod("DELETE");
int responseCode = con.getResponseCode();
BufferedReader in = new BufferedReader(
new InputStreamReader(con.getInputStream()));
String inputLine;
StringBuffer response = new StringBuffer();
while ((inputLine = in.readLine()) != null) {
response.append(inputLine);
}
in.close();
System.out.println(response.toString());
package main
import (
"bytes"
"net/http"
)
func main() {
headers := map[string][]string{
"Accept": []string{"application/json"},
"Authorization": []string{"API_KEY"},
}
data := bytes.NewBuffer([]byte{jsonReq})
req, err := http.NewRequest("DELETE", "/api/boards/{board}/cards/{card}/checklists/{checklist}/items/{item}", data)
req.Header = headers
client := &http.Client{}
resp, err := client.Do(req)
// ...
}
'application/json',
'Authorization' => 'API_KEY',
);
$client = new \GuzzleHttp\Client();
// Define array of request body.
$request_body = array();
try {
$response = $client->request('DELETE','/api/boards/{board}/cards/{card}/checklists/{checklist}/items/{item}', array(
'headers' => $headers,
'json' => $request_body,
)
);
print_r($response->getBody()->getContents());
}
catch (\GuzzleHttp\Exception\BadResponseException $e) {
// handle exception or api errors.
print_r($e->getMessage());
}
// ...
DELETE /api/boards/{board}/cards/{card}/checklists/{checklist}/items/{item}
Delete a checklist item
Note: this operation can't be reverted.
Parameters
Name | In | Type | Required | Description |
---|---|---|---|---|
board | path | string | true | the board ID |
card | path | string | true | the card ID |
checklist | path | string | true | the checklist ID |
item | path | string | true | the ID of the item to be removed |
Detailed descriptions
board: the board ID
card: the card ID
checklist: the checklist ID
item: the ID of the item to be removed
Example responses
200 Response
{
"_id": "string"
}
Responses
Status | Meaning | Description | Schema |
---|---|---|---|
200 | OK | 200 response | Inline |
Response Schema
Status Code 200
Name | Type | Required | Restrictions | Description |
---|---|---|---|---|
» _id | string | false | none | none |
CardComments
get_all_comments
Code samples
# You can also use wget
curl -X GET /api/boards/{board}/cards/{card}/comments \
-H 'Accept: application/json' \
-H 'Authorization: API_KEY'
GET /api/boards/{board}/cards/{card}/comments HTTP/1.1
Accept: application/json
const headers = {
'Accept':'application/json',
'Authorization':'API_KEY'
};
fetch('/api/boards/{board}/cards/{card}/comments',
{
method: 'GET',
headers: headers
})
.then(function(res) {
return res.json();
}).then(function(body) {
console.log(body);
});
const fetch = require('node-fetch');
const headers = {
'Accept':'application/json',
'Authorization':'API_KEY'
};
fetch('/api/boards/{board}/cards/{card}/comments',
{
method: 'GET',
headers: headers
})
.then(function(res) {
return res.json();
}).then(function(body) {
console.log(body);
});
require 'rest-client'
require 'json'
headers = {
'Accept' => 'application/json',
'Authorization' => 'API_KEY'
}
result = RestClient.get '/api/boards/{board}/cards/{card}/comments',
params: {
}, headers: headers
p JSON.parse(result)
import requests
headers = {
'Accept': 'application/json',
'Authorization': 'API_KEY'
}
r = requests.get('/api/boards/{board}/cards/{card}/comments', headers = headers)
print(r.json())
URL obj = new URL("/api/boards/{board}/cards/{card}/comments");
HttpURLConnection con = (HttpURLConnection) obj.openConnection();
con.setRequestMethod("GET");
int responseCode = con.getResponseCode();
BufferedReader in = new BufferedReader(
new InputStreamReader(con.getInputStream()));
String inputLine;
StringBuffer response = new StringBuffer();
while ((inputLine = in.readLine()) != null) {
response.append(inputLine);
}
in.close();
System.out.println(response.toString());
package main
import (
"bytes"
"net/http"
)
func main() {
headers := map[string][]string{
"Accept": []string{"application/json"},
"Authorization": []string{"API_KEY"},
}
data := bytes.NewBuffer([]byte{jsonReq})
req, err := http.NewRequest("GET", "/api/boards/{board}/cards/{card}/comments", data)
req.Header = headers
client := &http.Client{}
resp, err := client.Do(req)
// ...
}
'application/json',
'Authorization' => 'API_KEY',
);
$client = new \GuzzleHttp\Client();
// Define array of request body.
$request_body = array();
try {
$response = $client->request('GET','/api/boards/{board}/cards/{card}/comments', array(
'headers' => $headers,
'json' => $request_body,
)
);
print_r($response->getBody()->getContents());
}
catch (\GuzzleHttp\Exception\BadResponseException $e) {
// handle exception or api errors.
print_r($e->getMessage());
}
// ...
GET /api/boards/{board}/cards/{card}/comments
Get all comments attached to a card
Parameters
Name | In | Type | Required | Description |
---|---|---|---|---|
board | path | string | true | the board ID of the card |
card | path | string | true | the ID of the card |
Detailed descriptions
board: the board ID of the card
card: the ID of the card
Example responses
200 Response
[
{
"_id": "string",
"comment": "string",
"authorId": "string"
}
]
Responses
Status | Meaning | Description | Schema |
---|---|---|---|
200 | OK | 200 response | Inline |
Response Schema
Status Code 200
Name | Type | Required | Restrictions | Description |
---|---|---|---|---|
» _id | string | false | none | none |
» comment | string | false | none | none |
» authorId | string | false | none | none |
new_comment
Code samples
# You can also use wget
curl -X POST /api/boards/{board}/cards/{card}/comments \
-H 'Content-Type: multipart/form-data' \
-H 'Accept: application/json' \
-H 'Authorization: API_KEY'
POST /api/boards/{board}/cards/{card}/comments HTTP/1.1
Content-Type: multipart/form-data
Accept: application/json
const inputBody = '{
"authorId": "string",
"comment": "string"
}';
const headers = {
'Content-Type':'multipart/form-data',
'Accept':'application/json',
'Authorization':'API_KEY'
};
fetch('/api/boards/{board}/cards/{card}/comments',
{
method: 'POST',
body: inputBody,
headers: headers
})
.then(function(res) {
return res.json();
}).then(function(body) {
console.log(body);
});
const fetch = require('node-fetch');
const inputBody = {
"authorId": "string",
"comment": "string"
};
const headers = {
'Content-Type':'multipart/form-data',
'Accept':'application/json',
'Authorization':'API_KEY'
};
fetch('/api/boards/{board}/cards/{card}/comments',
{
method: 'POST',
body: JSON.stringify(inputBody),
headers: headers
})
.then(function(res) {
return res.json();
}).then(function(body) {
console.log(body);
});
require 'rest-client'
require 'json'
headers = {
'Content-Type' => 'multipart/form-data',
'Accept' => 'application/json',
'Authorization' => 'API_KEY'
}
result = RestClient.post '/api/boards/{board}/cards/{card}/comments',
params: {
}, headers: headers
p JSON.parse(result)
import requests
headers = {
'Content-Type': 'multipart/form-data',
'Accept': 'application/json',
'Authorization': 'API_KEY'
}
r = requests.post('/api/boards/{board}/cards/{card}/comments', headers = headers)
print(r.json())
URL obj = new URL("/api/boards/{board}/cards/{card}/comments");
HttpURLConnection con = (HttpURLConnection) obj.openConnection();
con.setRequestMethod("POST");
int responseCode = con.getResponseCode();
BufferedReader in = new BufferedReader(
new InputStreamReader(con.getInputStream()));
String inputLine;
StringBuffer response = new StringBuffer();
while ((inputLine = in.readLine()) != null) {
response.append(inputLine);
}
in.close();
System.out.println(response.toString());
package main
import (
"bytes"
"net/http"
)
func main() {
headers := map[string][]string{
"Content-Type": []string{"multipart/form-data"},
"Accept": []string{"application/json"},
"Authorization": []string{"API_KEY"},
}
data := bytes.NewBuffer([]byte{jsonReq})
req, err := http.NewRequest("POST", "/api/boards/{board}/cards/{card}/comments", data)
req.Header = headers
client := &http.Client{}
resp, err := client.Do(req)
// ...
}
'multipart/form-data',
'Accept' => 'application/json',
'Authorization' => 'API_KEY',
);
$client = new \GuzzleHttp\Client();
// Define array of request body.
$request_body = array();
try {
$response = $client->request('POST','/api/boards/{board}/cards/{card}/comments', array(
'headers' => $headers,
'json' => $request_body,
)
);
print_r($response->getBody()->getContents());
}
catch (\GuzzleHttp\Exception\BadResponseException $e) {
// handle exception or api errors.
print_r($e->getMessage());
}
// ...
POST /api/boards/{board}/cards/{card}/comments
Add a comment on a card
Body parameter
authorId: string
comment: string
Parameters
Name | In | Type | Required | Description |
---|---|---|---|---|
board | path | string | true | the board ID of the card |
card | path | string | true | the ID of the card |
body | body | object | true | none |
» authorId | body | string | true | the user who 'posted' the comment |
» comment | body | string | true | the comment value |
Detailed descriptions
board: the board ID of the card
card: the ID of the card
Example responses
200 Response
{
"_id": "string"
}
Responses
Status | Meaning | Description | Schema |
---|---|---|---|
200 | OK | 200 response | Inline |
Response Schema
Status Code 200
Name | Type | Required | Restrictions | Description |
---|---|---|---|---|
» _id | string | false | none | none |
get_comment
Code samples
# You can also use wget
curl -X GET /api/boards/{board}/cards/{card}/comments/{comment} \
-H 'Accept: application/json' \
-H 'Authorization: API_KEY'
GET /api/boards/{board}/cards/{card}/comments/{comment} HTTP/1.1
Accept: application/json
const headers = {
'Accept':'application/json',
'Authorization':'API_KEY'
};
fetch('/api/boards/{board}/cards/{card}/comments/{comment}',
{
method: 'GET',
headers: headers
})
.then(function(res) {
return res.json();
}).then(function(body) {
console.log(body);
});
const fetch = require('node-fetch');
const headers = {
'Accept':'application/json',
'Authorization':'API_KEY'
};
fetch('/api/boards/{board}/cards/{card}/comments/{comment}',
{
method: 'GET',
headers: headers
})
.then(function(res) {
return res.json();
}).then(function(body) {
console.log(body);
});
require 'rest-client'
require 'json'
headers = {
'Accept' => 'application/json',
'Authorization' => 'API_KEY'
}
result = RestClient.get '/api/boards/{board}/cards/{card}/comments/{comment}',
params: {
}, headers: headers
p JSON.parse(result)
import requests
headers = {
'Accept': 'application/json',
'Authorization': 'API_KEY'
}
r = requests.get('/api/boards/{board}/cards/{card}/comments/{comment}', headers = headers)
print(r.json())
URL obj = new URL("/api/boards/{board}/cards/{card}/comments/{comment}");
HttpURLConnection con = (HttpURLConnection) obj.openConnection();
con.setRequestMethod("GET");
int responseCode = con.getResponseCode();
BufferedReader in = new BufferedReader(
new InputStreamReader(con.getInputStream()));
String inputLine;
StringBuffer response = new StringBuffer();
while ((inputLine = in.readLine()) != null) {
response.append(inputLine);
}
in.close();
System.out.println(response.toString());
package main
import (
"bytes"
"net/http"
)
func main() {
headers := map[string][]string{
"Accept": []string{"application/json"},
"Authorization": []string{"API_KEY"},
}
data := bytes.NewBuffer([]byte{jsonReq})
req, err := http.NewRequest("GET", "/api/boards/{board}/cards/{card}/comments/{comment}", data)
req.Header = headers
client := &http.Client{}
resp, err := client.Do(req)
// ...
}
'application/json',
'Authorization' => 'API_KEY',
);
$client = new \GuzzleHttp\Client();
// Define array of request body.
$request_body = array();
try {
$response = $client->request('GET','/api/boards/{board}/cards/{card}/comments/{comment}', array(
'headers' => $headers,
'json' => $request_body,
)
);
print_r($response->getBody()->getContents());
}
catch (\GuzzleHttp\Exception\BadResponseException $e) {
// handle exception or api errors.
print_r($e->getMessage());
}
// ...
GET /api/boards/{board}/cards/{card}/comments/{comment}
Get a comment on a card
Parameters
Name | In | Type | Required | Description |
---|---|---|---|---|
board | path | string | true | the board ID of the card |
card | path | string | true | the ID of the card |
comment | path | string | true | the ID of the comment to retrieve |
Detailed descriptions
board: the board ID of the card
card: the ID of the card
comment: the ID of the comment to retrieve
Example responses
200 Response
{
"boardId": "string",
"cardId": "string",
"text": "string",
"createdAt": "string",
"modifiedAt": "string",
"userId": "string"
}
Responses
Status | Meaning | Description | Schema |
---|---|---|---|
200 | OK | 200 response | CardComments |
delete_comment
Code samples
# You can also use wget
curl -X DELETE /api/boards/{board}/cards/{card}/comments/{comment} \
-H 'Accept: application/json' \
-H 'Authorization: API_KEY'
DELETE /api/boards/{board}/cards/{card}/comments/{comment} HTTP/1.1
Accept: application/json
const headers = {
'Accept':'application/json',
'Authorization':'API_KEY'
};
fetch('/api/boards/{board}/cards/{card}/comments/{comment}',
{
method: 'DELETE',
headers: headers
})
.then(function(res) {
return res.json();
}).then(function(body) {
console.log(body);
});
const fetch = require('node-fetch');
const headers = {
'Accept':'application/json',
'Authorization':'API_KEY'
};
fetch('/api/boards/{board}/cards/{card}/comments/{comment}',
{
method: 'DELETE',
headers: headers
})
.then(function(res) {
return res.json();
}).then(function(body) {
console.log(body);
});
require 'rest-client'
require 'json'
headers = {
'Accept' => 'application/json',
'Authorization' => 'API_KEY'
}
result = RestClient.delete '/api/boards/{board}/cards/{card}/comments/{comment}',
params: {
}, headers: headers
p JSON.parse(result)
import requests
headers = {
'Accept': 'application/json',
'Authorization': 'API_KEY'
}
r = requests.delete('/api/boards/{board}/cards/{card}/comments/{comment}', headers = headers)
print(r.json())
URL obj = new URL("/api/boards/{board}/cards/{card}/comments/{comment}");
HttpURLConnection con = (HttpURLConnection) obj.openConnection();
con.setRequestMethod("DELETE");
int responseCode = con.getResponseCode();
BufferedReader in = new BufferedReader(
new InputStreamReader(con.getInputStream()));
String inputLine;
StringBuffer response = new StringBuffer();
while ((inputLine = in.readLine()) != null) {
response.append(inputLine);
}
in.close();
System.out.println(response.toString());
package main
import (
"bytes"
"net/http"
)
func main() {
headers := map[string][]string{
"Accept": []string{"application/json"},
"Authorization": []string{"API_KEY"},
}
data := bytes.NewBuffer([]byte{jsonReq})
req, err := http.NewRequest("DELETE", "/api/boards/{board}/cards/{card}/comments/{comment}", data)
req.Header = headers
client := &http.Client{}
resp, err := client.Do(req)
// ...
}
'application/json',
'Authorization' => 'API_KEY',
);
$client = new \GuzzleHttp\Client();
// Define array of request body.
$request_body = array();
try {
$response = $client->request('DELETE','/api/boards/{board}/cards/{card}/comments/{comment}', array(
'headers' => $headers,
'json' => $request_body,
)
);
print_r($response->getBody()->getContents());
}
catch (\GuzzleHttp\Exception\BadResponseException $e) {
// handle exception or api errors.
print_r($e->getMessage());
}
// ...
DELETE /api/boards/{board}/cards/{card}/comments/{comment}
Delete a comment on a card
Parameters
Name | In | Type | Required | Description |
---|---|---|---|---|
board | path | string | true | the board ID of the card |
card | path | string | true | the ID of the card |
comment | path | string | true | the ID of the comment to delete |
Detailed descriptions
board: the board ID of the card
card: the ID of the card
comment: the ID of the comment to delete
Example responses
200 Response
{
"_id": "string"
}
Responses
Status | Meaning | Description | Schema |
---|---|---|---|
200 | OK | 200 response | Inline |
Response Schema
Status Code 200
Name | Type | Required | Restrictions | Description |
---|---|---|---|---|
» _id | string | false | none | none |
Cards
get_cards_by_custom_field
Code samples
# You can also use wget
curl -X GET /api/boards/{board}/cardsByCustomField/{customField}/{customFieldValue} \
-H 'Accept: application/json' \
-H 'Authorization: API_KEY'
GET /api/boards/{board}/cardsByCustomField/{customField}/{customFieldValue} HTTP/1.1
Accept: application/json
const headers = {
'Accept':'application/json',
'Authorization':'API_KEY'
};
fetch('/api/boards/{board}/cardsByCustomField/{customField}/{customFieldValue}',
{
method: 'GET',
headers: headers
})
.then(function(res) {
return res.json();
}).then(function(body) {
console.log(body);
});
const fetch = require('node-fetch');
const headers = {
'Accept':'application/json',
'Authorization':'API_KEY'
};
fetch('/api/boards/{board}/cardsByCustomField/{customField}/{customFieldValue}',
{
method: 'GET',
headers: headers
})
.then(function(res) {
return res.json();
}).then(function(body) {
console.log(body);
});
require 'rest-client'
require 'json'
headers = {
'Accept' => 'application/json',
'Authorization' => 'API_KEY'
}
result = RestClient.get '/api/boards/{board}/cardsByCustomField/{customField}/{customFieldValue}',
params: {
}, headers: headers
p JSON.parse(result)
import requests
headers = {
'Accept': 'application/json',
'Authorization': 'API_KEY'
}
r = requests.get('/api/boards/{board}/cardsByCustomField/{customField}/{customFieldValue}', headers = headers)
print(r.json())
URL obj = new URL("/api/boards/{board}/cardsByCustomField/{customField}/{customFieldValue}");
HttpURLConnection con = (HttpURLConnection) obj.openConnection();
con.setRequestMethod("GET");
int responseCode = con.getResponseCode();
BufferedReader in = new BufferedReader(
new InputStreamReader(con.getInputStream()));
String inputLine;
StringBuffer response = new StringBuffer();
while ((inputLine = in.readLine()) != null) {
response.append(inputLine);
}
in.close();
System.out.println(response.toString());
package main
import (
"bytes"
"net/http"
)
func main() {
headers := map[string][]string{
"Accept": []string{"application/json"},
"Authorization": []string{"API_KEY"},
}
data := bytes.NewBuffer([]byte{jsonReq})
req, err := http.NewRequest("GET", "/api/boards/{board}/cardsByCustomField/{customField}/{customFieldValue}", data)
req.Header = headers
client := &http.Client{}
resp, err := client.Do(req)
// ...
}
'application/json',
'Authorization' => 'API_KEY',
);
$client = new \GuzzleHttp\Client();
// Define array of request body.
$request_body = array();
try {
$response = $client->request('GET','/api/boards/{board}/cardsByCustomField/{customField}/{customFieldValue}', array(
'headers' => $headers,
'json' => $request_body,
)
);
print_r($response->getBody()->getContents());
}
catch (\GuzzleHttp\Exception\BadResponseException $e) {
// handle exception or api errors.
print_r($e->getMessage());
}
// ...
GET /api/boards/{board}/cardsByCustomField/{customField}/{customFieldValue}
Get all Cards that matchs a value of a specific custom field
Parameters
Name | In | Type | Required | Description |
---|---|---|---|---|
board | path | string | true | the board ID |
customField | path | string | true | the list ID |
customFieldValue | path | string | true | the value to look for |
Detailed descriptions
board: the board ID
customField: the list ID
customFieldValue: the value to look for
Example responses
200 Response
[
{
"_id": "string",
"title": "string",
"description": "string",
"listId": "string",
"swinlaneId": "string"
}
]
Responses
Status | Meaning | Description | Schema |
---|---|---|---|
200 | OK | 200 response | Inline |
Response Schema
Status Code 200
Name | Type | Required | Restrictions | Description |
---|---|---|---|---|
» _id | string | false | none | none |
» title | string | false | none | none |
» description | string | false | none | none |
» listId | string | false | none | none |
» swinlaneId | string | false | none | none |
get_board_cards_count
Code samples
# You can also use wget
curl -X GET /api/boards/{board}/cards_count \
-H 'Accept: application/json' \
-H 'Authorization: API_KEY'
GET /api/boards/{board}/cards_count HTTP/1.1
Accept: application/json
const headers = {
'Accept':'application/json',
'Authorization':'API_KEY'
};
fetch('/api/boards/{board}/cards_count',
{
method: 'GET',
headers: headers
})
.then(function(res) {
return res.json();
}).then(function(body) {
console.log(body);
});
const fetch = require('node-fetch');
const headers = {
'Accept':'application/json',
'Authorization':'API_KEY'
};
fetch('/api/boards/{board}/cards_count',
{
method: 'GET',
headers: headers
})
.then(function(res) {
return res.json();
}).then(function(body) {
console.log(body);
});
require 'rest-client'
require 'json'
headers = {
'Accept' => 'application/json',
'Authorization' => 'API_KEY'
}
result = RestClient.get '/api/boards/{board}/cards_count',
params: {
}, headers: headers
p JSON.parse(result)
import requests
headers = {
'Accept': 'application/json',
'Authorization': 'API_KEY'
}
r = requests.get('/api/boards/{board}/cards_count', headers = headers)
print(r.json())
URL obj = new URL("/api/boards/{board}/cards_count");
HttpURLConnection con = (HttpURLConnection) obj.openConnection();
con.setRequestMethod("GET");
int responseCode = con.getResponseCode();
BufferedReader in = new BufferedReader(
new InputStreamReader(con.getInputStream()));
String inputLine;
StringBuffer response = new StringBuffer();
while ((inputLine = in.readLine()) != null) {
response.append(inputLine);
}
in.close();
System.out.println(response.toString());
package main
import (
"bytes"
"net/http"
)
func main() {
headers := map[string][]string{
"Accept": []string{"application/json"},
"Authorization": []string{"API_KEY"},
}
data := bytes.NewBuffer([]byte{jsonReq})
req, err := http.NewRequest("GET", "/api/boards/{board}/cards_count", data)
req.Header = headers
client := &http.Client{}
resp, err := client.Do(req)
// ...
}
'application/json',
'Authorization' => 'API_KEY',
);
$client = new \GuzzleHttp\Client();
// Define array of request body.
$request_body = array();
try {
$response = $client->request('GET','/api/boards/{board}/cards_count', array(
'headers' => $headers,
'json' => $request_body,
)
);
print_r($response->getBody()->getContents());
}
catch (\GuzzleHttp\Exception\BadResponseException $e) {
// handle exception or api errors.
print_r($e->getMessage());
}
// ...
GET /api/boards/{board}/cards_count
Get a cards count to a board
Parameters
Name | In | Type | Required | Description |
---|---|---|---|---|
board | path | string | true | the board ID |
Detailed descriptions
board: the board ID
Example responses
200 Response
{
"board_cards_count": 0
}
Responses
Status | Meaning | Description | Schema |
---|---|---|---|
200 | OK | 200 response | Inline |
Response Schema
Status Code 200
Name | Type | Required | Restrictions | Description |
---|---|---|---|---|
» board_cards_count | integer | false | none | none |
get_all_cards
Code samples
# You can also use wget
curl -X GET /api/boards/{board}/lists/{list}/cards \
-H 'Accept: application/json' \
-H 'Authorization: API_KEY'
GET /api/boards/{board}/lists/{list}/cards HTTP/1.1
Accept: application/json
const headers = {
'Accept':'application/json',
'Authorization':'API_KEY'
};
fetch('/api/boards/{board}/lists/{list}/cards',
{
method: 'GET',
headers: headers
})
.then(function(res) {
return res.json();
}).then(function(body) {
console.log(body);
});
const fetch = require('node-fetch');
const headers = {
'Accept':'application/json',
'Authorization':'API_KEY'
};
fetch('/api/boards/{board}/lists/{list}/cards',
{
method: 'GET',
headers: headers
})
.then(function(res) {
return res.json();
}).then(function(body) {
console.log(body);
});
require 'rest-client'
require 'json'
headers = {
'Accept' => 'application/json',
'Authorization' => 'API_KEY'
}
result = RestClient.get '/api/boards/{board}/lists/{list}/cards',
params: {
}, headers: headers
p JSON.parse(result)
import requests
headers = {
'Accept': 'application/json',
'Authorization': 'API_KEY'
}
r = requests.get('/api/boards/{board}/lists/{list}/cards', headers = headers)
print(r.json())
URL obj = new URL("/api/boards/{board}/lists/{list}/cards");
HttpURLConnection con = (HttpURLConnection) obj.openConnection();
con.setRequestMethod("GET");
int responseCode = con.getResponseCode();
BufferedReader in = new BufferedReader(
new InputStreamReader(con.getInputStream()));
String inputLine;
StringBuffer response = new StringBuffer();
while ((inputLine = in.readLine()) != null) {
response.append(inputLine);
}
in.close();
System.out.println(response.toString());
package main
import (
"bytes"
"net/http"
)
func main() {
headers := map[string][]string{
"Accept": []string{"application/json"},
"Authorization": []string{"API_KEY"},
}
data := bytes.NewBuffer([]byte{jsonReq})
req, err := http.NewRequest("GET", "/api/boards/{board}/lists/{list}/cards", data)
req.Header = headers
client := &http.Client{}
resp, err := client.Do(req)
// ...
}
'application/json',
'Authorization' => 'API_KEY',
);
$client = new \GuzzleHttp\Client();
// Define array of request body.
$request_body = array();
try {
$response = $client->request('GET','/api/boards/{board}/lists/{list}/cards', array(
'headers' => $headers,
'json' => $request_body,
)
);
print_r($response->getBody()->getContents());
}
catch (\GuzzleHttp\Exception\BadResponseException $e) {
// handle exception or api errors.
print_r($e->getMessage());
}
// ...
GET /api/boards/{board}/lists/{list}/cards
Get all Cards attached to a List
Parameters
Name | In | Type | Required | Description |
---|---|---|---|---|
board | path | string | true | the board ID |
list | path | string | true | the list ID |
Detailed descriptions
board: the board ID
list: the list ID
Example responses
200 Response
[
{
"_id": "string",
"title": "string",
"description": "string"
}
]
Responses
Status | Meaning | Description | Schema |
---|---|---|---|
200 | OK | 200 response | Inline |
Response Schema
Status Code 200
Name | Type | Required | Restrictions | Description |
---|---|---|---|---|
» _id | string | false | none | none |
» title | string | false | none | none |
» description | string | false | none | none |
new_card
Code samples
# You can also use wget
curl -X POST /api/boards/{board}/lists/{list}/cards \
-H 'Content-Type: multipart/form-data' \
-H 'Accept: application/json' \
-H 'Authorization: API_KEY'
POST /api/boards/{board}/lists/{list}/cards HTTP/1.1
Content-Type: multipart/form-data
Accept: application/json
const inputBody = '{
"authorId": "string",
"members": "string",
"assignees": "string",
"title": "string",
"description": "string",
"swimlaneId": "string"
}';
const headers = {
'Content-Type':'multipart/form-data',
'Accept':'application/json',
'Authorization':'API_KEY'
};
fetch('/api/boards/{board}/lists/{list}/cards',
{
method: 'POST',
body: inputBody,
headers: headers
})
.then(function(res) {
return res.json();
}).then(function(body) {
console.log(body);
});
const fetch = require('node-fetch');
const inputBody = {
"authorId": "string",
"members": "string",
"assignees": "string",
"title": "string",
"description": "string",
"swimlaneId": "string"
};
const headers = {
'Content-Type':'multipart/form-data',
'Accept':'application/json',
'Authorization':'API_KEY'
};
fetch('/api/boards/{board}/lists/{list}/cards',
{
method: 'POST',
body: JSON.stringify(inputBody),
headers: headers
})
.then(function(res) {
return res.json();
}).then(function(body) {
console.log(body);
});
require 'rest-client'
require 'json'
headers = {
'Content-Type' => 'multipart/form-data',
'Accept' => 'application/json',
'Authorization' => 'API_KEY'
}
result = RestClient.post '/api/boards/{board}/lists/{list}/cards',
params: {
}, headers: headers
p JSON.parse(result)
import requests
headers = {
'Content-Type': 'multipart/form-data',
'Accept': 'application/json',
'Authorization': 'API_KEY'
}
r = requests.post('/api/boards/{board}/lists/{list}/cards', headers = headers)
print(r.json())
URL obj = new URL("/api/boards/{board}/lists/{list}/cards");
HttpURLConnection con = (HttpURLConnection) obj.openConnection();
con.setRequestMethod("POST");
int responseCode = con.getResponseCode();
BufferedReader in = new BufferedReader(
new InputStreamReader(con.getInputStream()));
String inputLine;
StringBuffer response = new StringBuffer();
while ((inputLine = in.readLine()) != null) {
response.append(inputLine);
}
in.close();
System.out.println(response.toString());
package main
import (
"bytes"
"net/http"
)
func main() {
headers := map[string][]string{
"Content-Type": []string{"multipart/form-data"},
"Accept": []string{"application/json"},
"Authorization": []string{"API_KEY"},
}
data := bytes.NewBuffer([]byte{jsonReq})
req, err := http.NewRequest("POST", "/api/boards/{board}/lists/{list}/cards", data)
req.Header = headers
client := &http.Client{}
resp, err := client.Do(req)
// ...
}
'multipart/form-data',
'Accept' => 'application/json',
'Authorization' => 'API_KEY',
);
$client = new \GuzzleHttp\Client();
// Define array of request body.
$request_body = array();
try {
$response = $client->request('POST','/api/boards/{board}/lists/{list}/cards', array(
'headers' => $headers,
'json' => $request_body,
)
);
print_r($response->getBody()->getContents());
}
catch (\GuzzleHttp\Exception\BadResponseException $e) {
// handle exception or api errors.
print_r($e->getMessage());
}
// ...
POST /api/boards/{board}/lists/{list}/cards
Create a new Card
Body parameter
authorId: string
members: string
assignees: string
title: string
description: string
swimlaneId: string
Parameters
Name | In | Type | Required | Description |
---|---|---|---|---|
board | path | string | true | the board ID of the new card |
list | path | string | true | the list ID of the new card |
body | body | object | true | none |
» authorId | body | string | true | the authorId value |
» members | body | string | false | the member IDs list of the new card |
» assignees | body | string | false | the assignee IDs list of the new card |
» title | body | string | true | the title of the new card |
» description | body | string | true | the description of the new card |
» swimlaneId | body | string | true | the swimlane ID of the new card |
Detailed descriptions
board: the board ID of the new card
list: the list ID of the new card
Example responses
200 Response
{
"_id": "string"
}
Responses
Status | Meaning | Description | Schema |
---|---|---|---|
200 | OK | 200 response | Inline |
Response Schema
Status Code 200
Name | Type | Required | Restrictions | Description |
---|---|---|---|---|
» _id | string | false | none | none |
get_card
Code samples
# You can also use wget
curl -X GET /api/boards/{board}/lists/{list}/cards/{card} \
-H 'Accept: application/json' \
-H 'Authorization: API_KEY'
GET /api/boards/{board}/lists/{list}/cards/{card} HTTP/1.1
Accept: application/json
const headers = {
'Accept':'application/json',
'Authorization':'API_KEY'
};
fetch('/api/boards/{board}/lists/{list}/cards/{card}',
{
method: 'GET',
headers: headers
})
.then(function(res) {
return res.json();
}).then(function(body) {
console.log(body);
});
const fetch = require('node-fetch');
const headers = {
'Accept':'application/json',
'Authorization':'API_KEY'
};
fetch('/api/boards/{board}/lists/{list}/cards/{card}',
{
method: 'GET',
headers: headers
})
.then(function(res) {
return res.json();
}).then(function(body) {
console.log(body);
});
require 'rest-client'
require 'json'
headers = {
'Accept' => 'application/json',
'Authorization' => 'API_KEY'
}
result = RestClient.get '/api/boards/{board}/lists/{list}/cards/{card}',
params: {
}, headers: headers
p JSON.parse(result)
import requests
headers = {
'Accept': 'application/json',
'Authorization': 'API_KEY'
}
r = requests.get('/api/boards/{board}/lists/{list}/cards/{card}', headers = headers)
print(r.json())
URL obj = new URL("/api/boards/{board}/lists/{list}/cards/{card}");
HttpURLConnection con = (HttpURLConnection) obj.openConnection();
con.setRequestMethod("GET");
int responseCode = con.getResponseCode();
BufferedReader in = new BufferedReader(
new InputStreamReader(con.getInputStream()));
String inputLine;
StringBuffer response = new StringBuffer();
while ((inputLine = in.readLine()) != null) {
response.append(inputLine);
}
in.close();
System.out.println(response.toString());
package main
import (
"bytes"
"net/http"
)
func main() {
headers := map[string][]string{
"Accept": []string{"application/json"},
"Authorization": []string{"API_KEY"},
}
data := bytes.NewBuffer([]byte{jsonReq})
req, err := http.NewRequest("GET", "/api/boards/{board}/lists/{list}/cards/{card}", data)
req.Header = headers
client := &http.Client{}
resp, err := client.Do(req)
// ...
}
'application/json',
'Authorization' => 'API_KEY',
);
$client = new \GuzzleHttp\Client();
// Define array of request body.
$request_body = array();
try {
$response = $client->request('GET','/api/boards/{board}/lists/{list}/cards/{card}', array(
'headers' => $headers,
'json' => $request_body,
)
);
print_r($response->getBody()->getContents());
}
catch (\GuzzleHttp\Exception\BadResponseException $e) {
// handle exception or api errors.
print_r($e->getMessage());
}
// ...
GET /api/boards/{board}/lists/{list}/cards/{card}
Get a Card
Parameters
Name | In | Type | Required | Description |
---|---|---|---|---|
board | path | string | true | the board ID |
list | path | string | true | the list ID of the card |
card | path | string | true | the card ID |
Detailed descriptions
board: the board ID
list: the list ID of the card
card: the card ID
Example responses
200 Response
{
"title": "string",
"archived": true,
"archivedAt": "string",
"parentId": "string",
"listId": "string",
"swimlaneId": "string",
"boardId": "string",
"coverId": "string",
"color": "white",
"createdAt": "string",
"modifiedAt": "string",
"customFields": [
{}
],
"dateLastActivity": "string",
"description": "string",
"requestedBy": "string",
"assignedBy": "string",
"labelIds": [
"string"
],
"members": [
"string"
],
"assignees": [
"string"
],
"receivedAt": "string",
"startAt": "string",
"dueAt": "string",
"endAt": "string",
"spentTime": 0,
"isOvertime": true,
"userId": "string",
"sort": 0,
"subtaskSort": 0,
"type": "string",
"linkedId": "string",
"vote": {
"question": "string",
"positive": [
"string"
],
"negative": [
"string"
],
"end": "string",
"public": true,
"allowNonBoardMembers": true
},
"poker": {
"question": true,
"one": [
"string"
],
"two": [
"string"
],
"three": [
"string"
],
"five": [
"string"
],
"eight": [
"string"
],
"thirteen": [
"string"
],
"twenty": [
"string"
],
"forty": [
"string"
],
"oneHundred": [
"string"
],
"unsure": [
"string"
],
"end": "string",
"allowNonBoardMembers": true,
"estimation": 0
},
"targetId_gantt": [
"string"
],
"linkType_gantt": [
0
],
"linkId_gantt": [
"string"
],
"cardNumber": 0
}
Responses
Status | Meaning | Description | Schema |
---|---|---|---|
200 | OK | 200 response | Cards |
edit_card
Code samples
# You can also use wget
curl -X PUT /api/boards/{board}/lists/{list}/cards/{card} \
-H 'Content-Type: multipart/form-data' \
-H 'Accept: application/json' \
-H 'Authorization: API_KEY'
PUT /api/boards/{board}/lists/{list}/cards/{card} HTTP/1.1
Content-Type: multipart/form-data
Accept: application/json
const inputBody = '{
"newBoardId": "string",
"newSwimlaneId": "string",
"newListId": "string",
"title": "string",
"sort": "string",
"parentId": "string",
"description": "string",
"color": "string",
"vote": {},
"poker": {},
"labelIds": "string",
"requestedBy": "string",
"assignedBy": "string",
"receivedAt": "string",
"startAt": "string",
"dueAt": "string",
"endAt": "string",
"spentTime": "string",
"isOverTime": true,
"customFields": "string",
"members": "string",
"assignees": "string",
"swimlaneId": "string",
"listId": "string",
"authorId": "string",
"archive": "string"
}';
const headers = {
'Content-Type':'multipart/form-data',
'Accept':'application/json',
'Authorization':'API_KEY'
};
fetch('/api/boards/{board}/lists/{list}/cards/{card}',
{
method: 'PUT',
body: inputBody,
headers: headers
})
.then(function(res) {
return res.json();
}).then(function(body) {
console.log(body);
});
const fetch = require('node-fetch');
const inputBody = {
"newBoardId": "string",
"newSwimlaneId": "string",
"newListId": "string",
"title": "string",
"sort": "string",
"parentId": "string",
"description": "string",
"color": "string",
"vote": {},
"poker": {},
"labelIds": "string",
"requestedBy": "string",
"assignedBy": "string",
"receivedAt": "string",
"startAt": "string",
"dueAt": "string",
"endAt": "string",
"spentTime": "string",
"isOverTime": true,
"customFields": "string",
"members": "string",
"assignees": "string",
"swimlaneId": "string",
"listId": "string",
"authorId": "string",
"archive": "string"
};
const headers = {
'Content-Type':'multipart/form-data',
'Accept':'application/json',
'Authorization':'API_KEY'
};
fetch('/api/boards/{board}/lists/{list}/cards/{card}',
{
method: 'PUT',
body: JSON.stringify(inputBody),
headers: headers
})
.then(function(res) {
return res.json();
}).then(function(body) {
console.log(body);
});
require 'rest-client'
require 'json'
headers = {
'Content-Type' => 'multipart/form-data',
'Accept' => 'application/json',
'Authorization' => 'API_KEY'
}
result = RestClient.put '/api/boards/{board}/lists/{list}/cards/{card}',
params: {
}, headers: headers
p JSON.parse(result)
import requests
headers = {
'Content-Type': 'multipart/form-data',
'Accept': 'application/json',
'Authorization': 'API_KEY'
}
r = requests.put('/api/boards/{board}/lists/{list}/cards/{card}', headers = headers)
print(r.json())
URL obj = new URL("/api/boards/{board}/lists/{list}/cards/{card}");
HttpURLConnection con = (HttpURLConnection) obj.openConnection();
con.setRequestMethod("PUT");
int responseCode = con.getResponseCode();
BufferedReader in = new BufferedReader(
new InputStreamReader(con.getInputStream()));
String inputLine;
StringBuffer response = new StringBuffer();
while ((inputLine = in.readLine()) != null) {
response.append(inputLine);
}
in.close();
System.out.println(response.toString());
package main
import (
"bytes"
"net/http"
)
func main() {
headers := map[string][]string{
"Content-Type": []string{"multipart/form-data"},
"Accept": []string{"application/json"},
"Authorization": []string{"API_KEY"},
}
data := bytes.NewBuffer([]byte{jsonReq})
req, err := http.NewRequest("PUT", "/api/boards/{board}/lists/{list}/cards/{card}", data)
req.Header = headers
client := &http.Client{}
resp, err := client.Do(req)
// ...
}
'multipart/form-data',
'Accept' => 'application/json',
'Authorization' => 'API_KEY',
);
$client = new \GuzzleHttp\Client();
// Define array of request body.
$request_body = array();
try {
$response = $client->request('PUT','/api/boards/{board}/lists/{list}/cards/{card}', array(
'headers' => $headers,
'json' => $request_body,
)
);
print_r($response->getBody()->getContents());
}
catch (\GuzzleHttp\Exception\BadResponseException $e) {
// handle exception or api errors.
print_r($e->getMessage());
}
// ...
PUT /api/boards/{board}/lists/{list}/cards/{card}
Edit Fields in a Card
Edit a card
The color has to be chosen between white
, green
, yellow
, orange
,
red
, purple
, blue
, sky
, lime
, pink
, black
, silver
,
peachpuff
, crimson
, plum
, darkgreen
, slateblue
, magenta
,
gold
, navy
, gray
, saddlebrown
, paleturquoise
, mistyrose
,
indigo
:

Note: setting the color to white has the same effect than removing it.
Body parameter
newBoardId: string
newSwimlaneId: string
newListId: string
title: string
sort: string
parentId: string
description: string
color: string
vote: {}
poker: {}
labelIds: string
requestedBy: string
assignedBy: string
receivedAt: string
startAt: string
dueAt: string
endAt: string
spentTime: string
isOverTime: true
customFields: string
members: string
assignees: string
swimlaneId: string
listId: string
authorId: string
archive: string
Parameters
Name | In | Type | Required | Description |
---|---|---|---|---|
board | path | string | true | the board ID of the card |
list | path | string | true | the list ID of the card |
card | path | string | true | the ID of the card |
body | body | object | true | none |
» newBoardId | body | string | true | the newBoardId value |
» newSwimlaneId | body | string | true | the newSwimlaneId value |
» newListId | body | string | true | the newListId value |
» title | body | string | false | the new title of the card |
» sort | body | string | false | the new sort value of the card |
» parentId | body | string | false | change the parent of the card |
» description | body | string | false | the new description of the card |
» color | body | string | false | the new color of the card |
» vote | body | object | false | the vote object |
» poker | body | object | false | the poker object |
» labelIds | body | string | false | the new list of label IDs attached to the card |
» requestedBy | body | string | false | the new requestedBy field of the card |
» assignedBy | body | string | false | the new assignedBy field of the card |
» receivedAt | body | string | false | the new receivedAt field of the card |
» startAt | body | string | false | the new startAt field of the card |
» dueAt | body | string | false | the new dueAt field of the card |
» endAt | body | string | false | the new endAt field of the card |
» spentTime | body | string | false | the new spentTime field of the card |
» isOverTime | body | boolean | false | the new isOverTime field of the card |
» customFields | body | string | false | the new customFields value of the card |
» members | body | string | false | the new list of member IDs attached to the card |
» assignees | body | string | false | the array of maximum one ID of assignee attached to the card |
» swimlaneId | body | string | false | the new swimlane ID of the card |
» listId | body | string | false | the new list ID of the card (move operation) |
» authorId | body | string | false | change the owner of the card |
» archive | body | string | true | the archive value |
Detailed descriptions
board: the board ID of the card
list: the list ID of the card
card: the ID of the card
Example responses
200 Response
{
"_id": "string"
}
Responses
Status | Meaning | Description | Schema |
---|---|---|---|
200 | OK | 200 response | Inline |
Response Schema
Status Code 200
Name | Type | Required | Restrictions | Description |
---|---|---|---|---|
» _id | string | false | none | none |
delete_card
Code samples
# You can also use wget
curl -X DELETE /api/boards/{board}/lists/{list}/cards/{card} \
-H 'Accept: application/json' \
-H 'Authorization: API_KEY'
DELETE /api/boards/{board}/lists/{list}/cards/{card} HTTP/1.1
Accept: application/json
const headers = {
'Accept':'application/json',
'Authorization':'API_KEY'
};
fetch('/api/boards/{board}/lists/{list}/cards/{card}',
{
method: 'DELETE',
headers: headers
})
.then(function(res) {
return res.json();
}).then(function(body) {
console.log(body);
});
const fetch = require('node-fetch');
const headers = {
'Accept':'application/json',
'Authorization':'API_KEY'
};
fetch('/api/boards/{board}/lists/{list}/cards/{card}',
{
method: 'DELETE',
headers: headers
})
.then(function(res) {
return res.json();
}).then(function(body) {
console.log(body);
});
require 'rest-client'
require 'json'
headers = {
'Accept' => 'application/json',
'Authorization' => 'API_KEY'
}
result = RestClient.delete '/api/boards/{board}/lists/{list}/cards/{card}',
params: {
}, headers: headers
p JSON.parse(result)
import requests
headers = {
'Accept': 'application/json',
'Authorization': 'API_KEY'
}
r = requests.delete('/api/boards/{board}/lists/{list}/cards/{card}', headers = headers)
print(r.json())
URL obj = new URL("/api/boards/{board}/lists/{list}/cards/{card}");
HttpURLConnection con = (HttpURLConnection) obj.openConnection();
con.setRequestMethod("DELETE");
int responseCode = con.getResponseCode();
BufferedReader in = new BufferedReader(
new InputStreamReader(con.getInputStream()));
String inputLine;
StringBuffer response = new StringBuffer();
while ((inputLine = in.readLine()) != null) {
response.append(inputLine);
}
in.close();
System.out.println(response.toString());
package main
import (
"bytes"
"net/http"
)
func main() {
headers := map[string][]string{
"Accept": []string{"application/json"},
"Authorization": []string{"API_KEY"},
}
data := bytes.NewBuffer([]byte{jsonReq})
req, err := http.NewRequest("DELETE", "/api/boards/{board}/lists/{list}/cards/{card}", data)
req.Header = headers
client := &http.Client{}
resp, err := client.Do(req)
// ...
}
'application/json',
'Authorization' => 'API_KEY',
);
$client = new \GuzzleHttp\Client();
// Define array of request body.
$request_body = array();
try {
$response = $client->request('DELETE','/api/boards/{board}/lists/{list}/cards/{card}', array(
'headers' => $headers,
'json' => $request_body,
)
);
print_r($response->getBody()->getContents());
}
catch (\GuzzleHttp\Exception\BadResponseException $e) {
// handle exception or api errors.
print_r($e->getMessage());
}
// ...
DELETE /api/boards/{board}/lists/{list}/cards/{card}
Delete a card from a board
This operation deletes a card, and therefore the card is not put in the recycle bin.
Parameters
Name | In | Type | Required | Description |
---|---|---|---|---|
board | path | string | true | the board ID of the card |
list | path | string | true | the list ID of the card |
card | path | string | true | the ID of the card |
Detailed descriptions
board: the board ID of the card
list: the list ID of the card
card: the ID of the card
Example responses
200 Response
{
"_id": "string"
}
Responses
Status | Meaning | Description | Schema |
---|---|---|---|
200 | OK | 200 response | Inline |
Response Schema
Status Code 200
Name | Type | Required | Restrictions | Description |
---|---|---|---|---|
» _id | string | false | none | none |
edit_card_custom_field
Code samples
# You can also use wget
curl -X POST /api/boards/{board}/lists/{list}/cards/{card}/customFields/{customField} \
-H 'Content-Type: multipart/form-data' \
-H 'Accept: application/json' \
-H 'Authorization: API_KEY'
POST /api/boards/{board}/lists/{list}/cards/{card}/customFields/{customField} HTTP/1.1
Content-Type: multipart/form-data
Accept: application/json
const inputBody = '{
"value": "string"
}';
const headers = {
'Content-Type':'multipart/form-data',
'Accept':'application/json',
'Authorization':'API_KEY'
};
fetch('/api/boards/{board}/lists/{list}/cards/{card}/customFields/{customField}',
{
method: 'POST',
body: inputBody,
headers: headers
})
.then(function(res) {
return res.json();
}).then(function(body) {
console.log(body);
});
const fetch = require('node-fetch');
const inputBody = {
"value": "string"
};
const headers = {
'Content-Type':'multipart/form-data',
'Accept':'application/json',
'Authorization':'API_KEY'
};
fetch('/api/boards/{board}/lists/{list}/cards/{card}/customFields/{customField}',
{
method: 'POST',
body: JSON.stringify(inputBody),
headers: headers
})
.then(function(res) {
return res.json();
}).then(function(body) {
console.log(body);
});
require 'rest-client'
require 'json'
headers = {
'Content-Type' => 'multipart/form-data',
'Accept' => 'application/json',
'Authorization' => 'API_KEY'
}
result = RestClient.post '/api/boards/{board}/lists/{list}/cards/{card}/customFields/{customField}',
params: {
}, headers: headers
p JSON.parse(result)
import requests
headers = {
'Content-Type': 'multipart/form-data',
'Accept': 'application/json',
'Authorization': 'API_KEY'
}
r = requests.post('/api/boards/{board}/lists/{list}/cards/{card}/customFields/{customField}', headers = headers)
print(r.json())
URL obj = new URL("/api/boards/{board}/lists/{list}/cards/{card}/customFields/{customField}");
HttpURLConnection con = (HttpURLConnection) obj.openConnection();
con.setRequestMethod("POST");
int responseCode = con.getResponseCode();
BufferedReader in = new BufferedReader(
new InputStreamReader(con.getInputStream()));
String inputLine;
StringBuffer response = new StringBuffer();
while ((inputLine = in.readLine()) != null) {
response.append(inputLine);
}
in.close();
System.out.println(response.toString());
package main
import (
"bytes"
"net/http"
)
func main() {
headers := map[string][]string{
"Content-Type": []string{"multipart/form-data"},
"Accept": []string{"application/json"},
"Authorization": []string{"API_KEY"},
}
data := bytes.NewBuffer([]byte{jsonReq})
req, err := http.NewRequest("POST", "/api/boards/{board}/lists/{list}/cards/{card}/customFields/{customField}", data)
req.Header = headers
client := &http.Client{}
resp, err := client.Do(req)
// ...
}
'multipart/form-data',
'Accept' => 'application/json',
'Authorization' => 'API_KEY',
);
$client = new \GuzzleHttp\Client();
// Define array of request body.
$request_body = array();
try {
$response = $client->request('POST','/api/boards/{board}/lists/{list}/cards/{card}/customFields/{customField}', array(
'headers' => $headers,
'json' => $request_body,
)
);
print_r($response->getBody()->getContents());
}
catch (\GuzzleHttp\Exception\BadResponseException $e) {
// handle exception or api errors.
print_r($e->getMessage());
}
// ...
POST /api/boards/{board}/lists/{list}/cards/{card}/customFields/{customField}
Edit Custom Field in a Card
Edit a custom field value in a card
Body parameter
value: string
Parameters
Name | In | Type | Required | Description |
---|---|---|---|---|
board | path | string | true | the board ID of the card |
list | path | string | true | the list ID of the card |
card | path | string | true | the ID of the card |
customField | path | string | true | the ID of the custom field |
body | body | object | true | none |
» value | body | string | true | the new custom field value |
Detailed descriptions
board: the board ID of the card
list: the list ID of the card
card: the ID of the card
customField: the ID of the custom field
Example responses
200 Response
{
"_id": "string",
"customFields": {}
}
Responses
Status | Meaning | Description | Schema |
---|---|---|---|
200 | OK | 200 response | Inline |
Response Schema
Status Code 200
Name | Type | Required | Restrictions | Description |
---|---|---|---|---|
» _id | string | false | none | none |
» customFields | object | false | none | none |
get_list_cards_count
Code samples
# You can also use wget
curl -X GET /api/boards/{board}/lists/{list}/cards_count \
-H 'Accept: application/json' \
-H 'Authorization: API_KEY'
GET /api/boards/{board}/lists/{list}/cards_count HTTP/1.1
Accept: application/json
const headers = {
'Accept':'application/json',
'Authorization':'API_KEY'
};
fetch('/api/boards/{board}/lists/{list}/cards_count',
{
method: 'GET',
headers: headers
})
.then(function(res) {
return res.json();
}).then(function(body) {
console.log(body);
});
const fetch = require('node-fetch');
const headers = {
'Accept':'application/json',
'Authorization':'API_KEY'
};
fetch('/api/boards/{board}/lists/{list}/cards_count',
{
method: 'GET',
headers: headers
})
.then(function(res) {
return res.json();
}).then(function(body) {
console.log(body);
});
require 'rest-client'
require 'json'
headers = {
'Accept' => 'application/json',
'Authorization' => 'API_KEY'
}
result = RestClient.get '/api/boards/{board}/lists/{list}/cards_count',
params: {
}, headers: headers
p JSON.parse(result)
import requests
headers = {
'Accept': 'application/json',
'Authorization': 'API_KEY'
}
r = requests.get('/api/boards/{board}/lists/{list}/cards_count', headers = headers)
print(r.json())
URL obj = new URL("/api/boards/{board}/lists/{list}/cards_count");
HttpURLConnection con = (HttpURLConnection) obj.openConnection();
con.setRequestMethod("GET");
int responseCode = con.getResponseCode();
BufferedReader in = new BufferedReader(
new InputStreamReader(con.getInputStream()));
String inputLine;
StringBuffer response = new StringBuffer();
while ((inputLine = in.readLine()) != null) {
response.append(inputLine);
}
in.close();
System.out.println(response.toString());
package main
import (
"bytes"
"net/http"
)
func main() {
headers := map[string][]string{
"Accept": []string{"application/json"},
"Authorization": []string{"API_KEY"},
}
data := bytes.NewBuffer([]byte{jsonReq})
req, err := http.NewRequest("GET", "/api/boards/{board}/lists/{list}/cards_count", data)
req.Header = headers
client := &http.Client{}
resp, err := client.Do(req)
// ...
}
'application/json',
'Authorization' => 'API_KEY',
);
$client = new \GuzzleHttp\Client();
// Define array of request body.
$request_body = array();
try {
$response = $client->request('GET','/api/boards/{board}/lists/{list}/cards_count', array(
'headers' => $headers,
'json' => $request_body,
)
);
print_r($response->getBody()->getContents());
}
catch (\GuzzleHttp\Exception\BadResponseException $e) {
// handle exception or api errors.
print_r($e->getMessage());
}
// ...
GET /api/boards/{board}/lists/{list}/cards_count
Get a cards count to a list
Parameters
Name | In | Type | Required | Description |
---|---|---|---|---|
board | path | string | true | the board ID |
list | path | string | true | the List ID |
Detailed descriptions
board: the board ID
list: the List ID
Example responses
200 Response
{
"list_cards_count": 0
}
Responses
Status | Meaning | Description | Schema |
---|---|---|---|
200 | OK | 200 response | Inline |
Response Schema
Status Code 200
Name | Type | Required | Restrictions | Description |
---|---|---|---|---|
» list_cards_count | integer | false | none | none |
get_swimlane_cards
Code samples
# You can also use wget
curl -X GET /api/boards/{board}/swimlanes/{swimlane}/cards \
-H 'Accept: application/json' \
-H 'Authorization: API_KEY'
GET /api/boards/{board}/swimlanes/{swimlane}/cards HTTP/1.1
Accept: application/json
const headers = {
'Accept':'application/json',
'Authorization':'API_KEY'
};
fetch('/api/boards/{board}/swimlanes/{swimlane}/cards',
{
method: 'GET',
headers: headers
})
.then(function(res) {
return res.json();
}).then(function(body) {
console.log(body);
});
const fetch = require('node-fetch');
const headers = {
'Accept':'application/json',
'Authorization':'API_KEY'
};
fetch('/api/boards/{board}/swimlanes/{swimlane}/cards',
{
method: 'GET',
headers: headers
})
.then(function(res) {
return res.json();
}).then(function(body) {
console.log(body);
});
require 'rest-client'
require 'json'
headers = {
'Accept' => 'application/json',
'Authorization' => 'API_KEY'
}
result = RestClient.get '/api/boards/{board}/swimlanes/{swimlane}/cards',
params: {
}, headers: headers
p JSON.parse(result)
import requests
headers = {
'Accept': 'application/json',
'Authorization': 'API_KEY'
}
r = requests.get('/api/boards/{board}/swimlanes/{swimlane}/cards', headers = headers)
print(r.json())
URL obj = new URL("/api/boards/{board}/swimlanes/{swimlane}/cards");
HttpURLConnection con = (HttpURLConnection) obj.openConnection();
con.setRequestMethod("GET");
int responseCode = con.getResponseCode();
BufferedReader in = new BufferedReader(
new InputStreamReader(con.getInputStream()));
String inputLine;
StringBuffer response = new StringBuffer();
while ((inputLine = in.readLine()) != null) {
response.append(inputLine);
}
in.close();
System.out.println(response.toString());
package main
import (
"bytes"
"net/http"
)
func main() {
headers := map[string][]string{
"Accept": []string{"application/json"},
"Authorization": []string{"API_KEY"},
}
data := bytes.NewBuffer([]byte{jsonReq})
req, err := http.NewRequest("GET", "/api/boards/{board}/swimlanes/{swimlane}/cards", data)
req.Header = headers
client := &http.Client{}
resp, err := client.Do(req)
// ...
}
'application/json',
'Authorization' => 'API_KEY',
);
$client = new \GuzzleHttp\Client();
// Define array of request body.
$request_body = array();
try {
$response = $client->request('GET','/api/boards/{board}/swimlanes/{swimlane}/cards', array(
'headers' => $headers,
'json' => $request_body,
)
);
print_r($response->getBody()->getContents());
}
catch (\GuzzleHttp\Exception\BadResponseException $e) {
// handle exception or api errors.
print_r($e->getMessage());
}
// ...
GET /api/boards/{board}/swimlanes/{swimlane}/cards
get all cards attached to a swimlane
Parameters
Name | In | Type | Required | Description |
---|---|---|---|---|
board | path | string | true | the board ID |
swimlane | path | string | true | the swimlane ID |
Detailed descriptions
board: the board ID
swimlane: the swimlane ID
Example responses
200 Response
[
{
"_id": "string",
"title": "string",
"description": "string",
"listId": "string"
}
]
Responses
Status | Meaning | Description | Schema |
---|---|---|---|
200 | OK | 200 response | Inline |
Response Schema
Status Code 200
Name | Type | Required | Restrictions | Description |
---|---|---|---|---|
» _id | string | false | none | none |
» title | string | false | none | none |
» description | string | false | none | none |
» listId | string | false | none | none |
CustomFields
get_all_custom_fields
Code samples
# You can also use wget
curl -X GET /api/boards/{board}/custom-fields \
-H 'Accept: application/json' \
-H 'Authorization: API_KEY'
GET /api/boards/{board}/custom-fields HTTP/1.1
Accept: application/json
const headers = {
'Accept':'application/json',
'Authorization':'API_KEY'
};
fetch('/api/boards/{board}/custom-fields',
{
method: 'GET',
headers: headers
})
.then(function(res) {
return res.json();
}).then(function(body) {
console.log(body);
});
const fetch = require('node-fetch');
const headers = {
'Accept':'application/json',
'Authorization':'API_KEY'
};
fetch('/api/boards/{board}/custom-fields',
{
method: 'GET',
headers: headers
})
.then(function(res) {
return res.json();
}).then(function(body) {
console.log(body);
});
require 'rest-client'
require 'json'
headers = {
'Accept' => 'application/json',
'Authorization' => 'API_KEY'
}
result = RestClient.get '/api/boards/{board}/custom-fields',
params: {
}, headers: headers
p JSON.parse(result)
import requests
headers = {
'Accept': 'application/json',
'Authorization': 'API_KEY'
}
r = requests.get('/api/boards/{board}/custom-fields', headers = headers)
print(r.json())
URL obj = new URL("/api/boards/{board}/custom-fields");
HttpURLConnection con = (HttpURLConnection) obj.openConnection();
con.setRequestMethod("GET");
int responseCode = con.getResponseCode();
BufferedReader in = new BufferedReader(
new InputStreamReader(con.getInputStream()));
String inputLine;
StringBuffer response = new StringBuffer();
while ((inputLine = in.readLine()) != null) {
response.append(inputLine);
}
in.close();
System.out.println(response.toString());
package main
import (
"bytes"
"net/http"
)
func main() {
headers := map[string][]string{
"Accept": []string{"application/json"},
"Authorization": []string{"API_KEY"},
}
data := bytes.NewBuffer([]byte{jsonReq})
req, err := http.NewRequest("GET", "/api/boards/{board}/custom-fields", data)
req.Header = headers
client := &http.Client{}
resp, err := client.Do(req)
// ...
}
'application/json',
'Authorization' => 'API_KEY',
);
$client = new \GuzzleHttp\Client();
// Define array of request body.
$request_body = array();
try {
$response = $client->request('GET','/api/boards/{board}/custom-fields', array(
'headers' => $headers,
'json' => $request_body,
)
);
print_r($response->getBody()->getContents());
}
catch (\GuzzleHttp\Exception\BadResponseException $e) {
// handle exception or api errors.
print_r($e->getMessage());
}
// ...
GET /api/boards/{board}/custom-fields
Get the list of Custom Fields attached to a board
Parameters
Name | In | Type | Required | Description |
---|---|---|---|---|
board | path | string | true | the board value |
Example responses
200 Response
[
{
"_id": "string",
"name": "string",
"type": "string"
}
]
Responses
Status | Meaning | Description | Schema |
---|---|---|---|
200 | OK | 200 response | Inline |
Response Schema
Status Code 200
Name | Type | Required | Restrictions | Description |
---|---|---|---|---|
» _id | string | false | none | none |
» name | string | false | none | none |
» type | string | false | none | none |
new_custom_field
Code samples
# You can also use wget
curl -X POST /api/boards/{board}/custom-fields \
-H 'Content-Type: multipart/form-data' \
-H 'Accept: application/json' \
-H 'Authorization: API_KEY'
POST /api/boards/{board}/custom-fields HTTP/1.1
Content-Type: multipart/form-data
Accept: application/json
const inputBody = '{
"name": "string",
"type": "string",
"settings": "string",
"showOnCard": true,
"automaticallyOnCard": true,
"showLabelOnMiniCard": true,
"showSumAtTopOfList": true,
"authorId": "string"
}';
const headers = {
'Content-Type':'multipart/form-data',
'Accept':'application/json',
'Authorization':'API_KEY'
};
fetch('/api/boards/{board}/custom-fields',
{
method: 'POST',
body: inputBody,
headers: headers
})
.then(function(res) {
return res.json();
}).then(function(body) {
console.log(body);
});
const fetch = require('node-fetch');
const inputBody = {
"name": "string",
"type": "string",
"settings": "string",
"showOnCard": true,
"automaticallyOnCard": true,
"showLabelOnMiniCard": true,
"showSumAtTopOfList": true,
"authorId": "string"
};
const headers = {
'Content-Type':'multipart/form-data',
'Accept':'application/json',
'Authorization':'API_KEY'
};
fetch('/api/boards/{board}/custom-fields',
{
method: 'POST',
body: JSON.stringify(inputBody),
headers: headers
})
.then(function(res) {
return res.json();
}).then(function(body) {
console.log(body);
});
require 'rest-client'
require 'json'
headers = {
'Content-Type' => 'multipart/form-data',
'Accept' => 'application/json',
'Authorization' => 'API_KEY'
}
result = RestClient.post '/api/boards/{board}/custom-fields',
params: {
}, headers: headers
p JSON.parse(result)
import requests
headers = {
'Content-Type': 'multipart/form-data',
'Accept': 'application/json',
'Authorization': 'API_KEY'
}
r = requests.post('/api/boards/{board}/custom-fields', headers = headers)
print(r.json())
URL obj = new URL("/api/boards/{board}/custom-fields");
HttpURLConnection con = (HttpURLConnection) obj.openConnection();
con.setRequestMethod("POST");
int responseCode = con.getResponseCode();
BufferedReader in = new BufferedReader(
new InputStreamReader(con.getInputStream()));
String inputLine;
StringBuffer response = new StringBuffer();
while ((inputLine = in.readLine()) != null) {
response.append(inputLine);
}
in.close();
System.out.println(response.toString());
package main
import (
"bytes"
"net/http"
)
func main() {
headers := map[string][]string{
"Content-Type": []string{"multipart/form-data"},
"Accept": []string{"application/json"},
"Authorization": []string{"API_KEY"},
}
data := bytes.NewBuffer([]byte{jsonReq})
req, err := http.NewRequest("POST", "/api/boards/{board}/custom-fields", data)
req.Header = headers
client := &http.Client{}
resp, err := client.Do(req)
// ...
}
'multipart/form-data',
'Accept' => 'application/json',
'Authorization' => 'API_KEY',
);
$client = new \GuzzleHttp\Client();
// Define array of request body.
$request_body = array();
try {
$response = $client->request('POST','/api/boards/{board}/custom-fields', array(
'headers' => $headers,
'json' => $request_body,
)
);
print_r($response->getBody()->getContents());
}
catch (\GuzzleHttp\Exception\BadResponseException $e) {
// handle exception or api errors.
print_r($e->getMessage());
}
// ...
POST /api/boards/{board}/custom-fields
Create a Custom Field
Body parameter
name: string
type: string
settings: string
showOnCard: true
automaticallyOnCard: true
showLabelOnMiniCard: true
showSumAtTopOfList: true
authorId: string
Parameters
Name | In | Type | Required | Description |
---|---|---|---|---|
board | path | string | true | the board value |
body | body | object | true | none |
» name | body | string | true | the name of the custom field |
» type | body | string | true | the type of the custom field |
» settings | body | string | true | the settings object of the custom field |
» showOnCard | body | boolean | true | should we show the custom field on cards? |
» automaticallyOnCard | body | boolean | true | should the custom fields automatically be added on cards? |
» showLabelOnMiniCard | body | boolean | true | should the label of the custom field be shown on minicards? |
» showSumAtTopOfList | body | boolean | true | should the sum of the custom fields be shown at top of list? |
» authorId | body | string | true | the authorId value |
Example responses
200 Response
{
"_id": "string"
}
Responses
Status | Meaning | Description | Schema |
---|---|---|---|
200 | OK | 200 response | Inline |
Response Schema
Status Code 200
Name | Type | Required | Restrictions | Description |
---|---|---|---|---|
» _id | string | false | none | none |
get_custom_field
Code samples
# You can also use wget
curl -X GET /api/boards/{board}/custom-fields/{customField} \
-H 'Accept: application/json' \
-H 'Authorization: API_KEY'
GET /api/boards/{board}/custom-fields/{customField} HTTP/1.1
Accept: application/json
const headers = {
'Accept':'application/json',
'Authorization':'API_KEY'
};
fetch('/api/boards/{board}/custom-fields/{customField}',
{
method: 'GET',
headers: headers
})
.then(function(res) {
return res.json();
}).then(function(body) {
console.log(body);
});
const fetch = require('node-fetch');
const headers = {
'Accept':'application/json',
'Authorization':'API_KEY'
};
fetch('/api/boards/{board}/custom-fields/{customField}',
{
method: 'GET',
headers: headers
})
.then(function(res) {
return res.json();
}).then(function(body) {
console.log(body);
});
require 'rest-client'
require 'json'
headers = {
'Accept' => 'application/json',
'Authorization' => 'API_KEY'
}
result = RestClient.get '/api/boards/{board}/custom-fields/{customField}',
params: {
}, headers: headers
p JSON.parse(result)
import requests
headers = {
'Accept': 'application/json',
'Authorization': 'API_KEY'
}
r = requests.get('/api/boards/{board}/custom-fields/{customField}', headers = headers)
print(r.json())
URL obj = new URL("/api/boards/{board}/custom-fields/{customField}");
HttpURLConnection con = (HttpURLConnection) obj.openConnection();
con.setRequestMethod("GET");
int responseCode = con.getResponseCode();
BufferedReader in = new BufferedReader(
new InputStreamReader(con.getInputStream()));
String inputLine;
StringBuffer response = new StringBuffer();
while ((inputLine = in.readLine()) != null) {
response.append(inputLine);
}
in.close();
System.out.println(response.toString());
package main
import (
"bytes"
"net/http"
)
func main() {
headers := map[string][]string{
"Accept": []string{"application/json"},
"Authorization": []string{"API_KEY"},
}
data := bytes.NewBuffer([]byte{jsonReq})
req, err := http.NewRequest("GET", "/api/boards/{board}/custom-fields/{customField}", data)
req.Header = headers
client := &http.Client{}
resp, err := client.Do(req)
// ...
}
'application/json',
'Authorization' => 'API_KEY',
);
$client = new \GuzzleHttp\Client();
// Define array of request body.
$request_body = array();
try {
$response = $client->request('GET','/api/boards/{board}/custom-fields/{customField}', array(
'headers' => $headers,
'json' => $request_body,
)
);
print_r($response->getBody()->getContents());
}
catch (\GuzzleHttp\Exception\BadResponseException $e) {
// handle exception or api errors.
print_r($e->getMessage());
}
// ...
GET /api/boards/{board}/custom-fields/{customField}
Get a Custom Fields attached to a board
Parameters
Name | In | Type | Required | Description |
---|---|---|---|---|
board | path | string | true | the board value |
customField | path | string | true | the ID of the custom field |
Detailed descriptions
customField: the ID of the custom field
Example responses
200 Response
[
{
"_id": "string",
"boardIds": "string"
}
]
Responses
Status | Meaning | Description | Schema |
---|---|---|---|
200 | OK | 200 response | Inline |
Response Schema
Status Code 200
Name | Type | Required | Restrictions | Description |
---|---|---|---|---|
» _id | string | false | none | none |
» boardIds | string | false | none | none |
edit_custom_field
Code samples
# You can also use wget
curl -X PUT /api/boards/{board}/custom-fields/{customField} \
-H 'Content-Type: multipart/form-data' \
-H 'Accept: application/json' \
-H 'Authorization: API_KEY'
PUT /api/boards/{board}/custom-fields/{customField} HTTP/1.1
Content-Type: multipart/form-data
Accept: application/json
const inputBody = '{
"name": "string",
"type": "string",
"settings": "string",
"showOnCard": true,
"automaticallyOnCard": true,
"alwaysOnCard": "string",
"showLabelOnMiniCard": true,
"showSumAtTopOfList": true
}';
const headers = {
'Content-Type':'multipart/form-data',
'Accept':'application/json',
'Authorization':'API_KEY'
};
fetch('/api/boards/{board}/custom-fields/{customField}',
{
method: 'PUT',
body: inputBody,
headers: headers
})
.then(function(res) {
return res.json();
}).then(function(body) {
console.log(body);
});
const fetch = require('node-fetch');
const inputBody = {
"name": "string",
"type": "string",
"settings": "string",
"showOnCard": true,
"automaticallyOnCard": true,
"alwaysOnCard": "string",
"showLabelOnMiniCard": true,
"showSumAtTopOfList": true
};
const headers = {
'Content-Type':'multipart/form-data',
'Accept':'application/json',
'Authorization':'API_KEY'
};
fetch('/api/boards/{board}/custom-fields/{customField}',
{
method: 'PUT',
body: JSON.stringify(inputBody),
headers: headers
})
.then(function(res) {
return res.json();
}).then(function(body) {
console.log(body);
});
require 'rest-client'
require 'json'
headers = {
'Content-Type' => 'multipart/form-data',
'Accept' => 'application/json',
'Authorization' => 'API_KEY'
}
result = RestClient.put '/api/boards/{board}/custom-fields/{customField}',
params: {
}, headers: headers
p JSON.parse(result)
import requests
headers = {
'Content-Type': 'multipart/form-data',
'Accept': 'application/json',
'Authorization': 'API_KEY'
}
r = requests.put('/api/boards/{board}/custom-fields/{customField}', headers = headers)
print(r.json())
URL obj = new URL("/api/boards/{board}/custom-fields/{customField}");
HttpURLConnection con = (HttpURLConnection) obj.openConnection();
con.setRequestMethod("PUT");
int responseCode = con.getResponseCode();
BufferedReader in = new BufferedReader(
new InputStreamReader(con.getInputStream()));
String inputLine;
StringBuffer response = new StringBuffer();
while ((inputLine = in.readLine()) != null) {
response.append(inputLine);
}
in.close();
System.out.println(response.toString());
package main
import (
"bytes"
"net/http"
)
func main() {
headers := map[string][]string{
"Content-Type": []string{"multipart/form-data"},
"Accept": []string{"application/json"},
"Authorization": []string{"API_KEY"},
}
data := bytes.NewBuffer([]byte{jsonReq})
req, err := http.NewRequest("PUT", "/api/boards/{board}/custom-fields/{customField}", data)
req.Header = headers
client := &http.Client{}
resp, err := client.Do(req)
// ...
}
'multipart/form-data',
'Accept' => 'application/json',
'Authorization' => 'API_KEY',
);
$client = new \GuzzleHttp\Client();
// Define array of request body.
$request_body = array();
try {
$response = $client->request('PUT','/api/boards/{board}/custom-fields/{customField}', array(
'headers' => $headers,
'json' => $request_body,
)
);
print_r($response->getBody()->getContents());
}
catch (\GuzzleHttp\Exception\BadResponseException $e) {
// handle exception or api errors.
print_r($e->getMessage());
}
// ...
PUT /api/boards/{board}/custom-fields/{customField}
Update a Custom Field
Body parameter
name: string
type: string
settings: string
showOnCard: true
automaticallyOnCard: true
alwaysOnCard: string
showLabelOnMiniCard: true
showSumAtTopOfList: true
Parameters
Name | In | Type | Required | Description |
---|---|---|---|---|
board | path | string | true | the board value |
customField | path | string | true | the customField value |
body | body | object | true | none |
» name | body | string | true | the name of the custom field |
» type | body | string | true | the type of the custom field |
» settings | body | string | true | the settings object of the custom field |
» showOnCard | body | boolean | true | should we show the custom field on cards |
» automaticallyOnCard | body | boolean | true | should the custom fields automatically be added on cards |
» alwaysOnCard | body | string | true | the alwaysOnCard value |
» showLabelOnMiniCard | body | boolean | true | should the label of the custom field be shown on minicards |
» showSumAtTopOfList | body | boolean | true | should the sum of the custom fields be shown at top of list |
Example responses
200 Response
{
"_id": "string"
}
Responses
Status | Meaning | Description | Schema |
---|---|---|---|
200 | OK | 200 response | Inline |
Response Schema
Status Code 200
Name | Type | Required | Restrictions | Description |
---|---|---|---|---|
» _id | string | false | none | none |
delete_custom_field
Code samples
# You can also use wget
curl -X DELETE /api/boards/{board}/custom-fields/{customField} \
-H 'Accept: application/json' \
-H 'Authorization: API_KEY'
DELETE /api/boards/{board}/custom-fields/{customField} HTTP/1.1
Accept: application/json
const headers = {
'Accept':'application/json',
'Authorization':'API_KEY'
};
fetch('/api/boards/{board}/custom-fields/{customField}',
{
method: 'DELETE',
headers: headers
})
.then(function(res) {
return res.json();
}).then(function(body) {
console.log(body);
});
const fetch = require('node-fetch');
const headers = {
'Accept':'application/json',
'Authorization':'API_KEY'
};
fetch('/api/boards/{board}/custom-fields/{customField}',
{
method: 'DELETE',
headers: headers
})
.then(function(res) {
return res.json();
}).then(function(body) {
console.log(body);
});
require 'rest-client'
require 'json'
headers = {
'Accept' => 'application/json',
'Authorization' => 'API_KEY'
}
result = RestClient.delete '/api/boards/{board}/custom-fields/{customField}',
params: {
}, headers: headers
p JSON.parse(result)
import requests
headers = {
'Accept': 'application/json',
'Authorization': 'API_KEY'
}
r = requests.delete('/api/boards/{board}/custom-fields/{customField}', headers = headers)
print(r.json())
URL obj = new URL("/api/boards/{board}/custom-fields/{customField}");
HttpURLConnection con = (HttpURLConnection) obj.openConnection();
con.setRequestMethod("DELETE");
int responseCode = con.getResponseCode();
BufferedReader in = new BufferedReader(
new InputStreamReader(con.getInputStream()));
String inputLine;
StringBuffer response = new StringBuffer();
while ((inputLine = in.readLine()) != null) {
response.append(inputLine);
}
in.close();
System.out.println(response.toString());
package main
import (
"bytes"
"net/http"
)
func main() {
headers := map[string][]string{
"Accept": []string{"application/json"},
"Authorization": []string{"API_KEY"},
}
data := bytes.NewBuffer([]byte{jsonReq})
req, err := http.NewRequest("DELETE", "/api/boards/{board}/custom-fields/{customField}", data)
req.Header = headers
client := &http.Client{}
resp, err := client.Do(req)
// ...
}
'application/json',
'Authorization' => 'API_KEY',
);
$client = new \GuzzleHttp\Client();
// Define array of request body.
$request_body = array();
try {
$response = $client->request('DELETE','/api/boards/{board}/custom-fields/{customField}', array(
'headers' => $headers,
'json' => $request_body,
)
);
print_r($response->getBody()->getContents());
}
catch (\GuzzleHttp\Exception\BadResponseException $e) {
// handle exception or api errors.
print_r($e->getMessage());
}
// ...
DELETE /api/boards/{board}/custom-fields/{customField}
Delete a Custom Fields attached to a board
The Custom Field can't be retrieved after this operation
Parameters
Name | In | Type | Required | Description |
---|---|---|---|---|
board | path | string | true | the board value |
customField | path | string | true | the ID of the custom field |
Detailed descriptions
customField: the ID of the custom field
Example responses
200 Response
{
"_id": "string"
}
Responses
Status | Meaning | Description | Schema |
---|---|---|---|
200 | OK | 200 response | Inline |
Response Schema
Status Code 200
Name | Type | Required | Restrictions | Description |
---|---|---|---|---|
» _id | string | false | none | none |
add_custom_field_dropdown_items
Code samples
# You can also use wget
curl -X POST /api/boards/{board}/custom-fields/{customField}/dropdown-items \
-H 'Content-Type: multipart/form-data' \
-H 'Accept: application/json' \
-H 'Authorization: API_KEY'
POST /api/boards/{board}/custom-fields/{customField}/dropdown-items HTTP/1.1
Content-Type: multipart/form-data
Accept: application/json
const inputBody = '{
"items": "string"
}';
const headers = {
'Content-Type':'multipart/form-data',
'Accept':'application/json',
'Authorization':'API_KEY'
};
fetch('/api/boards/{board}/custom-fields/{customField}/dropdown-items',
{
method: 'POST',
body: inputBody,
headers: headers
})
.then(function(res) {
return res.json();
}).then(function(body) {
console.log(body);
});
const fetch = require('node-fetch');
const inputBody = {
"items": "string"
};
const headers = {
'Content-Type':'multipart/form-data',
'Accept':'application/json',
'Authorization':'API_KEY'
};
fetch('/api/boards/{board}/custom-fields/{customField}/dropdown-items',
{
method: 'POST',
body: JSON.stringify(inputBody),
headers: headers
})
.then(function(res) {
return res.json();
}).then(function(body) {
console.log(body);
});
require 'rest-client'
require 'json'
headers = {
'Content-Type' => 'multipart/form-data',
'Accept' => 'application/json',
'Authorization' => 'API_KEY'
}
result = RestClient.post '/api/boards/{board}/custom-fields/{customField}/dropdown-items',
params: {
}, headers: headers
p JSON.parse(result)
import requests
headers = {
'Content-Type': 'multipart/form-data',
'Accept': 'application/json',
'Authorization': 'API_KEY'
}
r = requests.post('/api/boards/{board}/custom-fields/{customField}/dropdown-items', headers = headers)
print(r.json())
URL obj = new URL("/api/boards/{board}/custom-fields/{customField}/dropdown-items");
HttpURLConnection con = (HttpURLConnection) obj.openConnection();
con.setRequestMethod("POST");
int responseCode = con.getResponseCode();
BufferedReader in = new BufferedReader(
new InputStreamReader(con.getInputStream()));
String inputLine;
StringBuffer response = new StringBuffer();
while ((inputLine = in.readLine()) != null) {
response.append(inputLine);
}
in.close();
System.out.println(response.toString());
package main
import (
"bytes"
"net/http"
)
func main() {
headers := map[string][]string{
"Content-Type": []string{"multipart/form-data"},
"Accept": []string{"application/json"},
"Authorization": []string{"API_KEY"},
}
data := bytes.NewBuffer([]byte{jsonReq})
req, err := http.NewRequest("POST", "/api/boards/{board}/custom-fields/{customField}/dropdown-items", data)
req.Header = headers
client := &http.Client{}
resp, err := client.Do(req)
// ...
}
'multipart/form-data',
'Accept' => 'application/json',
'Authorization' => 'API_KEY',
);
$client = new \GuzzleHttp\Client();
// Define array of request body.
$request_body = array();
try {
$response = $client->request('POST','/api/boards/{board}/custom-fields/{customField}/dropdown-items', array(
'headers' => $headers,
'json' => $request_body,
)
);
print_r($response->getBody()->getContents());
}
catch (\GuzzleHttp\Exception\BadResponseException $e) {
// handle exception or api errors.
print_r($e->getMessage());
}
// ...
POST /api/boards/{board}/custom-fields/{customField}/dropdown-items
Update a Custom Field's dropdown items
Body parameter
items: string
Parameters
Name | In | Type | Required | Description |
---|---|---|---|---|
board | path | string | true | the board value |
customField | path | string | true | the customField value |
body | body | object | false | none |
» items | body | string | false | names of the custom field |
Example responses
200 Response
{
"_id": "string"
}
Responses
Status | Meaning | Description | Schema |
---|---|---|---|
200 | OK | 200 response | Inline |
Response Schema
Status Code 200
Name | Type | Required | Restrictions | Description |
---|---|---|---|---|
» _id | string | false | none | none |
edit_custom_field_dropdown_item
Code samples
# You can also use wget
curl -X PUT /api/boards/{board}/custom-fields/{customField}/dropdown-items/{dropdownItem} \
-H 'Content-Type: multipart/form-data' \
-H 'Accept: application/json' \
-H 'Authorization: API_KEY'
PUT /api/boards/{board}/custom-fields/{customField}/dropdown-items/{dropdownItem} HTTP/1.1
Content-Type: multipart/form-data
Accept: application/json
const inputBody = '{
"name": "string"
}';
const headers = {
'Content-Type':'multipart/form-data',
'Accept':'application/json',
'Authorization':'API_KEY'
};
fetch('/api/boards/{board}/custom-fields/{customField}/dropdown-items/{dropdownItem}',
{
method: 'PUT',
body: inputBody,
headers: headers
})
.then(function(res) {
return res.json();
}).then(function(body) {
console.log(body);
});
const fetch = require('node-fetch');
const inputBody = {
"name": "string"
};
const headers = {
'Content-Type':'multipart/form-data',
'Accept':'application/json',
'Authorization':'API_KEY'
};
fetch('/api/boards/{board}/custom-fields/{customField}/dropdown-items/{dropdownItem}',
{
method: 'PUT',
body: JSON.stringify(inputBody),
headers: headers
})
.then(function(res) {
return res.json();
}).then(function(body) {
console.log(body);
});
require 'rest-client'
require 'json'
headers = {
'Content-Type' => 'multipart/form-data',
'Accept' => 'application/json',
'Authorization' => 'API_KEY'
}
result = RestClient.put '/api/boards/{board}/custom-fields/{customField}/dropdown-items/{dropdownItem}',
params: {
}, headers: headers
p JSON.parse(result)
import requests
headers = {
'Content-Type': 'multipart/form-data',
'Accept': 'application/json',
'Authorization': 'API_KEY'
}
r = requests.put('/api/boards/{board}/custom-fields/{customField}/dropdown-items/{dropdownItem}', headers = headers)
print(r.json())
URL obj = new URL("/api/boards/{board}/custom-fields/{customField}/dropdown-items/{dropdownItem}");
HttpURLConnection con = (HttpURLConnection) obj.openConnection();
con.setRequestMethod("PUT");
int responseCode = con.getResponseCode();
BufferedReader in = new BufferedReader(
new InputStreamReader(con.getInputStream()));
String inputLine;
StringBuffer response = new StringBuffer();
while ((inputLine = in.readLine()) != null) {
response.append(inputLine);
}
in.close();
System.out.println(response.toString());
package main
import (
"bytes"
"net/http"
)
func main() {
headers := map[string][]string{
"Content-Type": []string{"multipart/form-data"},
"Accept": []string{"application/json"},
"Authorization": []string{"API_KEY"},
}
data := bytes.NewBuffer([]byte{jsonReq})
req, err := http.NewRequest("PUT", "/api/boards/{board}/custom-fields/{customField}/dropdown-items/{dropdownItem}", data)
req.Header = headers
client := &http.Client{}
resp, err := client.Do(req)
// ...
}
'multipart/form-data',
'Accept' => 'application/json',
'Authorization' => 'API_KEY',
);
$client = new \GuzzleHttp\Client();
// Define array of request body.
$request_body = array();
try {
$response = $client->request('PUT','/api/boards/{board}/custom-fields/{customField}/dropdown-items/{dropdownItem}', array(
'headers' => $headers,
'json' => $request_body,
)
);
print_r($response->getBody()->getContents());
}
catch (\GuzzleHttp\Exception\BadResponseException $e) {
// handle exception or api errors.
print_r($e->getMessage());
}
// ...
PUT /api/boards/{board}/custom-fields/{customField}/dropdown-items/{dropdownItem}
Update a Custom Field's dropdown item
Body parameter
name: string
Parameters
Name | In | Type | Required | Description |
---|---|---|---|---|
board | path | string | true | the board value |
customField | path | string | true | the customField value |
dropdownItem | path | string | true | the dropdownItem value |
body | body | object | true | none |
» name | body | string | true | names of the custom field |
Example responses
200 Response
{
"_id": "string"
}
Responses
Status | Meaning | Description | Schema |
---|---|---|---|
200 | OK | 200 response | Inline |
Response Schema
Status Code 200
Name | Type | Required | Restrictions | Description |
---|---|---|---|---|
» _id | string | false | none | none |
delete_custom_field_dropdown_item
Code samples
# You can also use wget
curl -X DELETE /api/boards/{board}/custom-fields/{customField}/dropdown-items/{dropdownItem} \
-H 'Accept: application/json' \
-H 'Authorization: API_KEY'
DELETE /api/boards/{board}/custom-fields/{customField}/dropdown-items/{dropdownItem} HTTP/1.1
Accept: application/json
const headers = {
'Accept':'application/json',
'Authorization':'API_KEY'
};
fetch('/api/boards/{board}/custom-fields/{customField}/dropdown-items/{dropdownItem}',
{
method: 'DELETE',
headers: headers
})
.then(function(res) {
return res.json();
}).then(function(body) {
console.log(body);
});
const fetch = require('node-fetch');
const headers = {
'Accept':'application/json',
'Authorization':'API_KEY'
};
fetch('/api/boards/{board}/custom-fields/{customField}/dropdown-items/{dropdownItem}',
{
method: 'DELETE',
headers: headers
})
.then(function(res) {
return res.json();
}).then(function(body) {
console.log(body);
});
require 'rest-client'
require 'json'
headers = {
'Accept' => 'application/json',
'Authorization' => 'API_KEY'
}
result = RestClient.delete '/api/boards/{board}/custom-fields/{customField}/dropdown-items/{dropdownItem}',
params: {
}, headers: headers
p JSON.parse(result)
import requests
headers = {
'Accept': 'application/json',
'Authorization': 'API_KEY'
}
r = requests.delete('/api/boards/{board}/custom-fields/{customField}/dropdown-items/{dropdownItem}', headers = headers)
print(r.json())
URL obj = new URL("/api/boards/{board}/custom-fields/{customField}/dropdown-items/{dropdownItem}");
HttpURLConnection con = (HttpURLConnection) obj.openConnection();
con.setRequestMethod("DELETE");
int responseCode = con.getResponseCode();
BufferedReader in = new BufferedReader(
new InputStreamReader(con.getInputStream()));
String inputLine;
StringBuffer response = new StringBuffer();
while ((inputLine = in.readLine()) != null) {
response.append(inputLine);
}
in.close();
System.out.println(response.toString());
package main
import (
"bytes"
"net/http"
)
func main() {
headers := map[string][]string{
"Accept": []string{"application/json"},
"Authorization": []string{"API_KEY"},
}
data := bytes.NewBuffer([]byte{jsonReq})
req, err := http.NewRequest("DELETE", "/api/boards/{board}/custom-fields/{customField}/dropdown-items/{dropdownItem}", data)
req.Header = headers
client := &http.Client{}
resp, err := client.Do(req)
// ...
}
'application/json',
'Authorization' => 'API_KEY',
);
$client = new \GuzzleHttp\Client();
// Define array of request body.
$request_body = array();
try {
$response = $client->request('DELETE','/api/boards/{board}/custom-fields/{customField}/dropdown-items/{dropdownItem}', array(
'headers' => $headers,
'json' => $request_body,
)
);
print_r($response->getBody()->getContents());
}
catch (\GuzzleHttp\Exception\BadResponseException $e) {
// handle exception or api errors.
print_r($e->getMessage());
}
// ...
DELETE /api/boards/{board}/custom-fields/{customField}/dropdown-items/{dropdownItem}
Update a Custom Field's dropdown items
Parameters
Name | In | Type | Required | Description |
---|---|---|---|---|
board | path | string | true | the board value |
customField | path | string | true | the customField value |
dropdownItem | path | string | true | the dropdownItem value |
Example responses
200 Response
{
"_id": "string"
}
Responses
Status | Meaning | Description | Schema |
---|---|---|---|
200 | OK | 200 response | Inline |
Response Schema
Status Code 200
Name | Type | Required | Restrictions | Description |
---|---|---|---|---|
» _id | string | false | none | none |
Integrations
get_all_integrations
Code samples
# You can also use wget
curl -X GET /api/boards/{board}/integrations \
-H 'Accept: application/json' \
-H 'Authorization: API_KEY'
GET /api/boards/{board}/integrations HTTP/1.1
Accept: application/json
const headers = {
'Accept':'application/json',
'Authorization':'API_KEY'
};
fetch('/api/boards/{board}/integrations',
{
method: 'GET',
headers: headers
})
.then(function(res) {
return res.json();
}).then(function(body) {
console.log(body);
});
const fetch = require('node-fetch');
const headers = {
'Accept':'application/json',
'Authorization':'API_KEY'
};
fetch('/api/boards/{board}/integrations',
{
method: 'GET',
headers: headers
})
.then(function(res) {
return res.json();
}).then(function(body) {
console.log(body);
});
require 'rest-client'
require 'json'
headers = {
'Accept' => 'application/json',
'Authorization' => 'API_KEY'
}
result = RestClient.get '/api/boards/{board}/integrations',
params: {
}, headers: headers
p JSON.parse(result)
import requests
headers = {
'Accept': 'application/json',
'Authorization': 'API_KEY'
}
r = requests.get('/api/boards/{board}/integrations', headers = headers)
print(r.json())
URL obj = new URL("/api/boards/{board}/integrations");
HttpURLConnection con = (HttpURLConnection) obj.openConnection();
con.setRequestMethod("GET");
int responseCode = con.getResponseCode();
BufferedReader in = new BufferedReader(
new InputStreamReader(con.getInputStream()));
String inputLine;
StringBuffer response = new StringBuffer();
while ((inputLine = in.readLine()) != null) {
response.append(inputLine);
}
in.close();
System.out.println(response.toString());
package main
import (
"bytes"
"net/http"
)
func main() {
headers := map[string][]string{
"Accept": []string{"application/json"},
"Authorization": []string{"API_KEY"},
}
data := bytes.NewBuffer([]byte{jsonReq})
req, err := http.NewRequest("GET", "/api/boards/{board}/integrations", data)
req.Header = headers
client := &http.Client{}
resp, err := client.Do(req)
// ...
}
'application/json',
'Authorization' => 'API_KEY',
);
$client = new \GuzzleHttp\Client();
// Define array of request body.
$request_body = array();
try {
$response = $client->request('GET','/api/boards/{board}/integrations', array(
'headers' => $headers,
'json' => $request_body,
)
);
print_r($response->getBody()->getContents());
}
catch (\GuzzleHttp\Exception\BadResponseException $e) {
// handle exception or api errors.
print_r($e->getMessage());
}
// ...
GET /api/boards/{board}/integrations
Get all integrations in board
Parameters
Name | In | Type | Required | Description |
---|---|---|---|---|
board | path | string | true | the board ID |
Detailed descriptions
board: the board ID
Example responses
200 Response
[
{
"enabled": true,
"title": "string",
"type": "string",
"activities": [
"string"
],
"url": "string",
"token": "string",
"boardId": "string",
"createdAt": "string",
"modifiedAt": "string",
"userId": "string"
}
]
Responses
Status | Meaning | Description | Schema |
---|---|---|---|
200 | OK | 200 response | Inline |
Response Schema
Status Code 200
Name | Type | Required | Restrictions | Description |
---|---|---|---|---|
anonymous | [Integrations] | false | none | [Integration with third-party applications] |
» enabled | boolean | true | none | is the integration enabled? |
» title | string¦null | false | none | name of the integration |
» type | string | true | none | type of the integratation (Default to 'outgoing-webhooks') |
» activities | [string] | true | none | activities the integration gets triggered (list) |
» url | string | true | none | none |
» token | string¦null | false | none | token of the integration |
» boardId | string | true | none | Board ID of the integration |
» createdAt | string | true | none | Creation date of the integration |
» modifiedAt | string | true | none | none |
» userId | string | true | none | user ID who created the interation |
new_integration
Code samples
# You can also use wget
curl -X POST /api/boards/{board}/integrations \
-H 'Content-Type: multipart/form-data' \
-H 'Accept: application/json' \
-H 'Authorization: API_KEY'
POST /api/boards/{board}/integrations HTTP/1.1
Content-Type: multipart/form-data
Accept: application/json
const inputBody = '{
"url": "string"
}';
const headers = {
'Content-Type':'multipart/form-data',
'Accept':'application/json',
'Authorization':'API_KEY'
};
fetch('/api/boards/{board}/integrations',
{
method: 'POST',
body: inputBody,
headers: headers
})
.then(function(res) {
return res.json();
}).then(function(body) {
console.log(body);
});
const fetch = require('node-fetch');
const inputBody = {
"url": "string"
};
const headers = {
'Content-Type':'multipart/form-data',
'Accept':'application/json',
'Authorization':'API_KEY'
};
fetch('/api/boards/{board}/integrations',
{
method: 'POST',
body: JSON.stringify(inputBody),
headers: headers
})
.then(function(res) {
return res.json();
}).then(function(body) {
console.log(body);
});
require 'rest-client'
require 'json'
headers = {
'Content-Type' => 'multipart/form-data',
'Accept' => 'application/json',
'Authorization' => 'API_KEY'
}
result = RestClient.post '/api/boards/{board}/integrations',
params: {
}, headers: headers
p JSON.parse(result)
import requests
headers = {
'Content-Type': 'multipart/form-data',
'Accept': 'application/json',
'Authorization': 'API_KEY'
}
r = requests.post('/api/boards/{board}/integrations', headers = headers)
print(r.json())
URL obj = new URL("/api/boards/{board}/integrations");
HttpURLConnection con = (HttpURLConnection) obj.openConnection();
con.setRequestMethod("POST");
int responseCode = con.getResponseCode();
BufferedReader in = new BufferedReader(
new InputStreamReader(con.getInputStream()));
String inputLine;
StringBuffer response = new StringBuffer();
while ((inputLine = in.readLine()) != null) {
response.append(inputLine);
}
in.close();
System.out.println(response.toString());
package main
import (
"bytes"
"net/http"
)
func main() {
headers := map[string][]string{
"Content-Type": []string{"multipart/form-data"},
"Accept": []string{"application/json"},
"Authorization": []string{"API_KEY"},
}
data := bytes.NewBuffer([]byte{jsonReq})
req, err := http.NewRequest("POST", "/api/boards/{board}/integrations", data)
req.Header = headers
client := &http.Client{}
resp, err := client.Do(req)
// ...
}
'multipart/form-data',
'Accept' => 'application/json',
'Authorization' => 'API_KEY',
);
$client = new \GuzzleHttp\Client();
// Define array of request body.
$request_body = array();
try {
$response = $client->request('POST','/api/boards/{board}/integrations', array(
'headers' => $headers,
'json' => $request_body,
)
);
print_r($response->getBody()->getContents());
}
catch (\GuzzleHttp\Exception\BadResponseException $e) {
// handle exception or api errors.
print_r($e->getMessage());
}
// ...
POST /api/boards/{board}/integrations
Create a new integration
Body parameter
url: string
Parameters
Name | In | Type | Required | Description |
---|---|---|---|---|
board | path | string | true | the board ID |
body | body | object | true | none |
» url | body | string | true | the URL of the integration |
Detailed descriptions
board: the board ID
Example responses
200 Response
{
"_id": "string"
}
Responses
Status | Meaning | Description | Schema |
---|---|---|---|
200 | OK | 200 response | Inline |
Response Schema
Status Code 200
Name | Type | Required | Restrictions | Description |
---|---|---|---|---|
» _id | string | false | none | none |
get_integration
Code samples
# You can also use wget
curl -X GET /api/boards/{board}/integrations/{int} \
-H 'Accept: application/json' \
-H 'Authorization: API_KEY'
GET /api/boards/{board}/integrations/{int} HTTP/1.1
Accept: application/json
const headers = {
'Accept':'application/json',
'Authorization':'API_KEY'
};
fetch('/api/boards/{board}/integrations/{int}',
{
method: 'GET',
headers: headers
})
.then(function(res) {
return res.json();
}).then(function(body) {
console.log(body);
});
const fetch = require('node-fetch');
const headers = {
'Accept':'application/json',
'Authorization':'API_KEY'
};
fetch('/api/boards/{board}/integrations/{int}',
{
method: 'GET',
headers: headers
})
.then(function(res) {
return res.json();
}).then(function(body) {
console.log(body);
});
require 'rest-client'
require 'json'
headers = {
'Accept' => 'application/json',
'Authorization' => 'API_KEY'
}
result = RestClient.get '/api/boards/{board}/integrations/{int}',
params: {
}, headers: headers
p JSON.parse(result)
import requests
headers = {
'Accept': 'application/json',
'Authorization': 'API_KEY'
}
r = requests.get('/api/boards/{board}/integrations/{int}', headers = headers)
print(r.json())
URL obj = new URL("/api/boards/{board}/integrations/{int}");
HttpURLConnection con = (HttpURLConnection) obj.openConnection();
con.setRequestMethod("GET");
int responseCode = con.getResponseCode();
BufferedReader in = new BufferedReader(
new InputStreamReader(con.getInputStream()));
String inputLine;
StringBuffer response = new StringBuffer();
while ((inputLine = in.readLine()) != null) {
response.append(inputLine);
}
in.close();
System.out.println(response.toString());
package main
import (
"bytes"
"net/http"
)
func main() {
headers := map[string][]string{
"Accept": []string{"application/json"},
"Authorization": []string{"API_KEY"},
}
data := bytes.NewBuffer([]byte{jsonReq})
req, err := http.NewRequest("GET", "/api/boards/{board}/integrations/{int}", data)
req.Header = headers
client := &http.Client{}
resp, err := client.Do(req)
// ...
}
'application/json',
'Authorization' => 'API_KEY',
);
$client = new \GuzzleHttp\Client();
// Define array of request body.
$request_body = array();
try {
$response = $client->request('GET','/api/boards/{board}/integrations/{int}', array(
'headers' => $headers,
'json' => $request_body,
)
);
print_r($response->getBody()->getContents());
}
catch (\GuzzleHttp\Exception\BadResponseException $e) {
// handle exception or api errors.
print_r($e->getMessage());
}
// ...
GET /api/boards/{board}/integrations/{int}
Get a single integration in board
Parameters
Name | In | Type | Required | Description |
---|---|---|---|---|
board | path | string | true | the board ID |
int | path | string | true | the integration ID |
Detailed descriptions
board: the board ID
int: the integration ID
Example responses
200 Response
{
"enabled": true,
"title": "string",
"type": "string",
"activities": [
"string"
],
"url": "string",
"token": "string",
"boardId": "string",
"createdAt": "string",
"modifiedAt": "string",
"userId": "string"
}
Responses
Status | Meaning | Description | Schema |
---|---|---|---|
200 | OK | 200 response | Integrations |
edit_integration
Code samples
# You can also use wget
curl -X PUT /api/boards/{board}/integrations/{int} \
-H 'Content-Type: multipart/form-data' \
-H 'Accept: application/json' \
-H 'Authorization: API_KEY'
PUT /api/boards/{board}/integrations/{int} HTTP/1.1
Content-Type: multipart/form-data
Accept: application/json
const inputBody = '{
"enabled": "string",
"title": "string",
"url": "string",
"token": "string",
"activities": "string"
}';
const headers = {
'Content-Type':'multipart/form-data',
'Accept':'application/json',
'Authorization':'API_KEY'
};
fetch('/api/boards/{board}/integrations/{int}',
{
method: 'PUT',
body: inputBody,
headers: headers
})
.then(function(res) {
return res.json();
}).then(function(body) {
console.log(body);
});
const fetch = require('node-fetch');
const inputBody = {
"enabled": "string",
"title": "string",
"url": "string",
"token": "string",
"activities": "string"
};
const headers = {
'Content-Type':'multipart/form-data',
'Accept':'application/json',
'Authorization':'API_KEY'
};
fetch('/api/boards/{board}/integrations/{int}',
{
method: 'PUT',
body: JSON.stringify(inputBody),
headers: headers
})
.then(function(res) {
return res.json();
}).then(function(body) {
console.log(body);
});
require 'rest-client'
require 'json'
headers = {
'Content-Type' => 'multipart/form-data',
'Accept' => 'application/json',
'Authorization' => 'API_KEY'
}
result = RestClient.put '/api/boards/{board}/integrations/{int}',
params: {
}, headers: headers
p JSON.parse(result)
import requests
headers = {
'Content-Type': 'multipart/form-data',
'Accept': 'application/json',
'Authorization': 'API_KEY'
}
r = requests.put('/api/boards/{board}/integrations/{int}', headers = headers)
print(r.json())
URL obj = new URL("/api/boards/{board}/integrations/{int}");
HttpURLConnection con = (HttpURLConnection) obj.openConnection();
con.setRequestMethod("PUT");
int responseCode = con.getResponseCode();
BufferedReader in = new BufferedReader(
new InputStreamReader(con.getInputStream()));
String inputLine;
StringBuffer response = new StringBuffer();
while ((inputLine = in.readLine()) != null) {
response.append(inputLine);
}
in.close();
System.out.println(response.toString());
package main
import (
"bytes"
"net/http"
)
func main() {
headers := map[string][]string{
"Content-Type": []string{"multipart/form-data"},
"Accept": []string{"application/json"},
"Authorization": []string{"API_KEY"},
}
data := bytes.NewBuffer([]byte{jsonReq})
req, err := http.NewRequest("PUT", "/api/boards/{board}/integrations/{int}", data)
req.Header = headers
client := &http.Client{}
resp, err := client.Do(req)
// ...
}
'multipart/form-data',
'Accept' => 'application/json',
'Authorization' => 'API_KEY',
);
$client = new \GuzzleHttp\Client();
// Define array of request body.
$request_body = array();
try {
$response = $client->request('PUT','/api/boards/{board}/integrations/{int}', array(
'headers' => $headers,
'json' => $request_body,
)
);
print_r($response->getBody()->getContents());
}
catch (\GuzzleHttp\Exception\BadResponseException $e) {
// handle exception or api errors.
print_r($e->getMessage());
}
// ...
PUT /api/boards/{board}/integrations/{int}
Edit integration data
Body parameter
enabled: string
title: string
url: string
token: string
activities: string
Parameters
Name | In | Type | Required | Description |
---|---|---|---|---|
board | path | string | true | the board ID |
int | path | string | true | the integration ID |
body | body | object | false | none |
» enabled | body | string | false | is the integration enabled? |
» title | body | string | false | new name of the integration |
» url | body | string | false | new URL of the integration |
» token | body | string | false | new token of the integration |
» activities | body | string | false | new list of activities of the integration |
Detailed descriptions
board: the board ID
int: the integration ID
Example responses
200 Response
{
"_id": "string"
}
Responses
Status | Meaning | Description | Schema |
---|---|---|---|
200 | OK | 200 response | Inline |
Response Schema
Status Code 200
Name | Type | Required | Restrictions | Description |
---|---|---|---|---|
» _id | string | false | none | none |
delete_integration
Code samples
# You can also use wget
curl -X DELETE /api/boards/{board}/integrations/{int} \
-H 'Accept: application/json' \
-H 'Authorization: API_KEY'
DELETE /api/boards/{board}/integrations/{int} HTTP/1.1
Accept: application/json
const headers = {
'Accept':'application/json',
'Authorization':'API_KEY'
};
fetch('/api/boards/{board}/integrations/{int}',
{
method: 'DELETE',
headers: headers
})
.then(function(res) {
return res.json();
}).then(function(body) {
console.log(body);
});
const fetch = require('node-fetch');
const headers = {
'Accept':'application/json',
'Authorization':'API_KEY'
};
fetch('/api/boards/{board}/integrations/{int}',
{
method: 'DELETE',
headers: headers
})
.then(function(res) {
return res.json();
}).then(function(body) {
console.log(body);
});
require 'rest-client'
require 'json'
headers = {
'Accept' => 'application/json',
'Authorization' => 'API_KEY'
}
result = RestClient.delete '/api/boards/{board}/integrations/{int}',
params: {
}, headers: headers
p JSON.parse(result)
import requests
headers = {
'Accept': 'application/json',
'Authorization': 'API_KEY'
}
r = requests.delete('/api/boards/{board}/integrations/{int}', headers = headers)
print(r.json())
URL obj = new URL("/api/boards/{board}/integrations/{int}");
HttpURLConnection con = (HttpURLConnection) obj.openConnection();
con.setRequestMethod("DELETE");
int responseCode = con.getResponseCode();
BufferedReader in = new BufferedReader(
new InputStreamReader(con.getInputStream()));
String inputLine;
StringBuffer response = new StringBuffer();
while ((inputLine = in.readLine()) != null) {
response.append(inputLine);
}
in.close();
System.out.println(response.toString());
package main
import (
"bytes"
"net/http"
)
func main() {
headers := map[string][]string{
"Accept": []string{"application/json"},
"Authorization": []string{"API_KEY"},
}
data := bytes.NewBuffer([]byte{jsonReq})
req, err := http.NewRequest("DELETE", "/api/boards/{board}/integrations/{int}", data)
req.Header = headers
client := &http.Client{}
resp, err := client.Do(req)
// ...
}
'application/json',
'Authorization' => 'API_KEY',
);
$client = new \GuzzleHttp\Client();
// Define array of request body.
$request_body = array();
try {
$response = $client->request('DELETE','/api/boards/{board}/integrations/{int}', array(
'headers' => $headers,
'json' => $request_body,
)
);
print_r($response->getBody()->getContents());
}
catch (\GuzzleHttp\Exception\BadResponseException $e) {
// handle exception or api errors.
print_r($e->getMessage());
}
// ...
DELETE /api/boards/{board}/integrations/{int}
Delete integration
Parameters
Name | In | Type | Required | Description |
---|---|---|---|---|
board | path | string | true | the board ID |
int | path | string | true | the integration ID |
Detailed descriptions
board: the board ID
int: the integration ID
Example responses
200 Response
{
"_id": "string"
}
Responses
Status | Meaning | Description | Schema |
---|---|---|---|
200 | OK | 200 response | Inline |
Response Schema
Status Code 200
Name | Type | Required | Restrictions | Description |
---|---|---|---|---|
» _id | string | false | none | none |
delete_integration_activities
Code samples
# You can also use wget
curl -X DELETE /api/boards/{board}/integrations/{int}/activities \
-H 'Accept: application/json' \
-H 'Authorization: API_KEY'
DELETE /api/boards/{board}/integrations/{int}/activities HTTP/1.1
Accept: application/json
const headers = {
'Accept':'application/json',
'Authorization':'API_KEY'
};
fetch('/api/boards/{board}/integrations/{int}/activities',
{
method: 'DELETE',
headers: headers
})
.then(function(res) {
return res.json();
}).then(function(body) {
console.log(body);
});
const fetch = require('node-fetch');
const headers = {
'Accept':'application/json',
'Authorization':'API_KEY'
};
fetch('/api/boards/{board}/integrations/{int}/activities',
{
method: 'DELETE',
headers: headers
})
.then(function(res) {
return res.json();
}).then(function(body) {
console.log(body);
});
require 'rest-client'
require 'json'
headers = {
'Accept' => 'application/json',
'Authorization' => 'API_KEY'
}
result = RestClient.delete '/api/boards/{board}/integrations/{int}/activities',
params: {
}, headers: headers
p JSON.parse(result)
import requests
headers = {
'Accept': 'application/json',
'Authorization': 'API_KEY'
}
r = requests.delete('/api/boards/{board}/integrations/{int}/activities', headers = headers)
print(r.json())
URL obj = new URL("/api/boards/{board}/integrations/{int}/activities");
HttpURLConnection con = (HttpURLConnection) obj.openConnection();
con.setRequestMethod("DELETE");
int responseCode = con.getResponseCode();
BufferedReader in = new BufferedReader(
new InputStreamReader(con.getInputStream()));
String inputLine;
StringBuffer response = new StringBuffer();
while ((inputLine = in.readLine()) != null) {
response.append(inputLine);
}
in.close();
System.out.println(response.toString());
package main
import (
"bytes"
"net/http"
)
func main() {
headers := map[string][]string{
"Accept": []string{"application/json"},
"Authorization": []string{"API_KEY"},
}
data := bytes.NewBuffer([]byte{jsonReq})
req, err := http.NewRequest("DELETE", "/api/boards/{board}/integrations/{int}/activities", data)
req.Header = headers
client := &http.Client{}
resp, err := client.Do(req)
// ...
}
'application/json',
'Authorization' => 'API_KEY',
);
$client = new \GuzzleHttp\Client();
// Define array of request body.
$request_body = array();
try {
$response = $client->request('DELETE','/api/boards/{board}/integrations/{int}/activities', array(
'headers' => $headers,
'json' => $request_body,
)
);
print_r($response->getBody()->getContents());
}
catch (\GuzzleHttp\Exception\BadResponseException $e) {
// handle exception or api errors.
print_r($e->getMessage());
}
// ...
DELETE /api/boards/{board}/integrations/{int}/activities
Delete subscribed activities
Parameters
Name | In | Type | Required | Description |
---|---|---|---|---|
board | path | string | true | the board ID |
int | path | string | true | the integration ID |
Detailed descriptions
board: the board ID
int: the integration ID
Example responses
200 Response
{
"enabled": true,
"title": "string",
"type": "string",
"activities": [
"string"
],
"url": "string",
"token": "string",
"boardId": "string",
"createdAt": "string",
"modifiedAt": "string",
"userId": "string"
}
Responses
Status | Meaning | Description | Schema |
---|---|---|---|
200 | OK | 200 response | Integrations |
new_integration_activities
Code samples
# You can also use wget
curl -X POST /api/boards/{board}/integrations/{int}/activities \
-H 'Content-Type: multipart/form-data' \
-H 'Accept: application/json' \
-H 'Authorization: API_KEY'
POST /api/boards/{board}/integrations/{int}/activities HTTP/1.1
Content-Type: multipart/form-data
Accept: application/json
const inputBody = '{
"activities": "string"
}';
const headers = {
'Content-Type':'multipart/form-data',
'Accept':'application/json',
'Authorization':'API_KEY'
};
fetch('/api/boards/{board}/integrations/{int}/activities',
{
method: 'POST',
body: inputBody,
headers: headers
})
.then(function(res) {
return res.json();
}).then(function(body) {
console.log(body);
});
const fetch = require('node-fetch');
const inputBody = {
"activities": "string"
};
const headers = {
'Content-Type':'multipart/form-data',
'Accept':'application/json',
'Authorization':'API_KEY'
};
fetch('/api/boards/{board}/integrations/{int}/activities',
{
method: 'POST',
body: JSON.stringify(inputBody),
headers: headers
})
.then(function(res) {
return res.json();
}).then(function(body) {
console.log(body);
});
require 'rest-client'
require 'json'
headers = {
'Content-Type' => 'multipart/form-data',
'Accept' => 'application/json',
'Authorization' => 'API_KEY'
}
result = RestClient.post '/api/boards/{board}/integrations/{int}/activities',
params: {
}, headers: headers
p JSON.parse(result)
import requests
headers = {
'Content-Type': 'multipart/form-data',
'Accept': 'application/json',
'Authorization': 'API_KEY'
}
r = requests.post('/api/boards/{board}/integrations/{int}/activities', headers = headers)
print(r.json())
URL obj = new URL("/api/boards/{board}/integrations/{int}/activities");
HttpURLConnection con = (HttpURLConnection) obj.openConnection();
con.setRequestMethod("POST");
int responseCode = con.getResponseCode();
BufferedReader in = new BufferedReader(
new InputStreamReader(con.getInputStream()));
String inputLine;
StringBuffer response = new StringBuffer();
while ((inputLine = in.readLine()) != null) {
response.append(inputLine);
}
in.close();
System.out.println(response.toString());
package main
import (
"bytes"
"net/http"
)
func main() {
headers := map[string][]string{
"Content-Type": []string{"multipart/form-data"},
"Accept": []string{"application/json"},
"Authorization": []string{"API_KEY"},
}
data := bytes.NewBuffer([]byte{jsonReq})
req, err := http.NewRequest("POST", "/api/boards/{board}/integrations/{int}/activities", data)
req.Header = headers
client := &http.Client{}
resp, err := client.Do(req)
// ...
}
'multipart/form-data',
'Accept' => 'application/json',
'Authorization' => 'API_KEY',
);
$client = new \GuzzleHttp\Client();
// Define array of request body.
$request_body = array();
try {
$response = $client->request('POST','/api/boards/{board}/integrations/{int}/activities', array(
'headers' => $headers,
'json' => $request_body,
)
);
print_r($response->getBody()->getContents());
}
catch (\GuzzleHttp\Exception\BadResponseException $e) {
// handle exception or api errors.
print_r($e->getMessage());
}
// ...
POST /api/boards/{board}/integrations/{int}/activities
Add subscribed activities
Body parameter
activities: string
Parameters
Name | In | Type | Required | Description |
---|---|---|---|---|
board | path | string | true | the board ID |
int | path | string | true | the integration ID |
body | body | object | true | none |
» activities | body | string | true | the activities value |
Detailed descriptions
board: the board ID
int: the integration ID
Example responses
200 Response
{
"enabled": true,
"title": "string",
"type": "string",
"activities": [
"string"
],
"url": "string",
"token": "string",
"boardId": "string",
"createdAt": "string",
"modifiedAt": "string",
"userId": "string"
}
Responses
Status | Meaning | Description | Schema |
---|---|---|---|
200 | OK | 200 response | Integrations |
Lists
get_all_lists
Code samples
# You can also use wget
curl -X GET /api/boards/{board}/lists \
-H 'Accept: application/json' \
-H 'Authorization: API_KEY'
GET /api/boards/{board}/lists HTTP/1.1
Accept: application/json
const headers = {
'Accept':'application/json',
'Authorization':'API_KEY'
};
fetch('/api/boards/{board}/lists',
{
method: 'GET',
headers: headers
})
.then(function(res) {
return res.json();
}).then(function(body) {
console.log(body);
});
const fetch = require('node-fetch');
const headers = {
'Accept':'application/json',
'Authorization':'API_KEY'
};
fetch('/api/boards/{board}/lists',
{
method: 'GET',
headers: headers
})
.then(function(res) {
return res.json();
}).then(function(body) {
console.log(body);
});
require 'rest-client'
require 'json'
headers = {
'Accept' => 'application/json',
'Authorization' => 'API_KEY'
}
result = RestClient.get '/api/boards/{board}/lists',
params: {
}, headers: headers
p JSON.parse(result)
import requests
headers = {
'Accept': 'application/json',
'Authorization': 'API_KEY'
}
r = requests.get('/api/boards/{board}/lists', headers = headers)
print(r.json())
URL obj = new URL("/api/boards/{board}/lists");
HttpURLConnection con = (HttpURLConnection) obj.openConnection();
con.setRequestMethod("GET");
int responseCode = con.getResponseCode();
BufferedReader in = new BufferedReader(
new InputStreamReader(con.getInputStream()));
String inputLine;
StringBuffer response = new StringBuffer();
while ((inputLine = in.readLine()) != null) {
response.append(inputLine);
}
in.close();
System.out.println(response.toString());
package main
import (
"bytes"
"net/http"
)
func main() {
headers := map[string][]string{
"Accept": []string{"application/json"},
"Authorization": []string{"API_KEY"},
}
data := bytes.NewBuffer([]byte{jsonReq})
req, err := http.NewRequest("GET", "/api/boards/{board}/lists", data)
req.Header = headers
client := &http.Client{}
resp, err := client.Do(req)
// ...
}
'application/json',
'Authorization' => 'API_KEY',
);
$client = new \GuzzleHttp\Client();
// Define array of request body.
$request_body = array();
try {
$response = $client->request('GET','/api/boards/{board}/lists', array(
'headers' => $headers,
'json' => $request_body,
)
);
print_r($response->getBody()->getContents());
}
catch (\GuzzleHttp\Exception\BadResponseException $e) {
// handle exception or api errors.
print_r($e->getMessage());
}
// ...
GET /api/boards/{board}/lists
Get the list of Lists attached to a board
Parameters
Name | In | Type | Required | Description |
---|---|---|---|---|
board | path | string | true | the board ID |
Detailed descriptions
board: the board ID
Example responses
200 Response
[
{
"_id": "string",
"title": "string"
}
]
Responses
Status | Meaning | Description | Schema |
---|---|---|---|
200 | OK | 200 response | Inline |
Response Schema
Status Code 200
Name | Type | Required | Restrictions | Description |
---|---|---|---|---|
» _id | string | false | none | none |
» title | string | false | none | none |
new_list
Code samples
# You can also use wget
curl -X POST /api/boards/{board}/lists \
-H 'Content-Type: multipart/form-data' \
-H 'Accept: application/json' \
-H 'Authorization: API_KEY'
POST /api/boards/{board}/lists HTTP/1.1
Content-Type: multipart/form-data
Accept: application/json
const inputBody = '{
"title": "string"
}';
const headers = {
'Content-Type':'multipart/form-data',
'Accept':'application/json',
'Authorization':'API_KEY'
};
fetch('/api/boards/{board}/lists',
{
method: 'POST',
body: inputBody,
headers: headers
})
.then(function(res) {
return res.json();
}).then(function(body) {
console.log(body);
});
const fetch = require('node-fetch');
const inputBody = {
"title": "string"
};
const headers = {
'Content-Type':'multipart/form-data',
'Accept':'application/json',
'Authorization':'API_KEY'
};
fetch('/api/boards/{board}/lists',
{
method: 'POST',
body: JSON.stringify(inputBody),
headers: headers
})
.then(function(res) {
return res.json();
}).then(function(body) {
console.log(body);
});
require 'rest-client'
require 'json'
headers = {
'Content-Type' => 'multipart/form-data',
'Accept' => 'application/json',
'Authorization' => 'API_KEY'
}
result = RestClient.post '/api/boards/{board}/lists',
params: {
}, headers: headers
p JSON.parse(result)
import requests
headers = {
'Content-Type': 'multipart/form-data',
'Accept': 'application/json',
'Authorization': 'API_KEY'
}
r = requests.post('/api/boards/{board}/lists', headers = headers)
print(r.json())
URL obj = new URL("/api/boards/{board}/lists");
HttpURLConnection con = (HttpURLConnection) obj.openConnection();
con.setRequestMethod("POST");
int responseCode = con.getResponseCode();
BufferedReader in = new BufferedReader(
new InputStreamReader(con.getInputStream()));
String inputLine;
StringBuffer response = new StringBuffer();
while ((inputLine = in.readLine()) != null) {
response.append(inputLine);
}
in.close();
System.out.println(response.toString());
package main
import (
"bytes"
"net/http"
)
func main() {
headers := map[string][]string{
"Content-Type": []string{"multipart/form-data"},
"Accept": []string{"application/json"},
"Authorization": []string{"API_KEY"},
}
data := bytes.NewBuffer([]byte{jsonReq})
req, err := http.NewRequest("POST", "/api/boards/{board}/lists", data)
req.Header = headers
client := &http.Client{}
resp, err := client.Do(req)
// ...
}
'multipart/form-data',
'Accept' => 'application/json',
'Authorization' => 'API_KEY',
);
$client = new \GuzzleHttp\Client();
// Define array of request body.
$request_body = array();
try {
$response = $client->request('POST','/api/boards/{board}/lists', array(
'headers' => $headers,
'json' => $request_body,
)
);
print_r($response->getBody()->getContents());
}
catch (\GuzzleHttp\Exception\BadResponseException $e) {
// handle exception or api errors.
print_r($e->getMessage());
}
// ...
POST /api/boards/{board}/lists
Add a List to a board
Body parameter
title: string
Parameters
Name | In | Type | Required | Description |
---|---|---|---|---|
board | path | string | true | the board ID |
body | body | object | true | none |
» title | body | string | true | the title of the List |
Detailed descriptions
board: the board ID
Example responses
200 Response
{
"_id": "string"
}
Responses
Status | Meaning | Description | Schema |
---|---|---|---|
200 | OK | 200 response | Inline |
Response Schema
Status Code 200
Name | Type | Required | Restrictions | Description |
---|---|---|---|---|
» _id | string | false | none | none |
get_list
Code samples
# You can also use wget
curl -X GET /api/boards/{board}/lists/{list} \
-H 'Accept: application/json' \
-H 'Authorization: API_KEY'
GET /api/boards/{board}/lists/{list} HTTP/1.1
Accept: application/json
const headers = {
'Accept':'application/json',
'Authorization':'API_KEY'
};
fetch('/api/boards/{board}/lists/{list}',
{
method: 'GET',
headers: headers
})
.then(function(res) {
return res.json();
}).then(function(body) {
console.log(body);
});
const fetch = require('node-fetch');
const headers = {
'Accept':'application/json',
'Authorization':'API_KEY'
};
fetch('/api/boards/{board}/lists/{list}',
{
method: 'GET',
headers: headers
})
.then(function(res) {
return res.json();
}).then(function(body) {
console.log(body);
});
require 'rest-client'
require 'json'
headers = {
'Accept' => 'application/json',
'Authorization' => 'API_KEY'
}
result = RestClient.get '/api/boards/{board}/lists/{list}',
params: {
}, headers: headers
p JSON.parse(result)
import requests
headers = {
'Accept': 'application/json',
'Authorization': 'API_KEY'
}
r = requests.get('/api/boards/{board}/lists/{list}', headers = headers)
print(r.json())
URL obj = new URL("/api/boards/{board}/lists/{list}");
HttpURLConnection con = (HttpURLConnection) obj.openConnection();
con.setRequestMethod("GET");
int responseCode = con.getResponseCode();
BufferedReader in = new BufferedReader(
new InputStreamReader(con.getInputStream()));
String inputLine;
StringBuffer response = new StringBuffer();
while ((inputLine = in.readLine()) != null) {
response.append(inputLine);
}
in.close();
System.out.println(response.toString());
package main
import (
"bytes"
"net/http"
)
func main() {
headers := map[string][]string{
"Accept": []string{"application/json"},
"Authorization": []string{"API_KEY"},
}
data := bytes.NewBuffer([]byte{jsonReq})
req, err := http.NewRequest("GET", "/api/boards/{board}/lists/{list}", data)
req.Header = headers
client := &http.Client{}
resp, err := client.Do(req)
// ...
}
'application/json',
'Authorization' => 'API_KEY',
);
$client = new \GuzzleHttp\Client();
// Define array of request body.
$request_body = array();
try {
$response = $client->request('GET','/api/boards/{board}/lists/{list}', array(
'headers' => $headers,
'json' => $request_body,
)
);
print_r($response->getBody()->getContents());
}
catch (\GuzzleHttp\Exception\BadResponseException $e) {
// handle exception or api errors.
print_r($e->getMessage());
}
// ...
GET /api/boards/{board}/lists/{list}
Get a List attached to a board
Parameters
Name | In | Type | Required | Description |
---|---|---|---|---|
board | path | string | true | the board ID |
list | path | string | true | the List ID |
Detailed descriptions
board: the board ID
list: the List ID
Example responses
200 Response
{
"title": "string",
"starred": true,
"archived": true,
"archivedAt": "string",
"boardId": "string",
"swimlaneId": "string",
"createdAt": "string",
"sort": 0,
"updatedAt": "string",
"modifiedAt": "string",
"wipLimit": {
"value": 0,
"enabled": true,
"soft": true
},
"color": "white",
"type": "string",
"collapsed": true
}
Responses
Status | Meaning | Description | Schema |
---|---|---|---|
200 | OK | 200 response | Lists |
delete_list
Code samples
# You can also use wget
curl -X DELETE /api/boards/{board}/lists/{list} \
-H 'Accept: application/json' \
-H 'Authorization: API_KEY'
DELETE /api/boards/{board}/lists/{list} HTTP/1.1
Accept: application/json
const headers = {
'Accept':'application/json',
'Authorization':'API_KEY'
};
fetch('/api/boards/{board}/lists/{list}',
{
method: 'DELETE',
headers: headers
})
.then(function(res) {
return res.json();
}).then(function(body) {
console.log(body);
});
const fetch = require('node-fetch');
const headers = {
'Accept':'application/json',
'Authorization':'API_KEY'
};
fetch('/api/boards/{board}/lists/{list}',
{
method: 'DELETE',
headers: headers
})
.then(function(res) {
return res.json();
}).then(function(body) {
console.log(body);
});
require 'rest-client'
require 'json'
headers = {
'Accept' => 'application/json',
'Authorization' => 'API_KEY'
}
result = RestClient.delete '/api/boards/{board}/lists/{list}',
params: {
}, headers: headers
p JSON.parse(result)
import requests
headers = {
'Accept': 'application/json',
'Authorization': 'API_KEY'
}
r = requests.delete('/api/boards/{board}/lists/{list}', headers = headers)
print(r.json())
URL obj = new URL("/api/boards/{board}/lists/{list}");
HttpURLConnection con = (HttpURLConnection) obj.openConnection();
con.setRequestMethod("DELETE");
int responseCode = con.getResponseCode();
BufferedReader in = new BufferedReader(
new InputStreamReader(con.getInputStream()));
String inputLine;
StringBuffer response = new StringBuffer();
while ((inputLine = in.readLine()) != null) {
response.append(inputLine);
}
in.close();
System.out.println(response.toString());
package main
import (
"bytes"
"net/http"
)
func main() {
headers := map[string][]string{
"Accept": []string{"application/json"},
"Authorization": []string{"API_KEY"},
}
data := bytes.NewBuffer([]byte{jsonReq})
req, err := http.NewRequest("DELETE", "/api/boards/{board}/lists/{list}", data)
req.Header = headers
client := &http.Client{}
resp, err := client.Do(req)
// ...
}
'application/json',
'Authorization' => 'API_KEY',
);
$client = new \GuzzleHttp\Client();
// Define array of request body.
$request_body = array();
try {
$response = $client->request('DELETE','/api/boards/{board}/lists/{list}', array(
'headers' => $headers,
'json' => $request_body,
)
);
print_r($response->getBody()->getContents());
}
catch (\GuzzleHttp\Exception\BadResponseException $e) {
// handle exception or api errors.
print_r($e->getMessage());
}
// ...
DELETE /api/boards/{board}/lists/{list}
Delete a List
This deletes a list from a board. The list is not put in the recycle bin.
Parameters
Name | In | Type | Required | Description |
---|---|---|---|---|
board | path | string | true | the board ID |
list | path | string | true | the ID of the list to remove |
Detailed descriptions
board: the board ID
list: the ID of the list to remove
Example responses
200 Response
{
"_id": "string"
}
Responses
Status | Meaning | Description | Schema |
---|---|---|---|
200 | OK | 200 response | Inline |
Response Schema
Status Code 200
Name | Type | Required | Restrictions | Description |
---|---|---|---|---|
» _id | string | false | none | none |
Swimlanes
get_all_swimlanes
Code samples
# You can also use wget
curl -X GET /api/boards/{board}/swimlanes \
-H 'Accept: application/json' \
-H 'Authorization: API_KEY'
GET /api/boards/{board}/swimlanes HTTP/1.1
Accept: application/json
const headers = {
'Accept':'application/json',
'Authorization':'API_KEY'
};
fetch('/api/boards/{board}/swimlanes',
{
method: 'GET',
headers: headers
})
.then(function(res) {
return res.json();
}).then(function(body) {
console.log(body);
});
const fetch = require('node-fetch');
const headers = {
'Accept':'application/json',
'Authorization':'API_KEY'
};
fetch('/api/boards/{board}/swimlanes',
{
method: 'GET',
headers: headers
})
.then(function(res) {
return res.json();
}).then(function(body) {
console.log(body);
});
require 'rest-client'
require 'json'
headers = {
'Accept' => 'application/json',
'Authorization' => 'API_KEY'
}
result = RestClient.get '/api/boards/{board}/swimlanes',
params: {
}, headers: headers
p JSON.parse(result)
import requests
headers = {
'Accept': 'application/json',
'Authorization': 'API_KEY'
}
r = requests.get('/api/boards/{board}/swimlanes', headers = headers)
print(r.json())
URL obj = new URL("/api/boards/{board}/swimlanes");
HttpURLConnection con = (HttpURLConnection) obj.openConnection();
con.setRequestMethod("GET");
int responseCode = con.getResponseCode();
BufferedReader in = new BufferedReader(
new InputStreamReader(con.getInputStream()));
String inputLine;
StringBuffer response = new StringBuffer();
while ((inputLine = in.readLine()) != null) {
response.append(inputLine);
}
in.close();
System.out.println(response.toString());
package main
import (
"bytes"
"net/http"
)
func main() {
headers := map[string][]string{
"Accept": []string{"application/json"},
"Authorization": []string{"API_KEY"},
}
data := bytes.NewBuffer([]byte{jsonReq})
req, err := http.NewRequest("GET", "/api/boards/{board}/swimlanes", data)
req.Header = headers
client := &http.Client{}
resp, err := client.Do(req)
// ...
}
'application/json',
'Authorization' => 'API_KEY',
);
$client = new \GuzzleHttp\Client();
// Define array of request body.
$request_body = array();
try {
$response = $client->request('GET','/api/boards/{board}/swimlanes', array(
'headers' => $headers,
'json' => $request_body,
)
);
print_r($response->getBody()->getContents());
}
catch (\GuzzleHttp\Exception\BadResponseException $e) {
// handle exception or api errors.
print_r($e->getMessage());
}
// ...
GET /api/boards/{board}/swimlanes
Get the list of swimlanes attached to a board
Parameters
Name | In | Type | Required | Description |
---|---|---|---|---|
board | path | string | true | the ID of the board |
Detailed descriptions
board: the ID of the board
Example responses
200 Response
[
{
"_id": "string",
"title": "string"
}
]
Responses
Status | Meaning | Description | Schema |
---|---|---|---|
200 | OK | 200 response | Inline |
Response Schema
Status Code 200
Name | Type | Required | Restrictions | Description |
---|---|---|---|---|
» _id | string | false | none | none |
» title | string | false | none | none |
new_swimlane
Code samples
# You can also use wget
curl -X POST /api/boards/{board}/swimlanes \
-H 'Content-Type: multipart/form-data' \
-H 'Accept: application/json' \
-H 'Authorization: API_KEY'
POST /api/boards/{board}/swimlanes HTTP/1.1
Content-Type: multipart/form-data
Accept: application/json
const inputBody = '{
"title": "string"
}';
const headers = {
'Content-Type':'multipart/form-data',
'Accept':'application/json',
'Authorization':'API_KEY'
};
fetch('/api/boards/{board}/swimlanes',
{
method: 'POST',
body: inputBody,
headers: headers
})
.then(function(res) {
return res.json();
}).then(function(body) {
console.log(body);
});
const fetch = require('node-fetch');
const inputBody = {
"title": "string"
};
const headers = {
'Content-Type':'multipart/form-data',
'Accept':'application/json',
'Authorization':'API_KEY'
};
fetch('/api/boards/{board}/swimlanes',
{
method: 'POST',
body: JSON.stringify(inputBody),
headers: headers
})
.then(function(res) {
return res.json();
}).then(function(body) {
console.log(body);
});
require 'rest-client'
require 'json'
headers = {
'Content-Type' => 'multipart/form-data',
'Accept' => 'application/json',
'Authorization' => 'API_KEY'
}
result = RestClient.post '/api/boards/{board}/swimlanes',
params: {
}, headers: headers
p JSON.parse(result)
import requests
headers = {
'Content-Type': 'multipart/form-data',
'Accept': 'application/json',
'Authorization': 'API_KEY'
}
r = requests.post('/api/boards/{board}/swimlanes', headers = headers)
print(r.json())
URL obj = new URL("/api/boards/{board}/swimlanes");
HttpURLConnection con = (HttpURLConnection) obj.openConnection();
con.setRequestMethod("POST");
int responseCode = con.getResponseCode();
BufferedReader in = new BufferedReader(
new InputStreamReader(con.getInputStream()));
String inputLine;
StringBuffer response = new StringBuffer();
while ((inputLine = in.readLine()) != null) {
response.append(inputLine);
}
in.close();
System.out.println(response.toString());
package main
import (
"bytes"
"net/http"
)
func main() {
headers := map[string][]string{
"Content-Type": []string{"multipart/form-data"},
"Accept": []string{"application/json"},
"Authorization": []string{"API_KEY"},
}
data := bytes.NewBuffer([]byte{jsonReq})
req, err := http.NewRequest("POST", "/api/boards/{board}/swimlanes", data)
req.Header = headers
client := &http.Client{}
resp, err := client.Do(req)
// ...
}
'multipart/form-data',
'Accept' => 'application/json',
'Authorization' => 'API_KEY',
);
$client = new \GuzzleHttp\Client();
// Define array of request body.
$request_body = array();
try {
$response = $client->request('POST','/api/boards/{board}/swimlanes', array(
'headers' => $headers,
'json' => $request_body,
)
);
print_r($response->getBody()->getContents());
}
catch (\GuzzleHttp\Exception\BadResponseException $e) {
// handle exception or api errors.
print_r($e->getMessage());
}
// ...
POST /api/boards/{board}/swimlanes
Add a swimlane to a board
Body parameter
title: string
Parameters
Name | In | Type | Required | Description |
---|---|---|---|---|
board | path | string | true | the ID of the board |
body | body | object | true | none |
» title | body | string | true | the new title of the swimlane |
Detailed descriptions
board: the ID of the board
Example responses
200 Response
{
"_id": "string"
}
Responses
Status | Meaning | Description | Schema |
---|---|---|---|
200 | OK | 200 response | Inline |
Response Schema
Status Code 200
Name | Type | Required | Restrictions | Description |
---|---|---|---|---|
» _id | string | false | none | none |
get_swimlane
Code samples
# You can also use wget
curl -X GET /api/boards/{board}/swimlanes/{swimlane} \
-H 'Accept: application/json' \
-H 'Authorization: API_KEY'
GET /api/boards/{board}/swimlanes/{swimlane} HTTP/1.1
Accept: application/json
const headers = {
'Accept':'application/json',
'Authorization':'API_KEY'
};
fetch('/api/boards/{board}/swimlanes/{swimlane}',
{
method: 'GET',
headers: headers
})
.then(function(res) {
return res.json();
}).then(function(body) {
console.log(body);
});
const fetch = require('node-fetch');
const headers = {
'Accept':'application/json',
'Authorization':'API_KEY'
};
fetch('/api/boards/{board}/swimlanes/{swimlane}',
{
method: 'GET',
headers: headers
})
.then(function(res) {
return res.json();
}).then(function(body) {
console.log(body);
});
require 'rest-client'
require 'json'
headers = {
'Accept' => 'application/json',
'Authorization' => 'API_KEY'
}
result = RestClient.get '/api/boards/{board}/swimlanes/{swimlane}',
params: {
}, headers: headers
p JSON.parse(result)
import requests
headers = {
'Accept': 'application/json',
'Authorization': 'API_KEY'
}
r = requests.get('/api/boards/{board}/swimlanes/{swimlane}', headers = headers)
print(r.json())
URL obj = new URL("/api/boards/{board}/swimlanes/{swimlane}");
HttpURLConnection con = (HttpURLConnection) obj.openConnection();
con.setRequestMethod("GET");
int responseCode = con.getResponseCode();
BufferedReader in = new BufferedReader(
new InputStreamReader(con.getInputStream()));
String inputLine;
StringBuffer response = new StringBuffer();
while ((inputLine = in.readLine()) != null) {
response.append(inputLine);
}
in.close();
System.out.println(response.toString());
package main
import (
"bytes"
"net/http"
)
func main() {
headers := map[string][]string{
"Accept": []string{"application/json"},
"Authorization": []string{"API_KEY"},
}
data := bytes.NewBuffer([]byte{jsonReq})
req, err := http.NewRequest("GET", "/api/boards/{board}/swimlanes/{swimlane}", data)
req.Header = headers
client := &http.Client{}
resp, err := client.Do(req)
// ...
}
'application/json',
'Authorization' => 'API_KEY',
);
$client = new \GuzzleHttp\Client();
// Define array of request body.
$request_body = array();
try {
$response = $client->request('GET','/api/boards/{board}/swimlanes/{swimlane}', array(
'headers' => $headers,
'json' => $request_body,
)
);
print_r($response->getBody()->getContents());
}
catch (\GuzzleHttp\Exception\BadResponseException $e) {
// handle exception or api errors.
print_r($e->getMessage());
}
// ...
GET /api/boards/{board}/swimlanes/{swimlane}
Get a swimlane
Parameters
Name | In | Type | Required | Description |
---|---|---|---|---|
board | path | string | true | the ID of the board |
swimlane | path | string | true | the ID of the swimlane |
Detailed descriptions
board: the ID of the board
swimlane: the ID of the swimlane
Example responses
200 Response
{
"title": "string",
"archived": true,
"archivedAt": "string",
"boardId": "string",
"createdAt": "string",
"sort": 0,
"color": "white",
"updatedAt": "string",
"modifiedAt": "string",
"type": "string",
"collapsed": true
}
Responses
Status | Meaning | Description | Schema |
---|---|---|---|
200 | OK | 200 response | Swimlanes |
edit_swimlane
Code samples
# You can also use wget
curl -X PUT /api/boards/{board}/swimlanes/{swimlane} \
-H 'Content-Type: multipart/form-data' \
-H 'Accept: application/json' \
-H 'Authorization: API_KEY'
PUT /api/boards/{board}/swimlanes/{swimlane} HTTP/1.1
Content-Type: multipart/form-data
Accept: application/json
const inputBody = '{
"title": "string"
}';
const headers = {
'Content-Type':'multipart/form-data',
'Accept':'application/json',
'Authorization':'API_KEY'
};
fetch('/api/boards/{board}/swimlanes/{swimlane}',
{
method: 'PUT',
body: inputBody,
headers: headers
})
.then(function(res) {
return res.json();
}).then(function(body) {
console.log(body);
});
const fetch = require('node-fetch');
const inputBody = {
"title": "string"
};
const headers = {
'Content-Type':'multipart/form-data',
'Accept':'application/json',
'Authorization':'API_KEY'
};
fetch('/api/boards/{board}/swimlanes/{swimlane}',
{
method: 'PUT',
body: JSON.stringify(inputBody),
headers: headers
})
.then(function(res) {
return res.json();
}).then(function(body) {
console.log(body);
});
require 'rest-client'
require 'json'
headers = {
'Content-Type' => 'multipart/form-data',
'Accept' => 'application/json',
'Authorization' => 'API_KEY'
}
result = RestClient.put '/api/boards/{board}/swimlanes/{swimlane}',
params: {
}, headers: headers
p JSON.parse(result)
import requests
headers = {
'Content-Type': 'multipart/form-data',
'Accept': 'application/json',
'Authorization': 'API_KEY'
}
r = requests.put('/api/boards/{board}/swimlanes/{swimlane}', headers = headers)
print(r.json())
URL obj = new URL("/api/boards/{board}/swimlanes/{swimlane}");
HttpURLConnection con = (HttpURLConnection) obj.openConnection();
con.setRequestMethod("PUT");
int responseCode = con.getResponseCode();
BufferedReader in = new BufferedReader(
new InputStreamReader(con.getInputStream()));
String inputLine;
StringBuffer response = new StringBuffer();
while ((inputLine = in.readLine()) != null) {
response.append(inputLine);
}
in.close();
System.out.println(response.toString());
package main
import (
"bytes"
"net/http"
)
func main() {
headers := map[string][]string{
"Content-Type": []string{"multipart/form-data"},
"Accept": []string{"application/json"},
"Authorization": []string{"API_KEY"},
}
data := bytes.NewBuffer([]byte{jsonReq})
req, err := http.NewRequest("PUT", "/api/boards/{board}/swimlanes/{swimlane}", data)
req.Header = headers
client := &http.Client{}
resp, err := client.Do(req)
// ...
}
'multipart/form-data',
'Accept' => 'application/json',
'Authorization' => 'API_KEY',
);
$client = new \GuzzleHttp\Client();
// Define array of request body.
$request_body = array();
try {
$response = $client->request('PUT','/api/boards/{board}/swimlanes/{swimlane}', array(
'headers' => $headers,
'json' => $request_body,
)
);
print_r($response->getBody()->getContents());
}
catch (\GuzzleHttp\Exception\BadResponseException $e) {
// handle exception or api errors.
print_r($e->getMessage());
}
// ...
PUT /api/boards/{board}/swimlanes/{swimlane}
Edit the title of a swimlane
Body parameter
title: string
Parameters
Name | In | Type | Required | Description |
---|---|---|---|---|
board | path | string | true | the ID of the board |
swimlane | path | string | true | the ID of the swimlane to edit |
body | body | object | true | none |
» title | body | string | true | the new title of the swimlane |
Detailed descriptions
board: the ID of the board
swimlane: the ID of the swimlane to edit
Example responses
200 Response
{
"_id": "string"
}
Responses
Status | Meaning | Description | Schema |
---|---|---|---|
200 | OK | 200 response | Inline |
Response Schema
Status Code 200
Name | Type | Required | Restrictions | Description |
---|---|---|---|---|
» _id | string | false | none | none |
delete_swimlane
Code samples
# You can also use wget
curl -X DELETE /api/boards/{board}/swimlanes/{swimlane} \
-H 'Accept: application/json' \
-H 'Authorization: API_KEY'
DELETE /api/boards/{board}/swimlanes/{swimlane} HTTP/1.1
Accept: application/json
const headers = {
'Accept':'application/json',
'Authorization':'API_KEY'
};
fetch('/api/boards/{board}/swimlanes/{swimlane}',
{
method: 'DELETE',
headers: headers
})
.then(function(res) {
return res.json();
}).then(function(body) {
console.log(body);
});
const fetch = require('node-fetch');
const headers = {
'Accept':'application/json',
'Authorization':'API_KEY'
};
fetch('/api/boards/{board}/swimlanes/{swimlane}',
{
method: 'DELETE',
headers: headers
})
.then(function(res) {
return res.json();
}).then(function(body) {
console.log(body);
});
require 'rest-client'
require 'json'
headers = {
'Accept' => 'application/json',
'Authorization' => 'API_KEY'
}
result = RestClient.delete '/api/boards/{board}/swimlanes/{swimlane}',
params: {
}, headers: headers
p JSON.parse(result)
import requests
headers = {
'Accept': 'application/json',
'Authorization': 'API_KEY'
}
r = requests.delete('/api/boards/{board}/swimlanes/{swimlane}', headers = headers)
print(r.json())
URL obj = new URL("/api/boards/{board}/swimlanes/{swimlane}");
HttpURLConnection con = (HttpURLConnection) obj.openConnection();
con.setRequestMethod("DELETE");
int responseCode = con.getResponseCode();
BufferedReader in = new BufferedReader(
new InputStreamReader(con.getInputStream()));
String inputLine;
StringBuffer response = new StringBuffer();
while ((inputLine = in.readLine()) != null) {
response.append(inputLine);
}
in.close();
System.out.println(response.toString());
package main
import (
"bytes"
"net/http"
)
func main() {
headers := map[string][]string{
"Accept": []string{"application/json"},
"Authorization": []string{"API_KEY"},
}
data := bytes.NewBuffer([]byte{jsonReq})
req, err := http.NewRequest("DELETE", "/api/boards/{board}/swimlanes/{swimlane}", data)
req.Header = headers
client := &http.Client{}
resp, err := client.Do(req)
// ...
}
'application/json',
'Authorization' => 'API_KEY',
);
$client = new \GuzzleHttp\Client();
// Define array of request body.
$request_body = array();
try {
$response = $client->request('DELETE','/api/boards/{board}/swimlanes/{swimlane}', array(
'headers' => $headers,
'json' => $request_body,
)
);
print_r($response->getBody()->getContents());
}
catch (\GuzzleHttp\Exception\BadResponseException $e) {
// handle exception or api errors.
print_r($e->getMessage());
}
// ...
DELETE /api/boards/{board}/swimlanes/{swimlane}
Delete a swimlane
The swimlane will be deleted, not moved to the recycle bin
Parameters
Name | In | Type | Required | Description |
---|---|---|---|---|
board | path | string | true | the ID of the board |
swimlane | path | string | true | the ID of the swimlane |
Detailed descriptions
board: the ID of the board
swimlane: the ID of the swimlane
Example responses
200 Response
{
"_id": "string"
}
Responses
Status | Meaning | Description | Schema |
---|---|---|---|
200 | OK | 200 response | Inline |
Response Schema
Status Code 200
Name | Type | Required | Restrictions | Description |
---|---|---|---|---|
» _id | string | false | none | none |
Schemas
Boards
{
"title": "string",
"slug": "string",
"archived": true,
"archivedAt": "string",
"createdAt": "string",
"modifiedAt": "string",
"stars": 0,
"labels": [
{
"_id": "string",
"name": "string",
"color": "white"
}
],
"members": [
{
"userId": "string",
"isAdmin": true,
"isActive": true,
"isNoComments": true,
"isCommentOnly": true,
"isWorker": true
}
],
"permission": "public",
"orgs": [
{
"orgId": "string",
"orgDisplayName": "string",
"isActive": true
}
],
"teams": [
{
"teamId": "string",
"teamDisplayName": "string",
"isActive": true
}
],
"color": "belize",
"backgroundImageURL": "string",
"allowsCardCounterList": true,
"allowsBoardMemberList": true,
"description": "string",
"subtasksDefaultBoardId": "string",
"subtasksDefaultListId": "string",
"dateSettingsDefaultBoardId": "string",
"dateSettingsDefaultListId": "string",
"allowsSubtasks": true,
"allowsAttachments": true,
"allowsChecklists": true,
"allowsComments": true,
"allowsDescriptionTitle": true,
"allowsDescriptionText": true,
"allowsDescriptionTextOnMinicard": true,
"allowsCoverAttachmentOnMinicard": true,
"allowsBadgeAttachmentOnMinicard": true,
"allowsCardSortingByNumberOnMinicard": true,
"allowsCardNumber": true,
"allowsActivities": true,
"allowsLabels": true,
"allowsCreator": true,
"allowsCreatorOnMinicard": true,
"allowsAssignee": true,
"allowsMembers": true,
"allowsRequestedBy": true,
"allowsCardSortingByNumber": true,
"allowsShowLists": true,
"allowsAssignedBy": true,
"allowsReceivedDate": true,
"allowsStartDate": true,
"allowsEndDate": true,
"allowsDueDate": true,
"presentParentTask": "prefix-with-full-path",
"receivedAt": "string",
"startAt": "string",
"dueAt": "string",
"endAt": "string",
"spentTime": 0,
"isOvertime": true,
"type": "board",
"sort": 0
}
This is a Board.
Properties
Name | Type | Required | Restrictions | Description |
---|---|---|---|---|
title | string | true | none | The title of the board |
slug | string | true | none | The title slugified. |
archived | boolean | true | none | Is the board archived? |
archivedAt | string¦null | false | none | Latest archiving time of the board |
createdAt | string | true | none | Creation time of the board |
modifiedAt | string¦null | false | none | Last modification time of the board |
stars | number | true | none | How many stars the board has |
labels | [BoardsLabels]¦null | false | none | List of labels attached to a board |
members | [BoardsMembers] | true | none | List of members of a board |
permission | string | true | none | visibility of the board |
orgs | [BoardsOrgs]¦null | false | none | the list of organizations that a board belongs to |
teams | [BoardsTeams]¦null | false | none | the list of teams that a board belongs to |
color | string | true | none | The color of the board. |
backgroundImageURL | string¦null | false | none | The background image URL of the board. |
allowsCardCounterList | boolean | true | none | Show card counter per list |
allowsBoardMemberList | boolean | true | none | Show board member list |
description | string¦null | false | none | The description of the board |
subtasksDefaultBoardId | string¦null | false | none | The default board ID assigned to subtasks. |
subtasksDefaultListId | string¦null | false | none | The default List ID assigned to subtasks. |
dateSettingsDefaultBoardId | string¦null | false | none | none |
dateSettingsDefaultListId | string¦null | false | none | none |
allowsSubtasks | boolean | true | none | Does the board allows subtasks? |
allowsAttachments | boolean | true | none | Does the board allows attachments? |
allowsChecklists | boolean | true | none | Does the board allows checklists? |
allowsComments | boolean | true | none | Does the board allows comments? |
allowsDescriptionTitle | boolean | true | none | Does the board allows description title? |
allowsDescriptionText | boolean | true | none | Does the board allows description text? |
allowsDescriptionTextOnMinicard | boolean | true | none | Does the board allows description text on minicard? |
allowsCoverAttachmentOnMinicard | boolean | true | none | Does the board allows cover attachment on minicard? |
allowsBadgeAttachmentOnMinicard | boolean | true | none | Does the board allows badge attachment on minicard? |
allowsCardSortingByNumberOnMinicard | boolean | true | none | Does the board allows card sorting by number on minicard? |
allowsCardNumber | boolean | true | none | Does the board allows card numbers? |
allowsActivities | boolean | true | none | Does the board allows comments? |
allowsLabels | boolean | true | none | Does the board allows labels? |
allowsCreator | boolean | true | none | Does the board allow creator? |
allowsCreatorOnMinicard | boolean | true | none | Does the board allow creator? |
allowsAssignee | boolean | true | none | Does the board allows assignee? |
allowsMembers | boolean | true | none | Does the board allows members? |
allowsRequestedBy | boolean | true | none | Does the board allows requested by? |
allowsCardSortingByNumber | boolean | true | none | Does the board allows card sorting by number? |
allowsShowLists | boolean | true | none | Does the board allows show lists on the card? |
allowsAssignedBy | boolean | true | none | Does the board allows requested by? |
allowsReceivedDate | boolean | true | none | Does the board allows received date? |
allowsStartDate | boolean | true | none | Does the board allows start date? |
allowsEndDate | boolean | true | none | Does the board allows end date? |
allowsDueDate | boolean | true | none | Does the board allows due date? |
presentParentTask | string¦null | false | none | Controls how to present the parent task: - prefix-with-full-path : add a prefix with the full path- prefix-with-parent : add a prefisx with the parent name- subtext-with-full-path : add a subtext with the full path- subtext-with-parent : add a subtext with the parent name- no-parent : does not show the parent at all |
receivedAt | string¦null | false | none | Date the card was received |
startAt | string¦null | false | none | Starting date of the board. |
dueAt | string¦null | false | none | Due date of the board. |
endAt | string¦null | false | none | End date of the board. |
spentTime | number¦null | false | none | Time spent in the board. |
isOvertime | boolean¦null | false | none | Is the board overtimed? |
type | string | true | none | The type of board possible values: board, template-board, template-container |
sort | number | true | none | Sort value |
Enumerated Values
Property | Value |
---|---|
permission | public |
permission | private |
color | belize |
color | nephritis |
color | pomegranate |
color | pumpkin |
color | wisteria |
color | moderatepink |
color | strongcyan |
color | limegreen |
color | midnight |
color | dark |
color | relax |
color | corteza |
color | clearblue |
color | natural |
color | modern |
color | moderndark |
color | exodark |
color | cleandark |
color | cleanlight |
presentParentTask | prefix-with-full-path |
presentParentTask | prefix-with-parent |
presentParentTask | subtext-with-full-path |
presentParentTask | subtext-with-parent |
presentParentTask | no-parent |
type | board |
type | template-board |
type | template-container |
BoardsLabels
{
"_id": "string",
"name": "string",
"color": "white"
}
Properties
Name | Type | Required | Restrictions | Description |
---|---|---|---|---|
_id | string | true | none | Unique id of a label |
name | string | false | none | Name of a label |
color | string | true | none | color of a label. Can be amongst green , yellow , orange , red , purple ,blue , sky , lime , pink , black ,silver , peachpuff , crimson , plum , darkgreen ,slateblue , magenta , gold , navy , gray ,saddlebrown , paleturquoise , mistyrose , indigo |
Enumerated Values
Property | Value |
---|---|
color | white |
color | green |
color | yellow |
color | orange |
color | red |
color | purple |
color | blue |
color | sky |
color | lime |
color | pink |
color | black |
color | silver |
color | peachpuff |
color | crimson |
color | plum |
color | darkgreen |
color | slateblue |
color | magenta |
color | gold |
color | navy |
color | gray |
color | saddlebrown |
color | paleturquoise |
color | mistyrose |
color | indigo |
BoardsMembers
{
"userId": "string",
"isAdmin": true,
"isActive": true,
"isNoComments": true,
"isCommentOnly": true,
"isWorker": true
}
Properties
Name | Type | Required | Restrictions | Description |
---|---|---|---|---|
userId | string | true | none | The uniq ID of the member |
isAdmin | boolean | true | none | Is the member an admin of the board? |
isActive | boolean | true | none | Is the member active? |
isNoComments | boolean | false | none | Is the member not allowed to make comments |
isCommentOnly | boolean | false | none | Is the member only allowed to comment on the board |
isWorker | boolean | false | none | Is the member only allowed to move card, assign himself to card and comment |
BoardsOrgs
{
"orgId": "string",
"orgDisplayName": "string",
"isActive": true
}
Properties
Name | Type | Required | Restrictions | Description |
---|---|---|---|---|
orgId | string | true | none | The uniq ID of the organization |
orgDisplayName | string | true | none | The display name of the organization |
isActive | boolean | true | none | Is the organization active? |
BoardsTeams
{
"teamId": "string",
"teamDisplayName": "string",
"isActive": true
}
Properties
Name | Type | Required | Restrictions | Description |
---|---|---|---|---|
teamId | string | true | none | The uniq ID of the team |
teamDisplayName | string | true | none | The display name of the team |
isActive | boolean | true | none | Is the team active? |
CardComments
{
"boardId": "string",
"cardId": "string",
"text": "string",
"createdAt": "string",
"modifiedAt": "string",
"userId": "string"
}
A comment on a card
Properties
Name | Type | Required | Restrictions | Description |
---|---|---|---|---|
boardId | string | true | none | the board ID |
cardId | string | true | none | the card ID |
text | string | true | none | the text of the comment |
createdAt | string | true | none | when was the comment created |
modifiedAt | string | true | none | none |
userId | string | true | none | the author ID of the comment |
Cards
{
"title": "string",
"archived": true,
"archivedAt": "string",
"parentId": "string",
"listId": "string",
"swimlaneId": "string",
"boardId": "string",
"coverId": "string",
"color": "white",
"createdAt": "string",
"modifiedAt": "string",
"customFields": [
{}
],
"dateLastActivity": "string",
"description": "string",
"requestedBy": "string",
"assignedBy": "string",
"labelIds": [
"string"
],
"members": [
"string"
],
"assignees": [
"string"
],
"receivedAt": "string",
"startAt": "string",
"dueAt": "string",
"endAt": "string",
"spentTime": 0,
"isOvertime": true,
"userId": "string",
"sort": 0,
"subtaskSort": 0,
"type": "string",
"linkedId": "string",
"vote": {
"question": "string",
"positive": [
"string"
],
"negative": [
"string"
],
"end": "string",
"public": true,
"allowNonBoardMembers": true
},
"poker": {
"question": true,
"one": [
"string"
],
"two": [
"string"
],
"three": [
"string"
],
"five": [
"string"
],
"eight": [
"string"
],
"thirteen": [
"string"
],
"twenty": [
"string"
],
"forty": [
"string"
],
"oneHundred": [
"string"
],
"unsure": [
"string"
],
"end": "string",
"allowNonBoardMembers": true,
"estimation": 0
},
"targetId_gantt": [
"string"
],
"linkType_gantt": [
0
],
"linkId_gantt": [
"string"
],
"cardNumber": 0
}
Properties
Name | Type | Required | Restrictions | Description |
---|---|---|---|---|
title | string¦null | false | none | the title of the card |
archived | boolean | true | none | is the card archived |
archivedAt | string¦null | false | none | latest archiving date |
parentId | string¦null | false | none | ID of the parent card |
listId | string¦null | false | none | List ID where the card is |
swimlaneId | string | true | none | Swimlane ID where the card is |
boardId | string¦null | false | none | Board ID of the card |
coverId | string¦null | false | none | Cover ID of the card |
color | string¦null | false | none | none |
createdAt | string | true | none | creation date |
modifiedAt | string | true | none | none |
customFields | [CardsCustomfields]¦null | false | none | list of custom fields |
dateLastActivity | string | true | none | Date of last activity |
description | string¦null | false | none | description of the card |
requestedBy | string¦null | false | none | who requested the card (ID of the user) |
assignedBy | string¦null | false | none | who assigned the card (ID of the user) |
labelIds | [string]¦null | false | none | list of labels ID the card has |
members | [string]¦null | false | none | list of members (user IDs) |
assignees | [string]¦null | false | none | who is assignee of the card (user ID), maximum one ID of assignee in array. |
receivedAt | string¦null | false | none | Date the card was received |
startAt | string¦null | false | none | Date the card was started to be worked on |
dueAt | string¦null | false | none | Date the card is due |
endAt | string¦null | false | none | Date the card ended |
spentTime | number¦null | false | none | How much time has been spent on this |
isOvertime | boolean¦null | false | none | is the card over time? |
userId | string | true | none | user ID of the author of the card |
sort | number¦null | false | none | Sort value |
subtaskSort | number¦null | false | none | subtask sort value |
type | string | true | none | type of the card |
linkedId | string¦null | false | none | ID of the linked card |
vote | CardsVote | false | none | none |
poker | CardsPoker | false | none | none |
targetId_gantt | [string]¦null | false | none | ID of card which is the child link in gantt view |
linkType_gantt | [number]¦null | false | none | ID of card which is the parent link in gantt view |
linkId_gantt | [string]¦null | false | none | ID of card which is the parent link in gantt view |
cardNumber | number¦null | false | none | A boardwise sequentially increasing number that is assigned to every newly created card |
Enumerated Values
Property | Value |
---|---|
color | white |
color | green |
color | yellow |
color | orange |
color | red |
color | purple |
color | blue |
color | sky |
color | lime |
color | pink |
color | black |
color | silver |
color | peachpuff |
color | crimson |
color | plum |
color | darkgreen |
color | slateblue |
color | magenta |
color | gold |
color | navy |
color | gray |
color | saddlebrown |
color | paleturquoise |
color | mistyrose |
color | indigo |
CardsVote
{
"question": "string",
"positive": [
"string"
],
"negative": [
"string"
],
"end": "string",
"public": true,
"allowNonBoardMembers": true
}
Properties
Name | Type | Required | Restrictions | Description |
---|---|---|---|---|
question | string | true | none | none |
positive | [string] | false | none | list of members (user IDs) |
negative | [string] | false | none | list of members (user IDs) |
end | string | false | none | none |
public | boolean | true | none | none |
allowNonBoardMembers | boolean | true | none | none |
CardsPoker
{
"question": true,
"one": [
"string"
],
"two": [
"string"
],
"three": [
"string"
],
"five": [
"string"
],
"eight": [
"string"
],
"thirteen": [
"string"
],
"twenty": [
"string"
],
"forty": [
"string"
],
"oneHundred": [
"string"
],
"unsure": [
"string"
],
"end": "string",
"allowNonBoardMembers": true,
"estimation": 0
}
Properties
Name | Type | Required | Restrictions | Description |
---|---|---|---|---|
question | boolean | false | none | none |
one | [string] | false | none | poker card one |
two | [string] | false | none | poker card two |
three | [string] | false | none | poker card three |
five | [string] | false | none | poker card five |
eight | [string] | false | none | poker card eight |
thirteen | [string] | false | none | poker card thirteen |
twenty | [string] | false | none | poker card twenty |
forty | [string] | false | none | poker card forty |
oneHundred | [string] | false | none | poker card oneHundred |
unsure | [string] | false | none | poker card unsure |
end | string | false | none | none |
allowNonBoardMembers | boolean | false | none | none |
estimation | number | false | none | poker estimation value |
CardsCustomfields
{}
Properties
None
ChecklistItems
{
"title": "string",
"sort": 0,
"isFinished": true,
"checklistId": "string",
"cardId": "string",
"createdAt": "string",
"modifiedAt": "string"
}
An item in a checklist
Properties
Name | Type | Required | Restrictions | Description |
---|---|---|---|---|
title | string | true | none | the text of the item |
sort | number | true | none | the sorting field of the item |
isFinished | boolean | true | none | Is the item checked? |
checklistId | string | true | none | the checklist ID the item is attached to |
cardId | string | true | none | the card ID the item is attached to |
createdAt | string¦null | false | none | none |
modifiedAt | string | true | none | none |
Checklists
{
"cardId": "string",
"title": "string",
"finishedAt": "string",
"showAtMinicard": true,
"createdAt": "string",
"modifiedAt": "string",
"sort": 0
}
A Checklist
Properties
Name | Type | Required | Restrictions | Description |
---|---|---|---|---|
cardId | string | true | none | The ID of the card the checklist is in |
title | string | true | none | the title of the checklist |
finishedAt | string¦null | false | none | When was the checklist finished |
showAtMinicard | boolean¦null | false | none | Show at minicard. Default: false. |
createdAt | string | true | none | Creation date of the checklist |
modifiedAt | string | true | none | none |
sort | number | true | none | sorting value of the checklist |
CustomFields
{
"boardIds": [
"string"
],
"name": "string",
"type": "text",
"settings": {
"currencyCode": "string",
"dropdownItems": [
{}
],
"stringtemplateFormat": "string",
"stringtemplateSeparator": "string"
},
"showOnCard": true,
"automaticallyOnCard": true,
"alwaysOnCard": true,
"showLabelOnMiniCard": true,
"showSumAtTopOfList": true,
"createdAt": "string",
"modifiedAt": "string"
}
A custom field on a card in the board
Properties
Name | Type | Required | Restrictions | Description |
---|---|---|---|---|
boardIds | [string] | true | none | the ID of the board |
name | string | true | none | name of the custom field |
type | string | true | none | type of the custom field |
settings | CustomFieldsSettings | true | none | none |
showOnCard | boolean | true | none | should we show on the cards this custom field |
automaticallyOnCard | boolean | true | none | should the custom fields automatically be added on cards? |
alwaysOnCard | boolean | true | none | should the custom field be automatically added to all cards? |
showLabelOnMiniCard | boolean | true | none | should the label of the custom field be shown on minicards? |
showSumAtTopOfList | boolean | true | none | should the sum of the custom fields be shown at top of list? |
createdAt | string¦null | false | none | none |
modifiedAt | string | true | none | none |
Enumerated Values
Property | Value |
---|---|
type | text |
type | number |
type | date |
type | dropdown |
type | checkbox |
type | currency |
type | stringtemplate |
CustomFieldsSettings
{
"currencyCode": "string",
"dropdownItems": [
{}
],
"stringtemplateFormat": "string",
"stringtemplateSeparator": "string"
}
Properties
Name | Type | Required | Restrictions | Description |
---|---|---|---|---|
currencyCode | string | false | none | none |
dropdownItems | [CustomFieldsSettingsDropdownitems] | false | none | list of drop down items objects |
stringtemplateFormat | string | false | none | none |
stringtemplateSeparator | string | false | none | none |
CustomFieldsSettingsDropdownitems
{}
Properties
None
Integrations
{
"enabled": true,
"title": "string",
"type": "string",
"activities": [
"string"
],
"url": "string",
"token": "string",
"boardId": "string",
"createdAt": "string",
"modifiedAt": "string",
"userId": "string"
}
Integration with third-party applications
Properties
Name | Type | Required | Restrictions | Description |
---|---|---|---|---|
enabled | boolean | true | none | is the integration enabled? |
title | string¦null | false | none | name of the integration |
type | string | true | none | type of the integratation (Default to 'outgoing-webhooks') |
activities | [string] | true | none | activities the integration gets triggered (list) |
url | string | true | none | none |
token | string¦null | false | none | token of the integration |
boardId | string | true | none | Board ID of the integration |
createdAt | string | true | none | Creation date of the integration |
modifiedAt | string | true | none | none |
userId | string | true | none | user ID who created the interation |
Lists
{
"title": "string",
"starred": true,
"archived": true,
"archivedAt": "string",
"boardId": "string",
"swimlaneId": "string",
"createdAt": "string",
"sort": 0,
"updatedAt": "string",
"modifiedAt": "string",
"wipLimit": {
"value": 0,
"enabled": true,
"soft": true
},
"color": "white",
"type": "string",
"collapsed": true
}
A list (column) in the Wekan board.
Properties
Name | Type | Required | Restrictions | Description |
---|---|---|---|---|
title | string | true | none | the title of the list |
starred | boolean¦null | false | none | if a list is stared then we put it on the top |
archived | boolean | true | none | is the list archived |
archivedAt | string¦null | false | none | latest archiving date |
boardId | string | true | none | the board associated to this list |
swimlaneId | string | true | none | the swimlane associated to this list. Used for templates |
createdAt | string | true | none | creation date |
sort | number¦null | false | none | is the list sorted |
updatedAt | string¦null | false | none | last update of the list |
modifiedAt | string | true | none | none |
wipLimit | ListsWiplimit | false | none | none |
color | string¦null | false | none | the color of the list |
type | string | true | none | The type of list |
collapsed | boolean | true | none | is the list collapsed |
Enumerated Values
Property | Value |
---|---|
color | white |
color | green |
color | yellow |
color | orange |
color | red |
color | purple |
color | blue |
color | sky |
color | lime |
color | pink |
color | black |
color | silver |
color | peachpuff |
color | crimson |
color | plum |
color | darkgreen |
color | slateblue |
color | magenta |
color | gold |
color | navy |
color | gray |
color | saddlebrown |
color | paleturquoise |
color | mistyrose |
color | indigo |
ListsWiplimit
{
"value": 0,
"enabled": true,
"soft": true
}
Properties
Name | Type | Required | Restrictions | Description |
---|---|---|---|---|
value | number | true | none | value of the WIP |
enabled | boolean | true | none | is the WIP enabled |
soft | boolean | true | none | is the WIP a soft or hard requirement |
Swimlanes
{
"title": "string",
"archived": true,
"archivedAt": "string",
"boardId": "string",
"createdAt": "string",
"sort": 0,
"color": "white",
"updatedAt": "string",
"modifiedAt": "string",
"type": "string",
"collapsed": true
}
A swimlane is an line in the kaban board.
Properties
Name | Type | Required | Restrictions | Description |
---|---|---|---|---|
title | string | true | none | the title of the swimlane |
archived | boolean | true | none | is the swimlane archived? |
archivedAt | string¦null | false | none | latest archiving date of the swimlane |
boardId | string | true | none | the ID of the board the swimlane is attached to |
createdAt | string | true | none | creation date of the swimlane |
sort | number¦null | false | none | the sort value of the swimlane |
color | string¦null | false | none | the color of the swimlane |
updatedAt | string¦null | false | none | when was the swimlane last edited |
modifiedAt | string | true | none | none |
type | string | true | none | The type of swimlane |
collapsed | boolean | true | none | is the swimlane collapsed |
Enumerated Values
Property | Value |
---|---|
color | white |
color | green |
color | yellow |
color | orange |
color | red |
color | purple |
color | blue |
color | sky |
color | lime |
color | pink |
color | black |
color | silver |
color | peachpuff |
color | crimson |
color | plum |
color | darkgreen |
color | slateblue |
color | magenta |
color | gold |
color | navy |
color | gray |
color | saddlebrown |
color | paleturquoise |
color | mistyrose |
color | indigo |